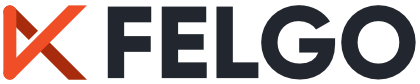
Sign Up and Download for Free
By signing up, you consent to Felgo processing your data & contacting you to fulfill your request. For more information on how we are committed to protecting & respecting your privacy, please review our privacy policy.
Already have a Felgo account? Login here.