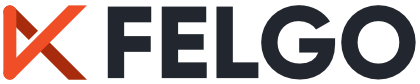
Qt already provides several different QML types to create graphical effects like blur, drop shadow, opacity masks and more. See here for an overview of all effects: Graphical Effects
The following example creates a list page that uses a custom navigation bar background with an image. When the list is scrolled, the background image is blurred using the FastBlur effect:
iOS | Android |
---|---|
|
|
import Felgo import QtQuick import Qt5Compat.GraphicalEffects App { NavigationStack { ListPage { id: page title: "Blur Effect" // get the total height of status bar and navigation bar readonly property real barHeight: dp(Theme.navigationBar.height) + Theme.statusBarHeight // navigation bar is 100 percent translucent, the page then also fills the whole screen // this allows us to display a custom navigation bar background for this page navigationBarTranslucency: 1.0 // list view only fills page area below navigation bar listView.anchors.topMargin: barHeight // add twenty dummy items to the list model: 20 delegate: SimpleRow { text: "Item #"+index } // custom navigation bar background that shows an image Rectangle { id: background width: parent.width height: page.barHeight color: Theme.navigationBar.backgroundColor // add the image Image { id: bgImage source: "https://felgo.com/web-assets/felgo-logo.png" anchors.fill: parent fillMode: Image.PreserveAspectCrop // the blur effect displays the image, we set the source image invisible visible: false } // apply blur effect FastBlur { id: blur source: bgImage anchors.fill: bgImage // the strength of the blur is based on the list view scroll position radius: Math.max(0, Math.min(64, page.listView.contentY/3)) } } // Rectangle }// ListPage } }
You can also define custom shaders using the ShaderEffect component.
To add particles, you can use the particle components: Qt Quick Particles QML Types
This guide is very helpful for using particles: https://qmlbook.github.io/ch08/index.html
As part of the free Business evaluation, we offer a free welcome call for companies, to talk about your requirements, and how the Felgo SDK & Services can help you. Just sign up and schedule your call.
Sign up now to start your free Business evaluation: