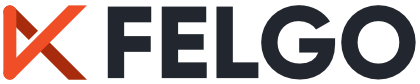
Base type for items to be placed in the left or right slots of NavigationBar. More...
Import Statement: | import Felgo 4.0 |
Inherits: | |
Inherited By: |
ActivityIndicatorBarItem, ButtonBarItem, and NavigationBarRow |
NavigationBar has a two slots for items: NavigationBar::leftBarItem and NavigationBar::rightBarItem. These items have to be derived from NavigationBarItem.
NavigationBarItems are also used for Pages, because these instantiate a NavigationBar automatically. If you use Pages in your app, use the properties AppPage::leftBarItem and AppPage::rightBarItem.
Typically, this type does not have to be used directly, as sub types for the most common use cases, like displaying a button icon or activity indicator exist already.
With the NavigationBarRow type, multiple items may be placed in the left or right slot.
To add custom items in a NavigationBar slot, derive them from NavigationBarItem.
The following example shows how to add a custom NavigationBarItem to the left item slot of a Page. Pushing the Page on the NavigationStack automatically places the NavigationBarItem in the left slot of the NavigationBar.
import Felgo import QtQuick App { NavigationStack { AppPage { title: qsTr("Main Page") // add a custom NavigationBarItem in the left slot of the NavigationBar leftBarItem: NavigationBarItem { // we specify the width of the item with the contentWidth property // the item width then includes the contentWidth and a default padding contentWidth: contentRect.width // the navigation bar item shows a colored rectangle Rectangle { id: contentRect width: dp(Theme.navigationBar.defaultIconSize) height: width anchors.centerIn: parent color: Theme.navigationBar.itemColor } } // NavigationBarItem } // Page } }
Note: To specify the item width use the contentWidth property. The NavigationBarItem then automatically adds a standard padding that matches the ThemeNavigationBar::defaultBarItemPadding setting.
[since Felgo 2.8.5] contentWidth : real |
The width of the NavigationBarItem is automatically based on the specified contentWidth and the padding setting. The
default value is a contentWidth of 0
. When creating a new NavigationBarItem, set this property to the desired width of the item content.
Note: Directly setting the item width property instead of contentWidth ignores the specified padding.
This property was introduced in Felgo 2.8.5.
See also padding.
[since Felgo 2.6.2] padding : real |
The width of the NavigationBarItem is automatically based on the contentWidth and the specified padding. The default padding value is ThemeNavigationBar::defaultBarItemPadding.
Note: The padding is not used when you set the item width property directly instead of specyfing a contentWidth.
This property was introduced in Felgo 2.6.2.
See also contentWidth.
[since Felgo 2.7.0] showItem : int |
This property only affects NavigationBarItems, that are placed within a NavigationBarRow. It defines whether the item is shown or hidden in the NavigationBarRow. For hidden items, the NavigationBarRow can automatically add a "show more" button that opens a submenu overlay for these items. Whether or not the "show more" button is added depends on the NavigationBarRow::showMoreButton setting.
The showItem property allows three options:
The default value is showItemIfRoom
for Android and showItemAlways
for iOS.
This property was introduced in Felgo 2.7.0.
See also NavigationBarItem::showItemNever, NavigationBarItem::showItemIfRoom, NavigationBarItem::showItemAlways, NavigationBarItem::title, and NavigationBarRow::showMoreButton.
[read-only, since Felgo 2.7.0] showItemAlways : int |
If used for NavigationBarItem::showItem, the item is always shown in the NavigationBar. Because the NavigationBarRow::showMoreButton feature is not used, this is the default setting on iOS to prevent hidden items.
This property was introduced in Felgo 2.7.0.
See also NavigationBarItem::showItem, NavigationBarItem::showItemNever, NavigationBarItem::showItemIfRoom, NavigationBarItem::title, and NavigationBarRow::showMoreButton.
[read-only, since Felgo 2.7.0] showItemIfRoom : int |
If used for NavigationBarItem::showItem, the item is only shown in the NavigationBar if there is enough room available. Otherwise it will be placed within a submenu. This is the default setting for NavigationBarItem::showItem on Android.
This property was introduced in Felgo 2.7.0.
See also NavigationBarItem::showItem, NavigationBarItem::showItemNever, NavigationBarItem::showItemAlways, NavigationBarItem::title, and NavigationBarRow::showMoreButton.
[read-only, since Felgo 2.7.0] showItemNever : int |
If used for NavigationBarItem::showItem, the item is never shown in the NavigationBar. Instead, it will always be placed within a submenu.
This property was introduced in Felgo 2.7.0.
See also NavigationBarItem::showItem, NavigationBarItem::showItemIfRoom, NavigationBarItem::showItemAlways, NavigationBarItem::title, and NavigationBarRow::showMoreButton.
[since Felgo 2.7.0] title : string |
This property is only relevant if the NavigationBarItem is placed within a NavigationBarRow. If the NavigationBarItem is not shown in the NavigationBar but hidden inside a submenu, the title of the NavigationBarItem specifies the text that is shown. The title is required whenever the setting showItemNever or showItemIfRoom is used and the feature NavigationBarRow::showMoreButton is set to true
.
This property was introduced in Felgo 2.7.0.
See also NavigationBarItem::showItem, NavigationBarItem::showItemNever, NavigationBarItem::showItemIfRoom, NavigationBarItem::showItemAlways, and NavigationBarRow::showMoreButton.