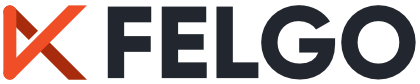
You can use the NavigationStack to push pages on a stack. The user can then navigate back through the stack page by page. On iOS the user will be able to use the swipe back gesture, and the back button can be used on Android.
import Felgo import QtQuick App { NavigationStack { AppPage { id: page title: "Main Page" AppButton { anchors.centerIn: parent text: "Push Sub Page" onClicked: { // You need to specify the NavigationStack where to push the new page // You can either give the NavigationStack and id, or like in this example, use the navigationStack property of the current page page.navigationStack.push(subPage) } } } } Component { id: subPage AppPage { title: "Sub Page" } } }
In addition to the common NavigationStack concept, you can also use a drawer or tabs for another form of navigation.
import Felgo import QtQuick App { Navigation { // You can try different navigation modes by uncommenting the lines below // By default, the mode is chosen depending on the platform. Tabs on iOS, drawer on Android //navigationMode: navigationModeDrawer //navigationMode: navigationModeTabs NavigationItem { title: "Main" iconType: IconType.heart NavigationStack { AppPage { id: page title: "Main Page" AppButton { anchors.centerIn: parent text: "Push Sub Page" onClicked: { page.navigationStack.push(subPage) } } } } } NavigationItem { title: "Second" iconType: IconType.thlarge NavigationStack { AppPage { title: "Second Page" } } } } Component { id: subPage AppPage { title: "Sub Page" } } }
Each NavigationStack automatically adds a NavigationBar that shows the current page title and other NavigationBarItems. By setting the AppPage::navigationBarHidden property to true
, the navigation bar won't be shown for
that page.
The following example shows a Page with a button that allows to show or hide the navigation bar:
iOS | Android |
---|---|
|
|
import Felgo App { NavigationStack { AppPage { id: page title: "Hide Navigation Bar" // this is the default, the NavigationStack shows a navigation bar for this page navigationBarHidden: false AppButton { anchors.centerIn: parent text: "Show/Hide Navigation Bar" // when clicked, we switch the navigationBarHidden property onClicked: page.navigationBarHidden = !page.navigationBarHidden } } } }
The NavigationStack shows the AppPage::titleItem of the currently active page in the navigation bar. This default title item is a simple Text that shows the AppPage::title. By overwriting the AppPage::titleItem you can replace the item.
The following example replaces the default title item to show an image together with the page title:
iOS | Android |
---|---|
|
|
import Felgo import QtQuick App { NavigationStack { AppPage { id: page title: "My Title" // we define a custom titleItem, that consists of an image and a title text titleItem: Row { spacing: dp(6) Image { anchors.verticalCenter: parent.verticalCenter height: titleText.height fillMode: Image.PreserveAspectFit source: "https://felgo.com/web-assets/felgo-logo-small.png" } AppText { id: titleText anchors.verticalCenter: parent.verticalCenter text: page.title font.bold: true font.family: Theme.boldFont.name font.pixelSize: dp(Theme.navigationBar.titleTextSize) color: "orange" } } // titleItem } } }
You can use the NativeUtils::openApp() or NativeUtils::openUrl() methods. It expects an iOS URL scheme or Android bundle id for the target app, which the device then opens.
Find more examples for frequently asked development questions and important concepts in the following guides: