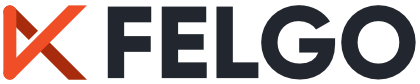
An important concept for apps is density independence. Which means, no matter how big the device is physically, and no matter what the display resolution is, any item should have the same physical size on every device. A button on a small phone should have the same size on a bigger phone or tablet. Same for the height of list items for example.
Felgo offers tool to get density independent values to support any screen size and resolution.
There is also an in-depth guide on this topic if you want to find out more about this: Supporting Multiple Screen Sizes & Screen Densities with Felgo Apps
Those are the most important functions and properties of the App components, that will help you with density independence:
font.pixelSize
. This allows to
dynamically change the size of all the text in your app with the App::spScale property.
In addition to these functions, you may also choose to adapt your layout based on the actual screen size or depending on the interface orientation:
true
if the display's diameter is bigger than 5 inches.
true
, depending on the current device orientation.
This is the most basic form of positioning. Note that you should always use density-independent values with dp(), for positioing and sizes of your components.
import Felgo import QtQuick App { NavigationStack { AppPage { title: "X & Y Positioning" AppText { x: dp(30) y: dp(50) text: "I am positioned with x and y!" } } } }
With anchoring, you can align an item relative to its parent item or any sibling item.
Here is a more detailed guide about anchoring: Positioning with Anchors
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Anchoring" AppText { id: centerItem anchors.centerIn: parent text: "Centered" } AppText { anchors.top: parent.top anchors.left: parent.left text: "TopLeft" } AppText { anchors.top: parent.top anchors.right: parent.right text: "TopRight" } AppText { anchors.bottom: parent.bottom anchors.left: parent.left text: "BottomLeft" } AppText { anchors.bottom: parent.bottom anchors.right: parent.right text: "BottomRight" } // This item uses some more available anchoring options // centerItem is a sibling of this text, thus we can anchor to it AppText { anchors.verticalCenter: centerItem.verticalCenter // Anchoring by vertical center anchors.verticalCenterOffset: dp(10) // Positive or negative offset from the vertical center anchors.right: centerItem.left // We anchor the right edge to the left edge of centerItem anchors.rightMargin: dp(20) // This creates an offset from the right edge text: "Relative" } } } }
You can also use anchoring to define the size of items:
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Boxes" // Fills the whole page with red Rectangle { anchors.fill: parent color: "red" } // Fills the page with green but with margins Rectangle { anchors.fill: parent anchors.topMargin: dp(50) anchors.bottomMargin: dp(50) anchors.leftMargin: dp(50) anchors.rightMargin: dp(50) color: "green" } // A fixed width blue rectangle centered in the page Rectangle { anchors.centerIn: parent width: dp(100) height: dp(100) color: "blue" } } } }
You can use positioner components to create rows, columns, grids and more complex layouts.
Here is a more detailed guide about positioners: Item Positioners
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Row" // Aligns all child items in a row Row { anchors.centerIn: parent // This is the space between each item in the row spacing: dp(10) Rectangle { width: dp(100) height: dp(100) color: "red" } Rectangle { width: dp(100) height: dp(100) color: "green" } Rectangle { width: dp(100) height: dp(100) color: "blue" } } } } }
This is closely documented here: Qt Quick Layouts
You can control the visibility of each item as you like. Items thus do not require to be re-created and can keep their state while being hidden.
This example toggles between two items with an AppButton:
import Felgo App { AppPage { AppText { id: appTextItem text: "Some Text" anchors.centerIn: parent } AppButton { text: "A Button" visible: !appTextItem.visible // hide button if text is visible and vice versa onClicked: console.log("Button clicked!") anchors.centerIn: parent } AppButton { anchors.bottom: parent.bottom flat: false text: "Toggle" onClicked: appTextItem.visible = !appTextItem.visible // show/hide text } } }
Find more examples for frequently asked development questions and important concepts in the following guides: