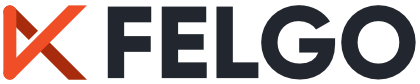
The RubeParser creates QML items based on the description of a JSON file exported by the RUBE level editor. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
The RubeParser creates QML items based on the description of a JSON file exported by the RUBE level editor.
Add the RubeParser to your scene and call the setupLevelFromJSON function like in this example:
import Felgo import QtQuick import "Rube" GameWindow { Scene { id: scene EntityManager { id: entityManager entityContainer: level } Item { id: level PhysicsWorld { id: physicsWorld } } RubeParser { id: parser entitiesFolder: "entities/" Component.onCompleted: { console.debug("load finished, start parsing") parser.setupLevelFromJSON( Qt.resolvedUrl("../assets/sidescroller.json"), physicsWorld, level ) } } } }
Just call setupLevelFromJSON().
The parameters are:
jsonPath
- a path to a .json file as stringworld
- the id of your game's physics worldlevelContainer
- the id of an item, the created RubeBodies will be children of this itemAt first, the JSON file is read and loaded into a JSON-object in the memory by the setupLevelFromJSON() function. Then, this function calls parseJSON() passing through all the parameters. This function reads the settings from the JSON and applies them to the physics world. The parseJSON() function calls the createBodies() with a list of body objects from the JSON, the world and the levelContainer. The createBodies() function creates objects from the Rube Components. If the custom property "qmlType" is set it tries to create an object of this type. Then the signal internalInit is emitted on this new body causing it to initialize its properties from the transmitted JSON. For each created body the addFixturesToBody() function is called, which creates objects of the different fixture components and attaches them to the body. Each fixtures has its internalInit signal emitted. When all fixtures are attached to the body the fixturesFinished signal is emitted on it. Afterwards, the initialized signal is emitted on the body giving the users a chance to react to the finished object. When all bodies are finished the createImages() function is called, which does the same as the createBodies() but with images. If the image belongs to a body (as said in the JSON) it is assigned as a child to this body. The the renderOrder aka. z in Qt is applied and the image if it doesn't belong to any body or to its parent body. This is because the z property works only among siblings. Then, internalInit and initialized are emitted on the image just like the body before.
In short:
Just call clearLevel. All created RubeBodies with their fixtures and all RubeImages will be destroyed.
Following properties are read from the JSON and applied to the World given as parameter:
Following properties are not yet supported:
Following properties are read from the JSON and applied to the RubeBody instances:
The RubeBody contains a list of fixtures Body::fixtures, too.
Following properties are read from the JSON and applied to all the different RubeFixture instances:
Following properties are read from the JSON and applied to the RubeFixtureCircle instances:
Following properties are read from the JSON and applied to the PolygonFixture instances:
The RUBE fixture type "line" and "loop" are not supported at the moment.
Following properties are read from the JSON and applied to the RubeImage instances:
Joints are not yet supported
entitiesFolder : url |
Set this folder to the relative path where the game entities are located. The game entities are set with the qmlType
custom property in RUBE.
If your entities are in "qml/entities/"
, and your current QML file is in the qml folder, set the path to "entities/"
.
Note: By default, it is set to "../qml/entities"
relative to the assetsFolder (the folder where your rube .json file is located). However, when you create a publish build of your game and protect your QML files, you need to set this path explicitly.
clearLevel() |
Destroys all RubeBodies with their fixtures and all RubeImages.
setupLevelFromJSON(jsonPath, world, levelContainer) |
Reads the given JSON file from jsonPath
and creates RubeBodies and RubeImages according to the definition. All created objects will be placed in levelContainer
. The world
property
holds a reference to the game's PhysicsWorld.