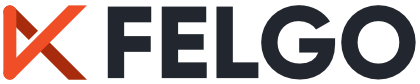
Felgo allows you to style your app with app-wide theme settings using the Theme component. This is in most cases more convenient than styling every single component itself in your code.
import Felgo import QtQuick App { onInitTheme: { // Set the navigation bar background to blue Theme.navigationBar.backgroundColor = "blue" // Set the global text color to red Theme.colors.textColor = "red" } NavigationStack { AppPage { title: "Hello Felgo" AppText { anchors.centerIn: parent text: "Hi :)" } } } }
This example applies dark theme settings. The navigation bar background is set to black. In order to see the device status bar, it is set to white (light) style.
import Felgo import QtQuick App { onInitTheme: { // Set the status bar style to white (light style) Theme.colors.statusBarStyle = Theme.colors.statusBarStyleWhite // Set the navigation bar background to black Theme.navigationBar.backgroundColor = "black" Theme.navigationBar.titleColor = "white" Theme.navigationBar.dividerColor = "black" // Set the background color, which is used as background color of pages Theme.colors.backgroundColor = "#222" // Set the global text color to white Theme.colors.textColor = "white" } // Displays a title bar and is used for navigation between pages NavigationStack { AppPage { title: "Hello Felgo" // Is displayed in the title bar AppText { anchors.centerIn: parent text: "Hi :)" } } } }
The Felgo blog contains a full step-by-step tutorial and example on how to query the native Dark Mode setting of a mobile device. The example also shows how to introduce a dark theme in your application and allow the user to switch between themes.
Example: Dark Mode with Qt on iOS and Android using Felgo Native Code Components
The default Theme settings implement platform-specific styles, like the default iOS style on iOS or Material Style on Android, for a native look and feel on both platforms. The following example shows how to manually change the used platform theme in your code:
import Felgo App { Navigation { NavigationItem { title: "Main" iconType: IconType.heart NavigationStack { AppPage { title: "Main Page" AppButton { text: "Switch Theme" onClicked: Theme.platform = Theme.isAndroid ? "ios" : "android" anchors.centerIn: parent } } } } NavigationItem { title: "Second" iconType: IconType.thlarge NavigationStack { AppPage { title: "Second Page" } } } } }
Felgo components make use of two different fonts, the Theme::normalFont and the Theme::boldFont. By default, these fonts match the platform's default font for Felgo Apps components.
If you want to explicitly provide your own font you can override the Theme properties with a FontLoader object:
import Felgo import QtQuick App { // initialize theme with new font onInitTheme: { Theme.normalFont = arialFont } // load new font with FontLoader FontLoader { id: arialFont source: "../assets/fonts/Arial.ttf" // loaded from your assets folder } AppPage { // Felgo components like AppText automatically use the Theme fonts AppText { text: "I'm in Arial" } } }
Make sure to add your custom fonts to your app's assets and to provide the correct path in the FontLoader object. You can also use the custom font in your own app components, like the following example:
Text { // Set reference to the global app font font.family: Theme.normalFont.name text: "Custom text item" }
Instead of replacing the general Theme font, you can also use a FontLoader and only overwrite the font.family
property for certain text
items.
Find more examples for frequently asked development questions and important concepts in the following guides:
As part of the free Business evaluation, we offer a free welcome call for companies, to talk about your requirements, and how the Felgo SDK & Services can help you. Just sign up and schedule your call.
Sign up now to start your free Business evaluation: