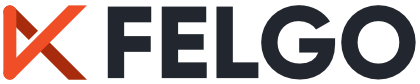
This first example fills the whole page with an AppImage. Since the image does not match the page aspect ratio, it will be distorted.
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Hello Felgo" AppImage { // The image fills the page completely, this can cause distortion depending on the image size anchors.fill: parent source: "https://felgo.com/web-assets/girl.jpg" } } } }
In the next example, we chose another fillMode to avoid distortion.
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Hello Felgo" AppImage { anchors.fill: parent // You can try different fillModes fillMode: Image.PreserveAspectFit //fillMode: Image.PreserveAspectCrop //fillMode: Image.Tile source: "https://felgo.com/web-assets/girl.jpg" } } } }
Rounded images are very common in apps, this is why Felgo offers the RoundedImage type, that lets you add a radius to an image.
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Hello Felgo" RoundedImage { anchors.centerIn: parent width: dp(200) height: dp(200) fillMode: Image.PreserveAspectCrop source: "https://felgo.com/web-assets/girl.jpg" // Using half the width as radius causes a full circle radius: width/2 } } } }
You can also use a basic fullscreen image preview component, the PictureViewer.
import Felgo import QtQuick App { id: app NavigationStack { AppPage { title: "Hello Felgo" AppButton { text: "Show Photo" onClicked: { // Open PictureViewer component PictureViewer.show(app, "https://felgo.com/web-assets/girl.jpg") } } } } }
ImagePicker is a custom QML GridView, which accesses photos from the device gallery or camera roll. It supports selecting multiple images.
Note: For selecting a single image, you can also use the NativeUtils::displayImagePicker() function. It uses the image picker dialog of the native platform instead of the custom QML view of ImagePicker.
The following example shows how you can use the ImagePicker in one of your app pages.
import Felgo import QtQuick App { NavigationStack { AppPage { id: page title: qsTr("Choose Photos") // right bar item to accept selection rightBarItem: TextButtonBarItem { text: "Next" enabled: imagePicker.selectedCount > 0 // your app will probably pop the photo selection page from the stack to move on // this example only logs the selected photos onClicked: console.debug("SELECTED:", JSON.stringify(imagePicker.selection)) } // image picker view for photo selection ImagePicker { id: imagePicker anchors.fill: parent } } } }
You can use the NativeUtils::displayImagePicker function to open the native image gallery select dialog.
import Felgo import QtQuick App { id: app NavigationStack { AppPage { AppImage { id: image anchors.fill: parent // important to automatically rotate the image taken from the camera autoTransform: true fillMode: Image.PreserveAspectFit } AppButton { anchors.centerIn: parent text: "Display CameraPicker" onClicked: { NativeUtils.displayImagePicker("test") } } Connections { target: NativeUtils onImagePickerFinished: (accepted, path) => { if(accepted) image.source = path } } } } }
The Canvas item allows to draw straight and curved lines, simple and complex shapes, graphs, and referenced graphic images. It can also add text, colors, shadows, gradients, and patterns, and do low-level pixel operations.
The width and height properties of the Canvas item define the drawing area. If it requires rendering, the paint signal fires. When handling the signal, you can draw to the Canvas using a Context2D object:
import Felgo import QtQuick App { AppPage { Canvas { id: mycanvas width: 100 height: 200 onPaint: { var ctx = getContext("2d"); ctx.fillStyle = Qt.rgba(1, 0, 0, 1); ctx.fillRect(0, 0, width, height); } } } }
To use SVG images in your project, add the Qt SVG module to the CMakeLists.txt
project configuration file. You can then use the AppImage component to display SVG images.
Note: If the SVG module is not linked, you see errors like QML AppImage: Error decoding URL
in your app's log output.
find_package(Felgo REQUIRED) find_package(Qt6 REQUIRED COMPONENTS Svg) ... target_link_libraries(appExample PRIVATE Felgo) target_link_libraries(appExample PRIVATE Qt6::Svg)
Find more examples for frequently asked development questions and important concepts in the following guides: