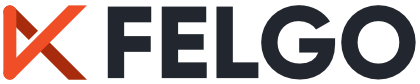
There are various forms of user input. The most important options are covered in this document.
This is covered in the following doc: Handle Touch and Gestures in Your App
Depending on the type of text, and how it fits your UI/UX, Felgo offers different components to get text input from the user.
For selecting a date, you can display the native date picker dialog with NativeUtils::displayDatePicker.
You can also use the DatePicker control to let the user choose a date, time or time interval:
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Date Picker" AppText { text: datePicker.selectedDate.toUTCString() anchors.horizontalCenter: parent.horizontalCenter anchors.bottom: datePicker.top } DatePicker { id: datePicker anchors.centerIn: parent } } } }
See more Usage Examples in the DatePicker documentation.
This is covered in the following doc: Use Native Device Sensors
The NativeUtils offer a lot more native features, like picking images from the gallery, or taking photos with the camera.
Input controls like AppTextInput or AppTextField own a placeHolderText property, which you can use for your placeholder text.
import Felgo import QtQuick App { AppPage { // remove focus from textedit if background is clicked MouseArea { anchors.fill: parent onClicked: textEdit.focus = false } // background for input Rectangle { anchors.fill: textEdit anchors.margins: -dp(8) color: "lightgrey" } // input AppTextInput { id: textEdit width: dp(200) placeholderText: "What's your name?" anchors.centerIn: parent } } }
The text input components provide predefined validators that you can use, they are called inputMethodHints.
You can also add custom validators to restrict the accepted input to a certain input type or expression. Input that does not match the validator is not accepted. To do custom validations and show errors for accepted input, you can add simple checks and control the visibility of errors with property bindings:
import Felgo import QtQuick App { // background for input Rectangle { anchors.fill: textInput anchors.margins: -dp(8) color: "lightgrey" } // input AppTextInput { id: textInput width: dp(200) placeholderText: "What's your name?" anchors.centerIn: parent // only allow letters and check length validator: RegularExpressionValidator { regularExpression: /[A-Za-z]+/ } property bool isTooLong: textInput.text.length >= 6 } // show error if too long AppText { text: "Error: Use less than 6 letters." color: "red" anchors.top: textInput.bottom anchors.topMargin: dp(16) anchors.left: textInput.left visible: textInput.isTooLong } }
Find more examples for frequently asked development questions and important concepts in the following guides:
As part of the free Business evaluation, we offer a free welcome call for companies, to talk about your requirements, and how the Felgo SDK & Services can help you. Just sign up and schedule your call.
Sign up now to start your free Business evaluation: