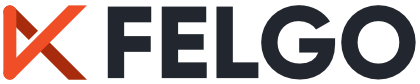
The ParallaxScrollingBackground allows to create an endlessly scrollable background, usable by SideScroller games for example. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
The ParallaxScrollingBackground uses one or two MultiResolutionImage instances next to each other to create an endless scrollable background. This can be used to create a parallax scrolling background effect in a game. Make sure you read our tutorial about How to create mobile games for different screen sizes and resolutions to learn about the recommended size for your background images.
The property sourceImage needs to bet set or the item won't work correctly. If sourceImage2 is empty, the image declared in sourceImage is mirrored as second image. It is mirrored vertically when scrolling into y direction and horizontally when scrolling into x direction.
Every image could be mirrored, but only a correct prepared image looks well afterwards. Following picture shows an image which can be used for mirroring. Both sides have the same height at the left and right edge and a very similar appearance as seen on the left side. It will look great when Image1, Image2 and again Image1 are beside each other as seen on the right side.
In contrast to the correct image from above, the follwoing image illustrates an incorrect picture for endless scrolling. The background looks faulty when the edges and appearance do not fit accordingly as seen on the left side. The incorrect result can be seen on the right side.
Furthermore, mirroring images only effects the fluctuation of your image data and does not matter if the images are well prepared. To avoid this problem, you can create one image which is big enough and includes variation. You could also prepare two images which fit together as seen in the picture below. There is only one important criteria: The left edge of Image1 needs to fit together with the right edge of Image2 as seen on the left side. The images will fit perfectly together when Image1, Image2 and again Image1 are beside each other as seen on the right side.
The ParallaxScrollingBackground can only move either horizontally or vertically. Directional changes along one axis (e.g. changing from left to right) is supported. Changing from horizontal to vertical movement or vice versa is not supported.
Examples for supported directions:
Following examples demonstrate the usage of the ParallaxScrollingBackground.
This example shows a single background image which leaves sourceImage2 empty to mirror sourceImage source file. The background scrolls with constant speed (10px per second) towards the left border with movementVelocity set to Qt.point(10,0)
. It scrolls with a ratio of 1/1
compared to its parent.
import Felgo import QtQuick GameWindow { Scene { ParallaxScrollingBackground { movementVelocity: Qt.point(10,0) ratio: Qt.point(1.0,1.0) sourceImage: "../assets/img/background-mountains.png" } } }
This example shows a single background image which leaves sourceImage2 empty to mirror sourceImage source file. The background scrolls with constant speed (10px per second) towards the bottom border with movementVelocity set to Qt.point(0,10)
. It scrolls with a ratio of 1/1
compared to its parent.
import Felgo import QtQuick GameWindow { Scene { ParallaxScrollingBackground { movementVelocity: Qt.point(0,10) ratio: Qt.point(1.0,1.0) sourceImage: "../assets/img/background-wood.png" } } }
This example shows two different backgrounds using sourceImage and sourceImage2.
The background scrolls with constant speed (50px per second) towards the right border with movementVelocity set to Qt.point(-50,0)
. It
scrolls with a ratio of 1/1
compared to its parent.
import Felgo import QtQuick GameWindow { Scene { ParallaxScrollingBackground { movementVelocity: Qt.point(-50,0) ratio: Qt.point(1.0,1.0) sourceImage: "../assets/img/background-hills.png" sourceImage2: "../assets/img/background-hills2.png" } } }
When a game should use different parallax effects it needs to use several items which move with different speeds. This case is demonstrated in this example.
import Felgo import QtQuick GameWindow { Scene { ParallaxScrollingBackground { movementVelocity: Qt.point(100,0) ratio: Qt.point(0.5,1.0) sourceImage: "../assets/img/background-mountains.png" } ParallaxScrollingBackground { id: background1 movementVelocity: Qt.point(-100,0) ratio: Qt.point(1.0,1.0) sourceImage: "../assets/img/background-hills.png" } ParallaxScrollingBackground { id: background2 movementVelocity: Qt.point(10,0) ratio: Qt.point(1.3,1.0) sourceImage: "../assets/img/background-lawn.png" } } // end of Scene } // end of GameWindow
Also see the Parallax Test for more information about the correct usage of the ParallaxScrollingBackground.
image : alias |
Note: The ParallaxScrollingBackground now has the size of the used MultiResolutionImage (sourceImage) by default. You can therefore center the ParallaxScrollingBackground (or other ways of anchoring) in the scene like you would do it with any other MultiResolutionImage.
You can still read the size of the used MultiResolutionImage with the image alias like shown in the example below. In this example it is used to position the ParallaxScrollingBackground in the center of the scene, however, the recommened approach is to just set anchors.centerIn: parent
.
import Felgo import QtQuick GameWindow { Scene { ParallaxScrollingBackground { id: pxBg sourceImage: "../assets/img/background-wood.png" x: -(pxBg.image.width-scene.width)/2 y: -(pxBg.image.height-scene.height)/2 mirrorSecondImage: false movementVelocity: Qt.point(-75, 0) } } // end of Scene } // end of GameWindow
mirrorSecondImage : bool |
Set this property if you want to mirror the sourceImage2 image. By default, if you only have specified the sourceImage property but no sourceImage2 property, the second image will be mirrored. If you explicitly set the sourceImage2 property, it will not be mirrored.
The mirroring is performed automatically based on the movementVelocity: if you set its x property, the image will be mirrored horizontally, or vertically when the y property is set.
movementVelocity : point |
The movementVelocity property is used to set the direction and speed of the movement.
ratio : point |
The ratio property is used to set how much shift should occur between parent and image.
running : bool |
If set to true
, the background images will scroll with the specified movementVelocity. If set to to false
, the scrolling
will pause. By default, it is set to true
.
sourceImage2 : url |
The sourceImage2 property is not always used. It can be used to provide an additional second image which will alternate with the sourceImage during scrolling. If the property sourceImage2 is left empty, the first image from the property sourceImage will be used and mirrored automatically.
sourceImage : url |
The sourceImage property is used to provide the image data for the background. This needs to be set accordingly or the ParallaxScrollingBackground will not work correctly.
resetBackgrounds() |
Reset the position of the images in the ParallaxScrollingBackground.