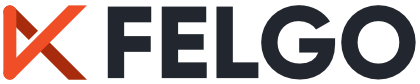
A global object for defining app-wide styling attributes. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
All apps created with Felgo Apps ship with a default style which follows platform-specific attributes. For most flexibility it's however also possible to overwrite these styles by your own.
The most basic example is changing the background color of a navigation bar within a NavigationStack item.
To change a theme-able property implement the App::initTheme signal handler and overwrite the values like in this example:
import Felgo App { onInitTheme: { // Set the navigation bar background to a shiny blue Theme.navigationBar.backgroundColor = "blue" // Set the global text color to a dark blue Theme.colors.textColor = "#000080" } }
Note: You can change theme-able properties at any given time from within your app's code, not only in the App::initTheme handler. The recommended approach is however to use the given handler for setting a default style at app start.
The default Theme settings implement platform-specific styles, like the default iOS style on iOS or Material Style on Android, for a native look and feel on both platforms. The following example shows how to manually change the used platform theme in your code:
import Felgo App { Navigation { NavigationItem { title: "Main" iconType: IconType.heart NavigationStack { AppPage { title: "Main Page" AppButton { text: "Switch Theme" onClicked: Theme.platform = (Theme.platform === "android") ? "ios" : "android" anchors.centerIn: parent } } } } NavigationItem { title: "Second" iconType: IconType.thlarge NavigationStack { AppPage { title: "Second Page" } } } } }
Felgo components make use of two different fonts, the Theme::normalFont and the Theme::boldFont. By default, these fonts match the platform's default font for Felgo Apps components.
If you want to explicitly provide your own font you can override the Theme properties with a FontLoader object:
import Felgo import QtQuick App { // initialize theme with new font onInitTheme: { Theme.normalFont = arialFont } // load new font with FontLoader FontLoader { id: arialFont source: "../assets/fonts/Arial.ttf" // loaded from your assets folder } AppPage { // Felgo components like AppText automatically use the Theme fonts AppText { text: "I'm in Arial" } } }
Make sure to add your custom fonts to your app's assets and to provide the correct path in the FontLoader object. You can also use the custom font in your own app components, like the following example:
Text { // Set reference to the global app font font.family: Theme.normalFont.name text: "Custom text item" }
Instead of replacing the general Theme font, you can also use a FontLoader and only overwrite the font.family
property for certain text items.
Find more examples for frequently asked development questions and important concepts in the following guides:
appButton : ThemeAppButton |
You can override sub-properties of the appButton property to set the global appearance of AppButton items.
Following properties can be overridden:
true
.
true
.
false
.
true
.
[since Felgo 2.7.1] appCheckBox : ThemeAppCheckBox |
You can override sub-properties of the appCheckBox property to set the global appearance of AppCheckBox items. See the documentation of the ThemeAppCheckBox type for an overview of available properties.
This property was introduced in Felgo 2.7.1.
[since Felgo 3.7.0] appRadio : ThemeAppRadio |
You can override sub-properties of the appRadio property to set the global appearance of AppRadio items. See the documentation of the ThemeAppRadio type for an overview of available properties.
This property was introduced in Felgo 3.7.0.
[read-only] backgroundColor : color |
Read-only alias for colors.backgroundColor.
boldFont : QtObject |
Override this property to change the app-wide default bold font. It can either be set to a FontLoader object, or a simple QtObject with a name property, defining the used font name.
If not changed, the default font of an empty Text item is used.
colors : ThemeColors |
You can override sub-properties of the colors property to change global colors for the various AppSDK controls.
Following properties can be overridden:
[since Felgo 3.3.0] contentPadding : real |
This property holds the default padding that is used for the content. This padding is also used for navigation bar left/right items, the navigation drawer icon, the padding in rows etc.
Use this padding to streamline your content with the existing layout.
The default value is 16
on Android and 15
for iOS and other platforms.
Note: Set this property without wrapping it with dp()
. If you use this property in your custom code, wrap it with dp()
.
This property was introduced in Felgo 3.3.0.
[read-only, since Felgo 2.8.2] controlBackgroundColor : color |
Read-only alias for colors.controlBackgroundColor.
This property was introduced in Felgo 2.8.2.
[since Felgo 3.9.1] defaultIconSize : real |
[since Felgo 2.7.1] dialog : ThemeDialog |
You can override sub-properties of the dialog property to set the global appearance of Dialog items and inherited components like InputDialog. See the documentation of the ThemeDialog type for an overview of available properties.
This property was introduced in Felgo 2.7.1.
[read-only] disabledColor : color |
Read-only alias for colors.disabledColor.
[read-only] disclosureColor : color |
Read-only alias for colors.disclosureColor.
[read-only] dividerColor : color |
Read-only alias for colors.dividerColor.
[read-only, since Felgo 2.7.0] inputCursorColor : color |
Read-only alias for colors.inputCursorColor.
This property was introduced in Felgo 2.7.0.
[read-only, since Felgo 2.7.0] inputSelectionColor : color |
Read-only alias for colors.inputSelectionColor.
This property was introduced in Felgo 2.7.0.
[read-only] isAndroid : bool |
Read-only property returning true
if the app is currently running on an Android device.
You can use this property to change your app's style or logic depending on the underlying platform. The following example shows how to use a different navigation bar color for Android devices:
import Felgo App { onInitTheme: { // Set the navigation bar background to a shiny blue on Android only Theme.navigationBar.backgroundColor = Theme.isAndroid ? "blue" : "red" } }
[read-only] isDesktop : bool |
Read-only property returning true
if the app is currently running on an desktop operating system, i.e. Windows, macOS (Mac OS X) or Linux.
You can use this property to change your app's style or logic depending on the underlying platform.
[read-only, since Felgo 4.0] isInitialized : bool |
This property is set to true after App::initTheme() was called.
You can use this to check if the theme is already initialized.
It is a good idea to only play animations after initializing the theme. This prevents parts of your UI animating their e.g. background colors on app startup.
For example, only enable Behavior after the theme is initialized:
import Felgo import QtQuick App { onInitTheme: { Theme.colors.backgroundColor = "black" } Rectangle { anchors.fill: parent color: Theme.backgroundColor Behavior on color { // do not animate the initial color change during onInitTheme enabled: Theme.isInitialized ColorAnimation { duration: 150; easing.type: Easing.InOutQuad } } } }
This property was introduced in Felgo 4.0.
[read-only] isIos : bool |
Read-only property returning true
if the app is currently running on an iOS device.
You can use this property to change your app's style or logic depending on the underlying platform. The following example shows how to use a different navigation bar color for iOS devices:
import Felgo App { onInitTheme: { // Set the navigation bar background to a shiny blue on iOS only Theme.navigationBar.backgroundColor = Theme.isIos ? "blue" : "red" } }
[read-only] isLinux : bool |
Read-only property returning true
if the app is currently running on a Linux operating system.
You can use this property to change your app's style or logic depending on the underlying platform. The following example shows how to use a different navigation bar color for Linux devices:
import Felgo App { onInitTheme: { // Set the navigation bar background to a shiny blue on Linux only Theme.navigationBar.backgroundColor = Theme.isLinux ? "blue" : "red" } }
[read-only] isOSX : bool |
Read-only property returning true
if the app is currently running on a macOS (Mac OS X) operating system.
You can use this property to change your app's style or logic depending on the underlying platform. The following example shows how to use a different navigation bar color for macOS devices:
import Felgo App { onInitTheme: { // Set the navigation bar background to a shiny blue on macOS only Theme.navigationBar.backgroundColor = Theme.isOSX ? "blue" : "red" } }
[read-only, since Felgo 2.6.2] isPortrait : bool |
Read-only property returning whether the app is currently in portrait mode. You can use this property to change your app's style based on the interface orientation. It refers to the App::portrait property of your application.
This property was introduced in Felgo 2.6.2.
[read-only, since Felgo 3.5.0] isWasm : bool |
Read-only property returning true
if the app is currently running as a WebAssembly build.
You can use this property to change your app's style or logic depending on the underlying platform. The following example shows how to use a different navigation bar color for WebAssembly builds:
import Felgo App { onInitTheme: { // Set the navigation bar background to a shiny blue on Linux only Theme.navigationBar.backgroundColor = Theme.isWasm ? "blue" : "red" } }
This property was introduced in Felgo 3.5.0.
[read-only] isWinPhone : bool |
Read-only property returning true
if the app is currently running on a Windows Phone device.
You can use this property to change your app's style or logic depending on the underlying platform. The following example shows how to use a different navigation bar color for Windows Phone devices:
import Felgo App { onInitTheme: { // Set the navigation bar background to a shiny blue on Windows Phone only Theme.navigationBar.backgroundColor = Theme.isWinPhone ? "blue" : "red" } }
[read-only] isWindows : bool |
Read-only property returning true
if the app is currently running on a Windows operating system.
You can use this property to change your app's style or logic depending on the underlying platform. The following example shows how to use a different navigation bar color for Windows devices:
import Felgo App { onInitTheme: { // Set the navigation bar background to a shiny blue on Windows only Theme.navigationBar.backgroundColor = Theme.isWindows ? "blue" : "red" } }
[since Felgo 2.6.2] listItem : ThemeSimpleRow |
You can override sub-properties of the listItem property to set the default appearance of SimpleRow list items. These items are the default component for ListView::delegate when using a ListPage.
The following properties can be overridden:
true
.
false
.true
.
true
to display a disclosure indicator for enabled items.This property was introduced in Felgo 2.6.2.
[since Felgo 2.6.2] listSection : ThemeSimpleSection |
You can override sub-properties of the listSection property to set the default appearance of ListView sections based on the SimpleSection type. This component is the default type for ListView::section.delegate when using a ListPage.
See the documentation of the ThemeSimpleSection type for an overview of available properties.
This property was introduced in Felgo 2.6.2.
[since Felgo 2.6.2] listSectionCompact : ThemeSimpleSection |
You can override sub-properties of the listSectionCompact property to set the default appearance of ListView sections based on the SimpleSection type, if SimpleSection::style.compactStyle is set to true
.
See the documentation of the ThemeSimpleSection type for an overview of available properties.
This property was introduced in Felgo 2.6.2.
[since Felgo 2.6.1] navigationAppDrawer : ThemeNavigationAppDrawer |
You can override sub-properties of the navigationAppDrawer property to set the global appearance of Navigation's AppDrawer items.
Following properties can be overridden:
This property was introduced in Felgo 2.6.1.
navigationBar : ThemeNavigationBar |
You can override sub-properties of the navigationBar property to set the global appearance of NavigationBar items (e.g. within a NavigationStack).
Following properties can be overridden:
navigationTabBar : ThemeNavigationTabControl |
You can override sub-properties of the navigation tabs property to set the global appearance of tabs created with Navigation.
Following properties can be overridden:
normalFont : QtObject |
Override this property to change the app-wide default font. It can either be set to a FontLoader object, or a simple QtObject with a name property, defining the used font name.
If not changed, the default font of an empty Text item is used.
[read-only] placeholderTextColor : color |
Read-only alias for colors.placeholderTextColor.
platform : string |
This property contains a string representing the current platform the app runs on.
It can also be changed to simulate running on a different platform, for testing purposes.
It has one of the following values:
[read-only, since Felgo 2.6.1] safeAreaTop : int |
Read-only property to retrieve the top inset of the display's safe area, in pixels.
On Android, if the status bar is system or hidden, 0
is returned. This is because then the actual window content starts at least below the safe area.
This property was introduced in Felgo 2.6.1.
[read-only, since Felgo 2.6.2] secondaryBackgroundColor : color |
Read-only alias for colors.secondaryBackgroundColor.
This property was introduced in Felgo 2.6.2.
[read-only] secondaryTextColor : color |
Read-only alias for colors.secondaryTextColor.
[read-only, since Felgo 2.6.2] selectedBackgroundColor : color |
Read-only alias for colors.selectedBackgroundColor.
This property was introduced in Felgo 2.6.2.
[read-only, since Felgo 2.6.1] statusBarHeight : int |
Read-only property to retrieve the status bar height of the device, in pixels. If the status bar is system or hidden, 0
is returned. This is because then the actual window content starts at least below the
status bar.
This property was introduced in Felgo 2.6.1.
[read-only] statusBarStyle : int |
Read-only alias for colors.statusBarStyle.
tabBar : ThemeTabControl |
You can override sub-properties of the tabBar property to set the global appearance of tabs created with TabControl or AppTabBar.
Following properties can be overridden:
[read-only] tintLightColor : color |
Read-only alias for colors.tintLightColor.
reset(softReset) |
Reset the default Theme. This discards any changes made to the Theme.