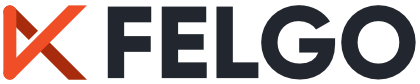
A search bar with native styling for iOS and Android based on the Theme settings. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 2.9.2 |
Inherits: |
The SearchBar control provides a native-looking search input field for Android and iOS based on the Theme settings.
iOS | Android |
|
|
Note: The item offers two different styles for the search bar on iOS. To switch to the second style, which is used in the image above, set iosAlternateStyle
to true
.
import Felgo App { NavigationStack { AppPage { title: "SearchBar Example" SearchBar { id: searchBar onAccepted: { console.log("search accepted: "+text) listView.model = 2 // update result } } AppListView { id: listView anchors.top: searchBar.bottom model: 50 delegate: SimpleRow { text: "Entry "+index } } } // Page } }
The SearchBar can also be combined with a ListView to hide the bar by default and only show it after pulling down the list. To use this feature, activate pullEnabled and specify the target ListView.
import Felgo App { NavigationStack { AppPage { title: "SearchBar Example" SearchBar { id: searchBar target: listView pullEnabled: true onAccepted: { console.log("search accepted: "+text) // if the search bar is not empty, we prevent it from disappearing if(text !== "") { searchBar.keepVisible = true listView.model = 2 // update result } else { // initial settings searchBar.keepVisible = false listView.model = 50 } } } AppListView { id: listView height: parent.height - y // the search bar modifies the y-position of the list model: 50 delegate: SimpleRow { text: "Entry "+index } } } // Page } }
Note: The pullEnabled setting modifies the y-position of the ListView, so we decrease its height in the example to always exactly fill the page.
barBackgroundColor : color |
The background color of the search bar. Matches platform-specific values by default.
[since Felgo 3.9.1] clearButtonSize : real |
The size of the clear button. The default value matches Theme::defaultIconSize.
This property was introduced in Felgo 3.9.1.
iconColor : color |
The color of the search icon. Matches Theme::tintColor if the search field has focus, Theme::placeholderTextColor otherwise.
iconLeftPadding : real |
The padding to the left of the search icon. The default value is 0 on iOS, else 7 dp
.
[since Felgo 3.9.1] iconRightPadding : real |
The padding to the right of the clear icon. The default value is 0 on iOS, else -5 dp
.
This property was introduced in Felgo 3.9.1.
[since Felgo 3.9.1] iconSize : real |
The size of the search icon. The default value matches Theme::defaultIconSize.
This property was introduced in Felgo 3.9.1.
iconSpacing : real |
The spacing to the right of the search icon. The default value is 10 dp
.
iconType : string |
The search icon, matches IconType.search
by default.
Note: This property was renamed from icon
in Felgo 4.0.0. This is because many types already inherit a property AbstractButton::icon from Qt Quick Controls 2.
inputBackgroundColor : color |
The background color of the input field. Matches platform-specific values by default.
iosAlternateStyle : bool |
Set this property to true
to use select an alternate search-bar style on iOS.
Default Style | Alternate Style |
|
|
keepVisible : bool |
If pullEnabled is activated and the search bar is linked with a ListView target, the search bar will stay visible as long as this property is true
. The default is false
, which hides the search bar if the list is scrolled down
again.
See also target and pullEnabled.
placeHolderColor : alias |
The color of the placeholder text in the input field. Matches Theme::placeholderTextColor by default.
placeHolderText : string |
The placeholder text of the search input field. The default value is Search
.
pullEnabled : bool |
If set to true
and the search bar is linked with a ListView target, the search will become
visible by dragging down the list while it is on top position.
Note: The target ListView's y-position will be set to match the search bar height (to show the search) or zero (to hide the search).
See also target and keepVisible.
[since Felgo 2.10.0] showClearButton : bool |
If set to true
, a clear button is displayed at the right side of the search bar as soon as the text field has content, as a way for the user to remove the current text.
The default value is true
.
This property was introduced in Felgo 2.10.0.
[since Felgo 3.3.0] showDivider : bool |
Shows a divider at the bottom of the search bar. The default value is false
.
This property was introduced in Felgo 3.3.0.
target : ListView |
If pullEnabled is set to true, the search bar will be linked to the target ListView to only become visible when pulling down the list.
Note: The target ListView's y-position will be set to match the search bar height (to show the search) or zero (to hide the search). Please do not anchor the list-view or set a custom y-position if you want to use this feature.
See also pullEnabled and keepVisible.
text : alias |
The text of the search input field.
textColor : alias |
The color of entered text in the search input field. The default value matches Theme::textColor.
[since Felgo 2.16.1] textField : alias |
Use this property to access the internal AppTextField item of the search bar.
This property was introduced in Felgo 2.16.1.
accepted(string text) |
This signal is emitted when the Return or Enter key is pressed by the user to trigger the search.
Note: The corresponding handler is onAccepted
.
editingFinished(string text) |
This signal is emitted when the Return or Enter key is pressed or the text field loses focus.
Note: The corresponding handler is onEditingFinished
.
hide() |
Hides the search bar by modifying the target ListView's y-position.
See also target, pullEnabled, and keepVisible.
show() |
Shows the search bar by modifying the target ListView's y-position.
See also target, pullEnabled, and keepVisible.