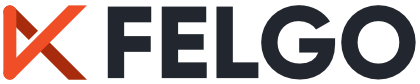
The AppMap component is an extension of the QML Map item and can display a map and optionally the user location. To actually display the map, it is required to specify a Plugin that provides the map data. QML currently supports the following plugins:
The following example creates a Map that uses the Maplibre GL Map plugin:
iOS | Android |
---|---|
|
|
import Felgo import QtLocation App { NavigationStack { AppPage { title: "Map Example" // show the map AppMap { anchors.fill: parent plugin: Plugin { name: "maplibregl" // configure your styles and other parameters here parameters: [ PluginParameter { name: "maplibregl.mapping.additional_style_urls" value: "https://api.maptiler.com/maps/streets/style.json?key=get_your_own_OpIi9ZULNHzrESv6T2vL" } ] } } } } }
The AppMap component allows to show a map and optionally the user location. It is based on the QML Map item.
The AppMap already comes with a built-in feature to detect and display the user location. Set the AppMap::showUserPosition property to
true
to enable this feature. The app then tries to detect the user location and displays a marker on the map if possible. The position can only be displayed if the device is capable of getting a position from
either GPS or other position sources.
Note: Also make sure to add required configuration settings to AndroidManifest.xml
on Android or the Project-Info.plist
on iOS so your app may access gps location on the device.
The following example detects and displays the user location:
iOS | Android |
---|---|
|
|
import Felgo import QtLocation import QtQuick App { NavigationStack { AppPage { title: "User Position" // show the map AppMap { anchors.fill: parent plugin: Plugin { name: "maplibregl" // configure your styles and other parameters here parameters: [ PluginParameter { name: "maplibregl.mapping.additional_style_urls" value: "https://api.maptiler.com/maps/streets/style.json?key=get_your_own_OpIi9ZULNHzrESv6T2vL" } ] } // configure the map to try to display the user's position showUserPosition: true zoomLevel: 13 // check for user position initially when component is created Component.onCompleted: { if(userPositionAvailable) center = userPosition.coordinate } // once we successfully received the location, we zoom to the user position onUserPositionAvailableChanged: { if(userPositionAvailable) zoomToUserPosition() } } } } }
There are several overlay items that can be placed on a map, for a list of all possible overlay items see here. The MapQuickItem type allows to place custom QML items on the map, which is what we will use for this example. The following QML code creates a map with a custom overlay at its center:
iOS | Android |
---|---|
|
|
import Felgo import QtLocation import QtPositioning import QtQuick App { NavigationStack { AppPage { title: "Map Overlay" // show the map AppMap { anchors.fill: parent center: QtPositioning.coordinate(48.2082,16.3738) plugin: Plugin { name: "maplibregl" // configure your styles and other parameters here parameters: [ PluginParameter { name: "maplibregl.mapping.additional_style_urls" value: "https://api.maptiler.com/maps/streets/style.json?key=get_your_own_OpIi9ZULNHzrESv6T2vL" } ] } MapQuickItem { // overlay will be placed at the map center coordinate: QtPositioning.coordinate(48.2082,16.3738) // the anchor point specifies the point of the sourceItem that will be placed at the given coordinate anchorPoint: Qt.point(sourceItem.width/2, sourceItem.height/2) // source item holds the actual item that is displayed on the map sourceItem: Rectangle { width: dp(180) height: dp(50) color: "white" radius: dp(10) AppText { text: "The heart of Vienna!" anchors.centerIn: parent } } } // MapQuickItem } // AppMap } } }
Felgo allows you to access various device sensors, with the Qt Sensors QML Types.
The components for Positioning, Maps, and Navigation are found in the Qt Positioning and Qt Location modules.
For example, you can also use the QML PositionSource type to retrieve the GPS position. The AppMap::showUserPosition feature internally uses PositionSource as well.
Find more examples for frequently asked development questions and important concepts in the following guides: