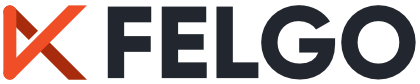
The root element for rendering 3D objects within a 2D GameWindow. More...
Import Statement: | import Felgo 3.0 |
Since: | Felgo 2.5.0 |
Inherits: |
The Scene3D component is the root element to display 3D objects within a 2D GameWindow.
In the Qt3D module, you have several options to display 3D content. However, using the Scene3D component is the most flexible one because you can then mix 2D UI with 3D rendering.
It is based on a Scene3D component with these additional benefits:
Note: Qt3D and also the Felgo 3D Components are in a Tech Preview state and may change its API in future versions.
Here is an example how to use Scene3D and Render3D in a Felgo project:
import Felgo 3.0 import QtQuick 2.0 GameWindow { // is needed by the Render3D component - to this entity the Entity objects are added to property alias rootEntity: scene3D.rootEntity Scene { id: gameScene Level { EntityBase { entityType: "car" // this Box2D physics component modifies the entity position by applying forces // when the entity position changes, the Render and Image components change too BoxCollider {} // allows loading a Mesh and a texture // registers itself at the rootEntity Render3D { source: "assets/3d/car_model.obj" texture: "assets/3d/car_texture.png" } // you could mix 2D images with the 3D renderer like this Image { source: "assets/2d/car.png" } }// EntityBase }// Level }// GameScene Scene3D { id: scene3D } Scene { id: hudScene // put user elements like buttons here } }
For a complete game how to use Scene3D together with the Render3D component, see the CarChallenge 3D Demo.
isControlledCamera : bool |
Set this property to set Configuration::controlledCamera of the default camera to true.
If you rotate the world by using the default input aspect of the camera, this can lead to unwanted rotation effects.
By default it is set to true, unless you defined a custom frameGraph.
This property provides a way to control the number of times to repeat the sound on each play().
By default, loops is set to Audio.Infinite
and therefore plays the sound infinitely until it gets stopped by calling stop().
Note: To have access to the Audio.Infinite
enum, make sure to call import QtMultimedia 5.0
in the header of your QML file.
Read-only property of the used SkyboxEntity.
Folder name string of the skybox cubemap. The folder must contain 6 images, named:
<skyboxBasename> + _negx.<skyboxExtension>
<skyboxBasename> + _posx.<skyboxExtension>
<skyboxBasename> + _negy.<skyboxExtension>
<skyboxBasename> + _posy.<skyboxExtension>
<skyboxBasename> + _negz.<skyboxExtension>
<skyboxBasename> + _posz.<skyboxExtension>
For example, if skyboxBaseName is set to "Qt.resolvedUrl(../assets/3d/cubemaps/mountains)"
and the skyboxExtension is ".bmp"
, these are the
expected skybox images:
assets/3d/cubemaps/mountains/mountains_negx.bmp
assets/3d/cubemaps/mountains/mountains_posx.bmp
assets/3d/cubemaps/mountains/mountains_negy.bmp
assets/3d/cubemaps/mountains/mountains_posy.bmp
assets/3d/cubemaps/mountains/mountains_negz.bmp
assets/3d/cubemaps/mountains/mountains_posz.bmp
If you leave this property empty, the SkyboxEntity is disabled.
See also skyboxExtension.
RotationAngle of the SkyboxEntity.
RotationAxis of the SkyboxEntity.
The file ending of the 6 skybox images. By default it is set to "png"
.
See also skyboxBaseName.