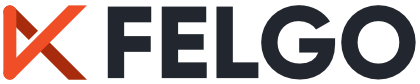
The AlphaVideo component allows you to show videos with transparency. More...
Import Statement: | import Felgo 3.0 |
Inherits: |
The AlphaVideo component internally uses the MediaPlayer, VideoOutput and ShaderEffect QML components to realize transparency for videos.
In order to use this component, it is necessary to correctly set up your videos in a special way. The reason for that is, that many video formats do not support alpha-channels for transparency. Especially important formats for final rendering with high compression rates can't be used. As file sizes and cross-platform compatibility are highly relevant for mobile games and apps, a restriction to only allow formats that support alpha-channels is not a viable solution.
But with a simple workaround, it is possible to use all available video formats. The basic idea is to split up the video into a color part and a transparency part. Look at the following example to see what the input video and the rendered video look like:
On the left side you can see the original input video. It contains two versions of the same animation:
The AlphaVideo component reads the input video and combines both videos parts into a single transparent video.
You can show such alpha-videos in your app or game, just like you would show a normal video without transparency. All properties, methods and signals of the Video QML component are also available for the AlphaVideo.
This small code snipped shows the alpha-video "AlphaAnimation.mp4" uniformly scaled to the scene size. The playback starts automatically and the video is infinitely looped.
import Felgo 3.0 import QtQuick 2.0 import QtMultimedia 5.0 GameWindow { id: gameWindow activeScene: scene screenWidth: 960 screenHeight: 640 Scene { id: scene // the "logical size" - the scene content is auto-scaled to match the GameWindow size width: 480 height: 320 // show alpha video AlphaVideo { source: "../assets/AlphaAnimation.mp4" autoPlay: true anchors.fill: scene loops: MediaPlayer.Infinite // playback loop } } }
For the loops property, you can use the enum MediaPlayer.Infinite
. That's why the statement import QtMultimedia 5.0
is necessary. For other properties,
like playbackState or fillMode, different enum types from the MediaPlayer or the VideoOutput component are used. If you don't need any of these
enum-types, you can skip the import and use the AlphaVideo directly.
The next section focuses on how to prepare an alpha-video, that can be used by the component.
If you already have a video animation, that you wan't to include in your project, several steps are required to correctly set up your alpha-video:
You can decide for yourself which software you want to use to create the video. The important thing is, that it's possible to render only the alpha-channel as a single video. The next section gives a step-by-step guide on how to create an alpha-video with Adobe After Effects.
The first step is to render your animation just with rgb-colors.
Render Queue
.Output Module Settings
, only select the RGB-channel.
Once you rendered the non-alpha part of your video, you can go on with rendering the alpha-channel. The procedure is nearly the same.
Render Queue
.Output Module Settings
, only select the Alpha-channel.
Now it's time to combine both videos into a single one.
With this you finished preparing your alpha-video, all thats left is to render and use it.
When rendering the final video, you can choose any video format or compression setting that you like. But keep in my mind that big file sizes should be avoided for mobile games and apps.
You can also use the Adobe Media Encoder for the final rendering. The Media Encoder supports many different formats and gives better control over compression rates and file sizes.
One of the most used and best supported formats is MP4, which is also what we recommend to use.
This property indicates if loading of the media should begin immediately. The default value is true
. If it is set to false
, media will not be loaded until playback is started
This property determines whether the media should begin playback automatically. Setting it to true
also sets autoLoad to true
. The default is
false
.
Returns the availability state of the alpha-video instance. This is one of:
Value | Description |
---|---|
MediaPlayer.Available | The video player is available to use. |
MediaPlayer.Busy | The video player is usually available, but some other process is utilizing the hardware necessary to play media. |
MediaPlayer.Unavailable | There are no supported video playback facilities. |
MediaPlayer.ResourceMissing | There is one or more resources missing, so the video player cannot be used. It may be possible to try again at a later time. |
Because the AlphaVideo type internally uses the MediaPlayer QML component, enumerations from MediaPlayer are used to access the availability state.
This property holds how much of the data buffer is currently filled, from 0.0
(empty) to 1.0
(full).
This property holds the duration of the media in milliseconds. If the media doesn't have a fixed duration (a live stream for example) this will be 0
.
This property holds the error state of the video. It can be one of:
Value | Description |
---|---|
MediaPlayer.NoError | There is no current error. |
MediaPlayer.ResourceError | The video cannot be played due to a problem allocating resources. |
MediaPlayer.FormatError | The video format is not supported. |
MediaPlayer.NetworkError | The video cannot be played due to network issues. |
MediaPlayer.AccessDenied | The video cannot be played due to insufficient permissions. |
MediaPlayer.ServiceMissing | The video cannot be played because the media service could not be instantiated. |
Because the AlphaVideo type internally uses the MediaPlayer QML component, enumerations from MediaPlayer are used to access the error state.
This property holds a string describing the current error condition in more detail.
Set this property to define how the video is scaled to fit the target area.
Value | Description |
---|---|
VideoOutput.Stretch | The video is scaled to fit. |
VideoOutput.PreserveAspectFit | The video is scaled uniformly to fit without cropping. |
VideoOutput.PreserveAspectCrop | The video is scaled uniformly to fill. It is cropped if necessary. |
Because the AlphaVideo type internally uses the VideoOutput QML component, enumerations from VideoOutput are used to access the available fill modes. The default fill mode is PreserveAspectFit
.
This property holds the number of times the media is played. A value of 0
or 1
means the media will be played only once. Set it to MediaPlayer.Infinite
to enable infinite looping. The
value can be changed while the media is playing, in which case it will update the remaining loops to the new value. The default is 1
.
This property may be used to access the video's meta-data. For more information, see the MediaPlayer documentation page.
The orientation of the video in degrees. Only multiples of 90
degrees are supported, that is 0
, 90
, 180
, 270
, 360
, etc.
This property holds the rate at which video is played at as a multiple of the normal rate.
This read only property indicates the playback state of the media.
Value | Description |
---|---|
MediaPlayer.PlayingState | The media is playing. |
MediaPlayer.PausedState | The media is paused. |
MediaPlayer.StoppedState | The media is stopped. |
Because the AlphaVideo type internally uses the MediaPlayer QML component, enumerations from MediaPlayer are used to access the playback state. The default state is MediaPlayer.StoppedState.
This property holds the current playback position in milliseconds. To change this position, use the seek() method.
This property holds whether the playback position of the video can be changed. If true
, calling the seek() method will cause playback to seek to the new
position.
This property holds the status of media loading. It can be one of:
Value | Description |
---|---|
MediaPlayer.NoMedia | No media has been set. |
MediaPlayer.Loading | The media is currently being loaded. |
MediaPlayer.Loaded | The media has been loaded. |
MediaPlayer.Buffering | The media is buffering data. |
MediaPlayer.Stalled | Playback has been interrupted while the media is buffering data. |
MediaPlayer.Buffered | The media has buffered data. |
MediaPlayer.EndOfMedia | The media has played to the end. |
MediaPlayer.InvalidMedia | The media cannot be played. |
MediaPlayer.UnknownStatus | The status of the media cannot be determined. |
As the AlphaVideo type internally uses the MediaPlayer QML component, enumerations from MediaPlayer are used to access the media loading status.
This property holds the volume of the audio output, from 0.0
(silent) to 1.0
(maximum volume).
This signal is emitted when playback is paused. The corresponding handler is onPaused
.
Note: The corresponding handler is onPaused
.
This signal is emitted when playback is started or continued. The corresponding handler is onPlaying
.
Note: The corresponding handler is onPlaying
.
This signal is emitted when playback is stopped. The corresponding handler is onStopped
.
Note: The corresponding handler is onStopped
.