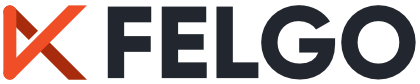
With the QML Multimedia components, you can access and capture from the device camera.
This example uses the Camera component to simply view the device camera output.
import Felgo import QtQuick import QtMultimedia App { // Displays a title bar and is used for navigation between pages NavigationStack { AppPage { title: "Camera" Camera { id: camera active: true } CaptureSession { camera: camera videoOutput: output } VideoOutput { id: output anchors.fill: parent } } } }
You can also use the Multimedia components to capture images or videos from the camera. You can look at the CaptureSession component to find an example.
As a convenient alternative, Felgo also provides methods for this with the NativeUtils. This example opens the native camera dialog to take a picture.
import Felgo import QtQuick App { id: app NavigationStack { AppPage { AppImage { id: image anchors.fill: parent // important to automatically rotate the image taken from the camera autoTransform: true fillMode: Image.PreserveAspectFit } AppButton { anchors.centerIn: parent text: "Display CameraPicker" onClicked: { NativeUtils.displayCameraPicker() } } Connections { target: NativeUtils onCameraPickerFinished: (accepted, path) => { if(accepted) image.source = path } } } } }
For QR Code scanning, we recommend this library: https://github.com/ftylitak/qzxing
We also used it in this open-source example app: Qt World Summit Conference App
Find more examples for frequently asked development questions and important concepts in the following guides:
As part of the free Business evaluation, we offer a free welcome call for companies, to talk about your requirements, and how the Felgo SDK & Services can help you. Just sign up and schedule your call.
Sign up now to start your free Business evaluation: