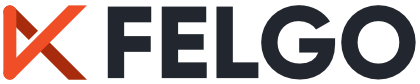
The Felgo Games are available with the following import statement in your QML file:
import Felgo 4.0
This is a list of all the Felgo components grouped into functional groups.
Felgo Plugins add platform-specific features and 3rd party SDK integration available from QML.
Integrate with AdMob to monetize and promote your apps & games with ads. |
|
Integrate with Amplitude to get insights into your app's usage. |
|
Let your users authorize with their Apple ID account on iOS devices. |
|
Integrate with Chartboost to monetize and cross-promote your games with ads. |
|
Integrate with Facebook to help you build engaging social apps and get more installs. |
|
Add Google's Firebase for user authentication and access to the Firebase Realtime Database. |
|
Integrate with Flurry to get insights into your app's usage. |
|
Integrate with GameCenter to send your games' highscores to Apple Game Center on iOS devices. |
|
Integrate with Google Cloud Messaging Push to send cross-platform push notifications and increase your users' engagement. |
|
Integrate with HockeyApp for beta distribution & crash reports. |
|
Schedule native local push notifications in your app. |
|
Let your users authorize with external OAuth 2.0 providers. |
|
Integrate with OneSignal Push to send cross-platform push notifications and increase your users' engagement. |
|
Integrate with Soomla to offer in-app purchases and a virtual economy model within your app. |
|
Integrate with Wikitude to create augmented reality apps. |
Note: For testing the plugins on mobile and source code examples, see the Felgo Plugin Demo. When you develop your own game and want to add plugins to it, a Felgo license is required.
These components every game will contain. Most important are GameWindow, Scene, EntityBase and EntityManager.
Root Felgo element usable with existing Qt applications |
|
Base class for custom game entities |
|
Manages all entities derived from the EntityBase component |
|
Default root Felgo Window containing all other components and Scenes |
|
Root Felgo ApplicationWindow containing all other components and Scenes |
|
Root element for a single game view |
|
Storage item provides a persistent and offline storage for arbitrary key-value pair data |
|
Provides often-needed functionality like generating a random number between 2 values |
The Context Components are global properties that are available in all QML files. They can be used to open native dialogs and browsers, network requests, dynamic QML component creation and provide system information.
Singleton provides file operations like reading, writing and listing files |
|
Singleton allows opening native message boxes, input dialogs and browsers |
|
The Qt Context Objects page contains information about often-used functionality of the Qt element, for network requests and useful JavaScript elements. |
|
Singleton allows to convert recorded spoken audio into text |
|
Singleton allows discovering network services via Bonjour/ZeroConf |
These components are used for efficient rendering in games and to handle multiple screen sizes. Most important is MultiResolutionImage for handling different screen sizes and aspect ratios. And GameSpriteSequence or GameAnimatedSprite for animated sprites.
Enables to follow an object, or be moved around freely |
|
Plays a single sprite animation stored as a series of frames |
|
Element defines a series of sprite frames within an image for a GameSpriteSequence |
|
Contains a list of GameSprite elements and allows switching between them with only one active at a time |
|
Can be used to display colored single and segmented lines |
|
Changes the used image based on the display size to improve performance and memory usage |
|
Allows to create parallax movement effects |
|
Allows to create an endlessly scrollable background, usable by SideScroller games for example |
|
Allows to display polygonal primitives with color |
|
Represents a Box2D RevoluteJoint as defined in the JSON file from the RUBE level editor |
In addition to the above list, also these Qt Quick Components are useful.
Image | The Image element is used to display image files. |
BorderImage | Useful for resolution-independent buttons, similar to Android's 9-patch images. |
Text | The Text element allows displaying text on screen. |
Rectangle | The Rectangle item provides a filled rectangle. |
These components allow entity movement with a keyboard or a virtual controller for touchscreens.
Element provides an input controller for a virtual joystick for moving entities, also called a virtual D-pad |
|
Represents a basic rectangular button with a clicked handler that can be used during development |
|
Allows the user to select from a set of preset values |
|
Has a gradient, radius and border and an optional flatStyle, ready to be published in games & apps |
|
Input controller with keyboard support for moving entities |
In addition to the above list, also these Qt Quick Components are useful:
MouseArea | Allows mouse and touch input handling. |
MultiPointTouchArea | Allows multi-touch input handling. |
Flickable | Provides a surface that can be swiped. |
Keys | Allows key handling, including the Android soft keys like Back . |
TextInput | Displays a single editable line of text. |
TextEdit | Displays multiple lines of editable formatted text. |
The Qt Quick Controls contain more input elements like native-looking Buttons, CheckBoxes and view components like TableView or StackView. See the Qt Quick Controls QML Types for more details.
These components add physics behavior to a game. They can either be used for collision detection, or for moving entities based on physics. The physics engine used by Felgo is Box2D.
Physics body with a rectangular shape |
|
Physics body with a round shape |
|
Simulates a physics world and contains all physics bodies |
|
Physics body with a polygonal shape set up with at least 3 vertices |
To create custom physics objects which use more than the default behavior of BoxCollider, CircleCollider or PolygonCollider, combinations of these Fixture types can be used inside a ColliderBase object:
A rectangular physics shape |
|
An empty chain of points, or empty polygon (loop) |
|
A circular physics shape |
|
A flat edge between two points |
|
Abstract base class of all fixture types |
|
A filled convex polygonal physics shape |
To link multiple physics objects together, the following types of joints can be used:
Keeps the distance between 2 bodies constant |
|
Top-down friction between 2 bodies |
|
Connects 2 Joint objects together |
|
Abstract base class of all joints types |
|
Keeps the relative translation and rotation between 2 bodies constant |
|
Used to manipulate bodies with the mouse or touch input |
|
Allows relative translation of two bodies along a specified axis and prevents rotation |
|
Keeps the total distance between a body, 2 points and another body constant |
|
Forces two bodies to share a common anchor point around which the bodies rotate |
|
Restricts the maximum distance between two points |
|
Glues two bodies together keeping a constant relative angle |
|
Allows relative rotation and translation of two bodies along a specified axis |
The above components will be used most of the time. These components are for advanced use:
A physics body which consists of one or more Fixture elements |
|
Contains static properties of the Box2D physics engine |
|
Base component for BoxCollider, CircleCollider and PolygonCollider. All physics colliders including BoxCollider, CircleCollider and PolygonCollider inherit from ColliderBase |
|
Information about a physics contact event |
|
Draws a debug view of the physics components |
|
A property group for getting profiling data about the current state and performance of the physics simulation |
|
A RayCast object that reports when a ray hits a Fixture |
|
Represents a Box2D physics world including bodies and joints |
These components allow to play audio and video files.
Element allows playing long-lasting and looping background sound in wav, mp3 or ogg file format |
|
Element allows playing short-lasting and looping sound effects in wav file format |
|
Embedded video player based on the YouTube Iframe-Player API |
In addition to the above list, also these Qt Quick Components are useful:
SoundEffect | Provides a way to play sound effects in QML |
MediaPlayer | Add media playback to a scene |
Video | A convenience type for showing a specified video |
VideoOutput | Render video or camera viewfinder |
Camera | Access viewfinder frames, and take photos and movies |
QtMultimedia | Provides a global object with useful functions from Qt Multimedia |
These components handle network activities.
Provides functionality for scaling and cropping an image file before uploading it with HttpRequest |
|
Convenience type to check pending requests when using HttpRequest |
|
Allows to implement networking features based on the SuperAgent library from VisionMedia |
|
Offers an API similar to the Promises API in ES2017 |
|
The XMLHttpRequest object can be used to asynchronously obtain data from over a network. |
JsonListModel allows to transform your JSON data into a QML ListModel for usage with e.g. an AppListView.
A proxy view model for JSON data sources |
SortFilterProxyModel is an implementation of QSortFilterProxyModel conveniently exposed for QML. You can use it to apply filter and sorting settings to your QML ListModel items.
Filter container accepting rows accepted by all its child filters |
|
Filter container accepting rows accepted by at least one of its child filters |
|
Filters row with a custom filtering |
|
A custom role computed from a javascript expression |
|
Sorts row with a custom javascript expression |
|
Base type for the SortFilterProxyModel filters |
|
Abstract interface for types containing Filters |
|
A role resolving to true for rows matching all its filters |
|
Sorts rows based on if they match filters |
|
Filters rows based on their source index |
|
Role made from concatenating other roles |
|
Sorts rows based on a locale aware comparison of a source model string role |
|
Base type for the SortFilterProxyModel proxy roles |
|
Filters rows between boundary values |
|
Filters rows matching a regular expression |
|
A ProxyRole extracting data from a source role via a regular expression |
|
Base type for filters based on a source model role |
|
Sorts rows based on a source model role |
|
Base type for the SortFilterProxyModel proxy roles defining a single role |
|
Allows to apply filter and sorting settings to QML ListModel items |
|
Base type for the SortFilterProxyModel sorters |
|
Abstract interface for types containing Sorters |
|
Sorts rows by comparing a source model string role with a localized collation algorithm |
|
A role using Filter to conditionnaly compute its data |
|
Filters rows matching exactly a value |
The AI components move entities towards a target point, with a constant velocity or along a path.
Provides information for a MovementAnimation or a ColliderBase about the direction and rotation when moving towards a target |
|
Modifies a property with a constant velocity and acceleration |
|
Interpolates an entity along a path and rotates it towards the target points |
The RUBE components allow loading of levels and Box2D objects created with RUBE, which stands for Really Useful Box2D Editor. You can use RUBE to create side scrollers like Badland or Jetpack Joyride, platformers like Super Mario, and other physics-based games.
Represents a Box2D body as defined in the JSON file from the RUBE level editor |
|
Represents a Box2D Circle fixture as defined in the JSON file from the RUBE level editor |
|
Represents a Box2D Polygon fixture as defined in the JSON file from the RUBE level editor |
|
Represents an image as defined in the JSON file from the RUBE level editor |
|
Creates QML items based on the description of a JSON file exported by the RUBE level editor |
The slot machine components allow to create slot games with Felgo.
Component allows to easily implement a slot machine with multiple reels and rows. It also provides methods to spin or stop the slot machine and lets you access the visible items in each reel and row |
|
Allows to easily generate reels with randomly shuffled items for a SlotMachine |
|
Element describes a reel to be displayed within the SlotMachine component |
The Particles components provide particle effect rendering.
Component allows visual effects like fire, explosions, smoke or rain |
The QML Native Code Components allow to interact with the native platform.
You can show native views as part of your QML scene. You can also call native APIs directly from QML.
Represents a platform-native class |
|
Represents a platform-native object |
|
Allows you to interact with objects native to the mobile platform |
|
Allows to instantiate and display platform-specific views and widgets |
|
Defines the platform specifics for NativeView |
The Native App Integration components allow to integrate Felgo in native applications.
Initializes the Felgo runtime from a native Android application |
|
Integrates Felgo in a native Android application |
|
Shows Felgo QML content in a native Android application |
|
Shows Felgo QML content in a native Android application |
|
Initializes the Felgo runtime from a native iOS application |
|
Shows Felgo QML content inside a native iOS application |
Item | The Item is the most basic of all visual items in QML. |
Animation | The Animation element is the base of all QML animations lasting for a fixed time. |
Timer | The Timer item triggers a handler at a specified interval. |
Loader | The Loader item allows dynamically loading an Item-based subtree from a URL or Component. |
See here for a full list of all QML components.