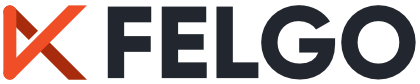
a generic property type. More...
The var
type is a generic property type that can refer to any data type.
It is equivalent to a regular JavaScript variable. For example, var properties can store numbers, strings, objects, arrays and functions:
Item { property var aNumber: 100 property var aBool: false property var aString: "Hello world!" property var anotherString: String("#FF008800") property var aColor: Qt.rgba(0.2, 0.3, 0.4, 0.5) property var aRect: Qt.rect(10, 10, 10, 10) property var aPoint: Qt.point(10, 10) property var aSize: Qt.size(10, 10) property var aVector3d: Qt.vector3d(100, 100, 100) property var anArray: [1, 2, 3, "four", "five", (function() { return "six"; })] property var anObject: { "foo": 10, "bar": 20 } property var aFunction: (function() { return "one"; }) }
It is important to note that changes in regular properties of JavaScript objects assigned to a var property will not trigger updates of bindings that access them. The example below will display "The car has 4 wheels" as the change to the wheels property will not cause the reevaluation of the binding assigned to the "text" property:
Item { property var car: new Object({wheels: 4}) Text { text: "The car has " + car.wheels + " wheels"; } Component.onCompleted: { car.wheels = 6; } }
If the onCompleted handler instead had "car = new Object({wheels: 6})"
then the text would be updated to say "The car has 6 wheels", since the car property itself would be changed, which causes a change
notification to be emitted.
The QML syntax defines that curly braces on the right-hand-side of a property value initialization assignment denote a binding assignment. This can be confusing when initializing a var
property, as empty curly
braces in JavaScript can denote either an expression block or an empty object declaration. If you wish to initialize a var
property to an empty object value, you should wrap the curly braces in parentheses.
For example:
Item { property var first: {} // nothing = undefined property var second: {{}} // empty expression block = undefined property var third: ({}) // empty object }
In the previous example, the first
property is bound to an empty expression, whose result is undefined. The second
property is bound to an expression which contains a single, empty expression block
("{}"), which similarly has an undefined result. The third
property is bound to an expression which is evaluated as an empty object declaration, and thus the property will be initialized with that empty object
value.
Similarly, a colon in JavaScript can be either an object property value assignment, or a code label. Thus, initializing a var property with an object declaration can also require parentheses:
Item { property var first: { example: 'true' } // example is interpreted as a label property var second: ({ example: 'true' }) // example is interpreted as a property property var third: { 'example': 'true' } // example is interpreted as a property Component.onCompleted: { console.log(first.example) // prints 'undefined', as "first" was assigned a string console.log(second.example) // prints 'true' console.log(third.example) // prints 'true' } }
See also QML Value Types.
As part of the free Business evaluation, we offer a free welcome call for companies, to talk about your requirements, and how the Felgo SDK & Services can help you. Just sign up and schedule your call.
Sign up now to start your free Business evaluation: