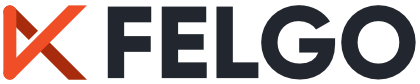
The root element for a single game view. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
This component contains the logic for auto-scaling its content (i.e. its children) to the parent GameWindow item.
The content of a Scene is scaled from its logical size to the GameWindow size depending on the scaleMode. This allows to use the same code for all screen resolutions. So if the Scene size is set to 480x320 for instance, the contents will be scaled by a factor of 2 with a GameWindow size of 960x640.
The simplest Felgo application consists of a GameWindow and a Scene. The scene has a default size of 480x320 and the scaleMode is letterbox
. The blue rectangle fills the whole GameWindow and is anchored to its bottom. You can resize
the GameWindow on your desktop by pressing the keyboard shortcuts ctrl(cmd)+1-7
to see the effect of different screen sizes. Fullscreen can be changed by pressing the keyboard
button ctrl(cmd)+F
.
import QtQuick // for the Rectangle element import Felgo // for the GameWindow and Scene element GameWindow { Scene { id: scene // this Scene is centered horizontally and vertically by default Rectangle { color: "green" width: 100 height: 50 } // this Rectangle has the size of the whole GameWindow (not only the logical Scene) // it is also anchored to the bottom of it Rectangle { color: "blue" width: scene.gameWindowAnchorItem.width height: 20 anchors.bottom: scene.gameWindowAnchorItem.bottom } } }
Also see the GameWindowSceneScaling Example for more information about different scaleModes.
See also GameWindow.
[since Felgo 2.1.1] backButtonAutoAccept : bool |
Set this property to false if you want to handle the back button events manually. If this is false, the event.accepted
property of the key event is not set to true. This causes the closing of the app on
Android, if you do not handle the back button event on your own.
The default value is true and prevents closing of the app on Android when the Scene has active focus.
This property was introduced in Felgo 2.1.1.
See also backButtonPressed.
[since Felgo 2.1.1] backButtonSimulationEnabled : bool |
This property holds whether a Qt.Key_Backspace keyPressed event calls the backButtonPressed signal.
By default, it is set to system.desktopPlatform
which is only true on Windows, Mac and Linux but false on iOS and Android.
With this property you can simplify testing the back button logic also on Desktop.
This property was introduced in Felgo 2.1.1.
See also backButtonPressed.
[since Felgo 2.2.0] dpScale : real |
[since Felgo 2.16.0] fullWindowAnchorItem : alias |
Modern devices like iPhone X may display buttons and overlays in certain areas of the screen. The fullWindowAnchorItem can be used for aligning direct children of a Scene to the full screen, regardless of such reserved areas. For anchoring items with respect to the safe area of the screen, you can use the gameWindowAnchorItem.
import Felgo import QtQuick GameWindow { id: gameWindow Scene { id: scene // fills the whole screen, including reserved areas Rectangle { anchors.fill: scene.fullWindowAnchorItem color: "lightblue" } // fills the game window, which only covers the safe area of the screen Rectangle { anchors.fill: scene.gameWindowAnchorItem color: "lightgreen" } // fills the scene, which is scaled to fit into the game window Rectangle { anchors.fill: parent color: "lightyellow" Text { anchors.centerIn: parent text: "Scene" font.pixelSize: 20 } } } }
Note: You can also access NativeUtils::safeAreaInsets to work with the safe area. If you do so, keep in mind that the returned pixel value does not take scene-scaling into consideration. It is required to manually apply the used scale factor in this case.
This property was introduced in Felgo 2.16.0.
See also gameWindowAnchorItem and useSafeArea.
gameWindowAnchorItem : alias |
This Item can be used for anchoring direct children of a Scene to the parent GameWindow and not the logical Scene size. For example use anchors.bottom:
gameWindowAnchorItem.bottom
to position an item to the bottom of the GameWindow.
Note: Certain devices, like iPhone X, use reserved areas of the screen for overlays and buttons. The gameWindowAnchorItem respects these safe areas of the screen. To align items of your scene to the whole screen on such devices, you can use the fullWindowAnchorItem instead.
import Felgo import QtQuick GameWindow { id: gameWindow Scene { id: scene // fills the whole screen, including reserved areas Rectangle { anchors.fill: scene.fullWindowAnchorItem color: "lightblue" } // fills the game window, which only covers the safe area of the screen Rectangle { anchors.fill: scene.gameWindowAnchorItem color: "lightgreen" } // fills the scene, which is scaled to fit into the game window Rectangle { anchors.fill: parent color: "lightyellow" Text { anchors.centerIn: parent text: "Scene" font.pixelSize: 20 } } } }
Note: You can also access NativeUtils::safeAreaInsets to work with the safe area. If you do so, keep in mind that the returned pixel value does not take scene-scaling into consideration. It is required to manually apply the used scale factor in this case.
See also How to create mobile games for different screen sizes and resolutions, GameWindowSceneScaling Example, fullWindowAnchorItem, and useSafeArea.
gridSize : real |
The gridSize can be set when the level editing feature is used and the entities should be snapped to a raster. The default gridSize is 16. So for instance for a 480x320 scene with gridSize 16, a 30x20 grid is created.
If you don't want to use entity snapping, disable it by setting the gridSize to 1
.
scaleMode : string |
This property specifies how the Scene gets scaled its contents to the parent GameWindow.
There are 4 scaleMode settings available:
By default, this property is set to the GameWindow default scaleMode which is letterbox
. It can however be set for each scene individually too.
See also AppItem::scaleMode and How to create mobile games for different screen sizes and resolutions.
sceneAlignmentX : string |
This property defines the horizontal position of the Scene based on the scaleMode and the GameWindow size.
It can have the values "left"
, "center"
and "right"
. The default value is center
.
Also see the GameWindowSceneScaling Example for more information about different scaleModes.
See also sceneAlignmentY.
sceneAlignmentY : string |
This property defines the vertical position of the Scene based on the scaleMode and the GameWindow size.
It can have the values "top"
, "center"
and "bottom"
. The default value is center
.
Also see the GameWindowSceneScaling Example for more information about different scaleModes.
See also sceneAlignmentX.
sceneGameWindow : variant |
This property can be set if the Scene is not a direct child of the GameWindow. By default, this property is set to parent.
It is used internally to calculate the top left position based on the scaleMode.
[since Felgo 2.2.0] spScale : real |
[since Felgo 2.2.0] uiScale : real |
useSafeArea : bool |
Modern devices like iPhone X may display buttons and overlays in certain areas of the screen. The Scene automatically scales its content to not overlay these reserved areas. Setting useSafeArea
to
false
allows the Scene to fill the whole screen, regardless of safe area insets.
To align items in your scene with the safe area of the screen, you can use the gameWindowAnchorItem. Items of your scene that should cover the whole screen can be anchored to fullWindowAnchorItem.
See also fullWindowAnchorItem and gameWindowAnchorItem.
xScaleForScene : real |
This property contains the applied scale factor for the children items of Scene. It gets internally calculated based on the scaleMode, the Scene size and the GameWindow size. Use this property if you want to write custom items that depend on the content scaling factor.
The xScaleForScene and yScaleForScene property are equal when scaleMode is set to
letterbox
or zoomToBiggerSide
. They are both 1 when scaleMode is set to none
.
Internally, Felgo uses this scaleFactor to determine which image version should be loaded, so with an sd, hd or hd2 suffix. See MultiResolutionImage for this.
See also yScaleForScene.
yScaleForScene : real |
This property contains the applied scale factor for the children items of Scene. It gets internally calculated based on the scaleMode, the Scene size and the GameWindow size. Use this property if you want to write custom items that depend on the content scaling factor.
The xScaleForScene and yScaleForScene property are equal when scaleMode is set to
letterbox
or zoomToBiggerSide
. They are both 1 when scaleMode is set to none
.
Internally, Felgo uses this scaleFactor to determine which image version should be loaded, so with an sd, hd or hd2 suffix. See MultiResolutionImage for this.
See also xScaleForScene.
|
This signal is emitted when the back button (Qt.Key_Back) was pressed. Handling the back button is required for a good user experience of your game on Android.
This signal is emitted when the Scene gets Item::focus. This is done automatically if AppItem::activeScene is set to this Scene.
For an example with back button handling per scene, see Advanced Example with Android Back Button and Focus Handling.
To prevent the app from accepting the back button event when it has focus, set backButtonAutoAccept to false. If you change this setting, do accept the back button event manually to avoid closing the application on Android when the back button is pressed.
When testing an app, it is often useful to simulate the back button handling and logic on Desktop platforms Windows, Mac and Linux. This is enabled by default when you press the Qt.Key_Backspace key. To disable this simulation, set backButtonSimulationEnabled to false.
The corresponding handler is onBackButtonPressed
.
Note: The corresponding handler is onBackButtonPressed
.
This signal was introduced in Felgo 2.1.1.
|
Returns the density-independent unit for this pixel value
for children of Scene components.
This allows you to define the same physical size for Item elements across platforms and screens with different densities.
DPI Examples
The dp value is calculated with the following formula: dp = pixel * screenDpi / 160
This means:
Although the pixel values are different, the physical size of them is the same!
160 dp equals 1 inch. You can calculate the inch value from the pixel value with pixelToInches().
Note: The recommended button height is 48dp. This equals 3/10 of an inch or 0.8 centimeters.
You can modify the result of all dp() function calls by changing the dpScale or uiScale properties.
Example Usage
import Felgo import QtQuick GameWindow { id: gameWindow Scene { id: gameScene // position on top of the GameWindow sceneAlignmentY: "top" Rectangle { width: parent.width height: gameScene.dp(48) Text { text: "20sp" font.pixelSize: gameScene.sp(20) } } }// Scene // this Rectangle & Text has the same size as the one defined in the Scene Rectangle { // position below the Rectangle of the Scene y: gameWindow.dp(48) width: parent.width height: gameWindow.dp(48) Text { text: "20sp" font.pixelSize: gameWindow.sp(20) } }// Rectangle }
Note: To calculate the dp value of elements that are NOT children of a Scene but from GameWindow, use AppItem::dp().
Also see the guide Density Independence Support: dp, sp, pixelToInches, tablet, orientation for more information on density independence. The Android Developers Guide about Supporting Multiple Screens is also a great read to better understand density independence, as the same concepts of Android are used in Felgo.
This method was introduced in Felgo 2.2.0.
|
Helper function to return the Scene pixel
value from a GameWindow pixel value.
The pixel
value is divided by xScaleForScene.
You can use this function to convert for example a GameWindow position to a Scene position.
This method was introduced in Felgo 2.2.0.
See also fromGameWindowPixel().
|
Returns the pixel value multiplied with the devicePixelRatio
of the screen.
On iOS & Mac devices, the reported screen size of GameWindow is a virtual point size. To get the real pixels of the screen, a multiplication with devicePixelRatio is needed.
You will mostly not need this value, but it may be useful for debug output or certain scenarios.
Note: The physicalPixels value of the screen is used in Felgo to choose the default Felgo File Selectors to support Dynamic Image Switching.
This method was introduced in Felgo 2.2.0.
|
|
Returns the density-independent unit for this pixel value
for children of Scene components. Only use this function for Text element
font.pixelSize
values.
This allows you to define the same physical size for Text elements across platforms and screens with different densities.
DPI Examples
The dp value is calculated with the following formula: dp = pixel * screenDpi / 160
This means:
Although the pixel values are different, the physical size of them is the same!
160 dp equals 1 inch. You can calculate the inch value from the pixel value with pixelToInches().
Note: The recommended button height is 48dp. This equals 3/10 of an inch or 0.8 centimeters.
You can modify the result of all sp() function calls by changing the spScale or uiScale properties.
Note: The only difference to dp() is that you have a different spScale value available to change the scale factor of Text elements. You could read the user settings for font sizes of the system and change it accordingly. This is done by default for native Android applications - you could implement this function yourself by querying the user font settings and then changing the spScale value.
You could also use the spSale to allow users change the font size in your app at runtime, for example with a slider in a settings scene.
Example Usage
import Felgo import QtQuick GameWindow { id: gameWindow Scene { id: gameScene // position on top of the GameWindow sceneAlignmentY: "top" Rectangle { width: parent.width height: gameScene.dp(48) Text { text: "20sp" font.pixelSize: gameScene.sp(20) } } }// Scene // this Rectangle & Text has the same size as the one defined in the Scene Rectangle { // position below the Rectangle of the Scene y: gameWindow.dp(48) width: parent.width height: gameWindow.dp(48) Text { text: "20sp" font.pixelSize: gameWindow.sp(20) } }// Rectangle }
Note: To calculate the sp value of elements that are NOT children of a Scene but from GameWindow, use AppItem::dp().
Also see the guide Density Independence Support: dp, sp, pixelToInches, tablet, orientation for more information on density independence. The Android Developers Guide about Supporting Multiple Screens is also a great read to better understand density independence, as the same concepts of Android are used in Felgo.
This method was introduced in Felgo 2.2.0.
|
Helper function to return the GameWindow pixel
value from a Scene Window pixel value.
The pixel
value is multiplied by xScaleForScene.
You can use this function to convert for example a Scene position to a GameWindow position.
This method was introduced in Felgo 2.2.0.
See also fromGameWindowPixel().