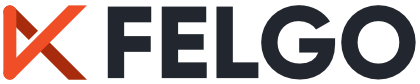
Qt 6 is a result of the conscious effort to make the framework more efficient and easy to use.
We try to maintain binary and source compatibility for all the public APIs in each release. But some changes were inevitable in an effort to make Qt a better framework.
In this topic we summarize those changes in Qt Concurrent, and provide guidance to handle them.
QtConcurrent::run() has been improved to work with a variable number of arguments, so the signatures are changed to:
// run template <typename T> QFuture<T> run(Function &&f, Args &&...args) // run with a QThreadPool argument template <typename T> QFuture<T> run(QThreadPool *pool, Function &&f, Args &&...args)
As a side effect, if f
is a pointer to a member function, the first argument of args
should be the object for which that member is defined (or a reference, or a pointer to it). So instead of
writing:
QImage image = ...; QFuture<void> future = QtConcurrent::run(&image, &QImage::invertPixels, QImage::InvertRgba);
You have to write:
QFuture<void> future = QtConcurrent::run(&QImage::invertPixels, &image, QImage::InvertRgba);
Another side effect is that QtConcurrent::run()
will not work with overloaded functions anymore. For example, the code below won't compile:
void foo(int arg); void foo(int arg1, int arg2); ... QFuture<void> future = QtConcurrent::run(foo, 42);
The easiest workaround is to call the overloaded function through lambda:
QFuture<void> future = QtConcurrent::run([] { foo(42); });
Or you can tell the compiler which overload to choose by using a static_cast
:
QFuture<void> future = QtConcurrent::run(static_cast<void(*)(int)>(foo), 42);
Or qOverload:
QFuture<void> future = QtConcurrent::run(qOverload<int>(foo), 42);
Other methods of QtConcurrent have no behavioral changes and do not introduce source compatibility breaks.
As part of the free Business evaluation, we offer a free welcome call for companies, to talk about your requirements, and how the Felgo SDK & Services can help you. Just sign up and schedule your call.
Sign up now to start your free Business evaluation: