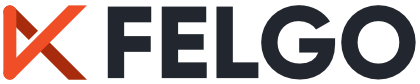
The PrismaticJoint allows relative translation of two bodies along a specified axis and prevents rotation. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
The PrismaticJoint is similar to the RevoluteJoint: just substitute translation for angle and force for torque. It translates two bodies around a common anchor point with a single degree of freedom: the relative translation of the two bodies, whereas the rotation stays fixed. The relative translation between the bodies is 0 after connection.
To control the translation between two bodies there are 2 options:
You can set the lowerTranslation and upperTranslation property to limit the translation between two bodies. To enable these limits, set enableLimit to true.
For applying a force about the localAnchorA, you can set the motorSpeed and maxMotorForce properties. For enabling them, set enableMotor to true.
For a comprehensive documentation of all joints see the Box2D documentation at http://www.box2d.org/manual.html#_Toc258082974.
The following example shows how two physics bodies connected with a PrismaticJoint. The motor force direction is reverted by clicking on the square. The source code of the full example is provided in the prismatic folder of the Box2D Examples.
import QtQuick 2.0 import Felgo 4.0 GameWindow { id: gameWindow // Timer that keeps creating heavy balls that crash down on the building Timer { running: true repeat: true interval: 1000 onTriggered: { var ballId = entityManager.createEntityFromUrlWithProperties( Qt.resolvedUrl("Ball.qml"), { // the radius of the ball is 20, so its width is 40 - generate numbers between 20 and world.width-20 x: Math.random() * (world.width-40) + 20, y: -100 }) } } MouseArea { anchors.fill: parent onClicked: { // invert direction where movingSquare is pulled to prismaticJoint.motorSpeed *= -1 } } Scene { id: scene PhysicsWorld { id: world anchors.fill: parent z:1 // for putting the debugDraw on top of the entities in QML renderer gravity.y: 20 updatesPerSecondForPhysics: 60 } // this square is moved right and left with a motor speed Square { id: movingSquare x: 50 y: 80 width: 60 height: 40 } // this square stays in the center Square { id: staticMiddleSquare // the world size is 480x320 x: 240 y: 160 width: 80 height: 20 color: "red" bodyType: Body.Static } PrismaticJoint { id: prismaticJoint lowerTranslation: -30 upperTranslation: 120 enableLimit: true maxMotorForce: 3000 motorSpeed: -10 enableMotor: true bodyA: staticMiddleSquare.body bodyB: movingSquare.body // the center of the joint will be at the top left corner of middle Square boxCollider by default // to change that, you could use the following: //localAnchorA: Qt.point(staticMiddleSquare.width/2, staticMiddleSquare.height/2) // only allow movement along the horizontal axis axis: Qt.point(1, 0) } Wall { id: ground height: 20 anchors { left: parent.left; right: parent.right; top: parent.bottom } } Wall { id: leftWall width: 20 anchors { right: parent.left; bottom: ground.top; top: parent.top } } Wall { id: rightWall width: 20 anchors { left: parent.right; bottom: ground.top; top: parent.top } } Text { z: 1 // put on top of DebugDraw in QML renderer color: "white" text: "Click on the screen to toggle the direction of the PrismaticJoint motor speed" } } // end of Scene EntityManager { id: entityManager entityContainer: scene } }
enableLimit : bool |
Set this property to true for lowerTranslation and upperTranslation to have an effect. By default, it is false.
enableMotor : bool |
Set this property to true for maxMotorForce and motorSpeed to have an effect. By default, it is false.
localAnchorA : point |
The local anchor point relative to the Joint::bodyA center in pixels.
The default is (0, 0), which means the center of Joint::bodyA.
localAnchorB : point |
The local anchor point relative to the Joint::bodyB center in pixels.
The default is (0, 0), which means the center of Joint::bodyB.
localAxisA : point |
The local translation axis relative Joint::bodyA is moved upon. Only the direction of the vector and not the length matters.
The default is 0/0.
lowerTranslation : real |
The lower limit for the joint translation range in pixels (it is in the same unit as the size of the bodies). To enable it, set enableLimit to true.
Note: The limit range should include zero, otherwise the joint will lurch when the simulation begins.
maxMotorForce : real |
The maximum force in kg*pixels/second^2. To enable it, set enableMotor to true.
The joint motor will maintain the specified motorSpeed unless the required force exceeds this maximum. When the maximum force is exceeded, the joint will slow down and can even reverse.
motorSpeed : real |
The speed of the motor in degrees per second. To enable it, set enableMotor to true.
upperTranslation : real |
The upper limit for the joint translation range in pixels (it is in the same unit as the size of the bodies). To enable it, set enableLimit to true.
Note: The limit range should include zero, otherwise the joint will lurch when the simulation begins.