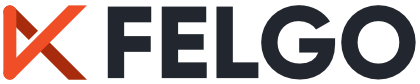
Input controller with keyboard support for moving entities. More...
Import Statement: | import Felgo 4.0 |
This component can be used for moving entities. It has 2 important properties: xAxis and yAxis. xAxis specifies the normalized output between -1 and +1 on the horizontal axis of a virtual TwoAxisController, the same for the yAxis axis.
You can inject input to a TwoAxisController from keyboard presses by mapping the inputs property to output actions. E.g. a Qt.Key_Left should match the "up" action, which sets the yAxis value to true. Use Keys.forwardTo: twoAxisController
to forward key presses of an item which has the focus to the controller. This item will usually be the
Scene.
You can also connect the xAxis and yAxis properties with other components like MoveToPointHelper, JoystickControllerHUD, Accelerometer. See the Advanced Usage section for more details.
GameWindow { Scene { focus: true Keys.forwardTo: controller EntityBase { id:player TwoAxisController { id: controller } } } }
If you have multiple players, the following is possible:
GameWindow { Scene { focus: true Keys.forwardTo: [controller1, controller2] EntityBase { id:player1 TwoAxisController { id: controller1 } } EntityBase { id:player2 TwoAxisController { id: controller2 inputActionsToKeyCode: { "up": Qt.Key_W, "down": Qt.Key_S, "left": Qt.Key_A, "right": Qt.Key_D, "fire": Qt.Key_Space } } } } }
This will use the Left, Right, Up, and Down keys to set the xAxis and yAxis value for player 1, and WASD for player2. When the Enter or Space key is pressed, the inputActionPressed signal will be emitted in TwoAxisController, which can be handled with inputActionPressed.
You can then connect the xAxis and yAxis properties to movement components like MovementAnimation or the force and torque properties of BoxCollider.
This is an example of connecting the TwoAxisController with a BoxCollider:
GameWindow { Scene { focus: true Keys.forwardTo: twoAxisController EntityBase { TwoAxisController { id: twoAxisController onInputActionPressed: { if(actionName == "fire") { // do something in here, e.g. create a new entity } } } BoxCollider { width: 60; height: 40 force: Qt.point(twoAxisController.yAxis*8000, 0) torque: twoAxisController.xAxis*2000 } } } }
The yAxis and xAxis property can also be set externally. This may be useful, when the same entity should be controlled by the AI. In that use-case, you can connect the xAxis and yAxis properties of TwoAxisController with MoveToPointHelper::outputXAxis and MoveToPointHelper::outputYAxis.
The TwoAxisController can also be connected to a JoystickControllerHUD. That has the advantage, that the game logic stays the same when playing on the desktop with a keyboard, as with a virtual thumbstick on mobile devices. This is an example of such a connection:
GameWindow { JoystickControllerHUD { width: 50; height: 50 source: "../assets/img/joystick_background.png" thumbSource: "../assets/img/joystick_thumb.png" // whenever the thumb position changes, update the twoAxisController onControllerXPositionChanged: twoAxisController.xAxis = controllerXPosition onControllerYPositionChanged: twoAxisController.yAxis = controllerYPosition } Scene { focus: true Keys.forwardTo: twoAxisController EntityBase { TwoAxisController { id: twoAxisController onInputActionPressed: { if(actionName == "fire") { // do something in here, e.g. create a new entity } } } BoxCollider { width: 60; height: 40 force: Qt.point(twoAxisController.yAxis*8000, 0) torque: twoAxisController.xAxis*2000 } } } }
inputActionsToKeyCode : variant |
This property contains the mapping between the pressed or released key code and the inputAction which should be emitted with inputActionPressed().
By default, it has the following value:
{ "up": Qt.Key_Up, "down": Qt.Key_Down, "left": Qt.Key_Left, "right": Qt.Key_Right, "fire": Qt.Key_Return }
Usually, you can just leave these default actions. If you want more input actions that can then be queried with your game logic, you can overwrite this variant map with something like the following:
{ "up": Qt.Key_Up, "down": Qt.Key_Down, "left": Qt.Key_Left, "right": Qt.Key_Right, "fire": Qt.Key_Return "changeToWeapon1": Qt.Key_1 "changeToWeapon2": Qt.Key_2 "reload": Qt.Key_R ... }
So this property allows you to set keyboard shortcuts for your game action. When you create a game for desktop and mobile, you will want to abstract your game actions in such a way, because the same game code should be called regardless if you press the fire button or press the fire key on the keyboard.
xAxis : real |
This property holds the x axis value of a 2-axis controller which can be connected to a rotation input property of supported components. When it is 0, that is equal to no required rotation, similar to no key being pressed on the keyboard. When it is +1, a clockwise rotation should be performed similar to a right key press. When it is -1, a counterclockwise rotation should be performed similar to a left key press.
When this property is set from keyboard presses, outputXAxis will not take values in between -1 and 0 or 0 and +1 because there is no analog value in between - either the button is pressed or it is not.
This property should be connected with a movement component like BoxCollider, CircleCollider or MovementAnimation. This example shows how to connect the outputXAxis property with the rotation property of a MovementAnimation:
GameWindow { Scene { focus: true Keys.forwardTo: twoAxisController EntityBase { TwoAxisController { id: twoAxisController } MovementAnimation { target: parent property: "rotation" // outputXAxis is +1 if target is to the right, -1 when to the left and 0 when aiming towards it velocity: 300*twoAxisController.xAxis // alternatively, also the acceleration could be set, depends on how you want the entity to behave // start rotating towards the target immediately, when xAxis is +1 or -1 running: true } } } }
yAxis : real |
This property holds the axis value of a 2-axis controller which can be connected to the velocity or force input property of supported components. When it is 0, that is equal to no required movement, similar to no key being pressed on the keyboard. When it is +1, a forward movement should be performed similar to an up key press. When it is -1, a backward movement should be performed similar to a down key press.
When this property is set from keyboard presses, outputYAxis will not take values in between -1 and 0 or 0 and +1 because there is no analog value in between - either the button is pressed or it is not.
This property should be connected with a movement component like BoxCollider, CircleCollider or MovementAnimation. This example shows how to connect the outputYAxis property with the force property of a BoxCollider:
GameWindow { Scene { focus: true Keys.forwardTo: twoAxisController EntityBase { TwoAxisController { id: twoAxisController } BoxCollider { width: 30; height:20 // move forwards and backwards, with a multiplication factor for the desired speed force: Qt.point(twoAxisController.outputYAxis*1000, 0) // rotate left and right, optional torque: twoAxisController.outputXAxis*300 } } } }
inputActionPressed(string actionName) |
This handler is called, when an input action is pressed. This happens either when a key with a matching key code from inputActionsToKeyCode occurs. Or when setInputActionPressedStatus() is called explicitly. The pressed action is available from the argument actionName.
Note: The corresponding handler is onInputActionPressed
.
inputActionReleased(string actionName) |
This handler is called, when an input action is released. This happens either when a key with a matching key code from inputActionsToKeyCode occurs. Or when setInputActionPressedStatus() is called explicitly. The pressed action is available from the argument actionName.
Note: The corresponding handler is onInputActionReleased
.
isPressed(actionName) |
This function returns whether the actionName is currently pressed. This will return true, in between a setInputActionStatus(action, true)
and a setInputActionStatus(action, false)
call. The
default pressed status for an action is false.
setInputActionPressedStatus(actionName, isPressed) |
Call this function to manually set the pressed status to isPressed of an input action with actionName.