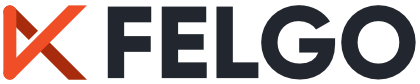
NativeViewBinding defines the platform specifics for NativeView. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 3.3.0 |
This type defines how to create, display and interact with a NativeView on one specific platform. You usually only use it for the NativeView::androidBinding and NativeView::iosBinding properties.
It defines which view class to instantiate via the viewClassName property. When NativeView instantiated the view, it emits viewCreated(). Use it to initialize the view before showing it. Afterwards, you can access the native view instance with view.
Example usage to display and interact with a native button:
import Felgo import QtQuick App { NativeView { id: dynamicNativeButton width: dp(170) height: dp(48) anchors.centerIn: parent property string text: qsTr("Native Button") signal clicked onTextChanged: if(binding) binding.updateText() androidBinding: NativeViewBinding { viewClassName: "android.widget.Button" onViewCreated: { updateText() // add OnClickListener: view.callMethod("setOnClickListener", function(v) { // call QML signal: dynamicNativeButton.clicked() }) } function updateText() { view.callMethod("setText", dynamicNativeButton.text, NativeObject.UiThread) } } iosBinding: NativeViewBinding { viewClassName: "UIButton" readonly property NativeObject _UIColor: NativeObjectUtils.getClass("UIColor") onViewCreated: { updateText() // configure button visuals: view.setProperty("backgroundColor", _UIColor.getProperty("grayColor")) view.callMethod("setTitleColor:forState:", [_UIColor.getProperty("blackColor"), 0]) view.callMethod("setTitleColor:forState:", [_UIColor.getProperty("whiteColor"), 1]) // add touchUpInside target: var _UIControlEventTouchUpInside = 1 << 6 view.callMethod("addTarget:action:forControlEvents:", [{ // note: selector name contains colon ':', so this key must also contain it "onClick:forEvent:": function(view, event) { dynamicNativeButton.clicked() } }, "onClick:forEvent:", _UIControlEventTouchUpInside]) // target selectors can either be "doSomething", "doSomething:(id)sender" or "doSomething:(id)sender forEvent:(UIEvent*)event" // selector name must be exactly the same as the function's key in the JS object } function updateText() { view.callMethod("setTitle:forState:", [dynamicNativeButton.text, 0]) } } } }
onCreateView : script |
This property lets you customize the native view initialization.
You can set it to a JavaScript code block. The item then executes this code block when creating the native view. Return an instance of a native view from the code block.
On Android, return any NativeObject derived from android.view.View
. On iOS, return any NativeObject derived from
UIView
.
The following example calls a custom constructor of WKWebView
on iOS. The web view requires this for changing its configuration. On Android, it uses auto-initialization via viewClassName.
import Felgo import QtQuick App { NativeView { anchors.fill: parent androidBinding: NativeViewBinding { viewClassName: "android.webkit.WebView" onViewCreated: { // post-initialization code here... updateUrl() } function updateUrl() { view.callMethod("loadUrl", "https://felgo.com", NativeObject.UiThread) } } iosBinding: NativeViewBinding { // imports readonly property NativeClass _WKWebView: NativeObjectUtils.getClass("WKWebView") readonly property NativeClass _WKWebViewConfiguration: NativeObjectUtils.getClass("WKWebViewConfiguration") readonly property NativeClass _NSURL: NativeObjectUtils.getClass("NSURL") readonly property NativeClass _NSURLRequest: NativeObjectUtils.getClass("NSURLRequest") // WKWebViewConfiguration must be passed on constructor // -> use custom initialization block: onCreateView: { var config = _WKWebViewConfiguration.newInstance() // configure your web view here: config.setProperty("allowsInlineMediaPlayback", true) // constructor method (selector) name is "initWithFrame:configuration:" return _WKWebView.newInstance([null, config], "initWithFrame:configuration:") } onViewCreated: { // post-initialization code here... updateUrl() } function updateUrl() { // must convert to NSURL from string, TODO maybe automatically convert QML url <-> NSURL var url = _NSURL.callMethod("URLWithString:", "https://felgo.com") var request = _NSURLRequest.callMethod("requestWithURL:", url) view.callMethod("loadRequest:", request) } } } }
If your view does not require custom initialization, you can instead just specify viewClassName.
See also viewClassName and viewCreated().
[read-only] view : NativeObject |
This read-only property contains an instance of a native View object. NativeView sets it when it instantiates the View, before showing it.
viewClassName : string |
This property defines which native View class to instantiate.
On Android, use any class derived from android.view.View
. On iOS, use any class derived from UIView
.
When you specify this property, the item calls a default constructor on the class. On iOS, this means it calls a method init
.
You can also customize view initialization. For this, specify a code block for onCreateView and omit the viewClassName
.
See also onCreateView and viewCreated().
viewCreated() |
This signal is emitted after NativeView instantiated the View object. You can use it to initialize the View before showing it.
Note: The corresponding handler is onViewCreated
.
See also viewClassName and onCreateView.