Welcome to the Web Demo
Only 2 Steps to Your First App
1. Download the Felgo Live Scripting App
The Felgo Live Scripting app is available for Android and iOS in the Appstore. Search for Felgo Live Scripting.
2. Connect the App
Open the app and switch to the Web Script tab. To connect the app with this editor, you only need to generate a Live-ID and enter it in the App.
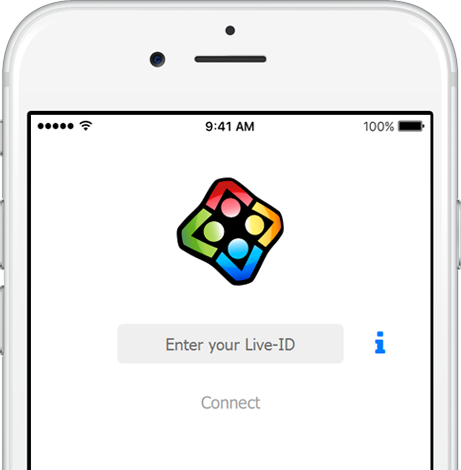