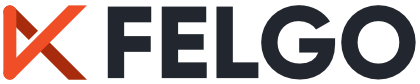
Provides meta-data for media files. More...
Header: | #include <QMediaMetaData> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS Multimedia) target_link_libraries(mytarget PRIVATE Qt6::Multimedia) |
qmake: | QT += multimedia |
enum | Key { Title, Author, Comment, Description, Genre, …, Resolution } |
void | clear() |
void | insert(QMediaMetaData::Key k, const QVariant &value) |
bool | isEmpty() const |
QList<QMediaMetaData::Key> | keys() const |
void | remove(QMediaMetaData::Key k) |
QString | stringValue(QMediaMetaData::Key key) const |
QVariant | value(QMediaMetaData::Key key) const |
QVariant & | operator[](QMediaMetaData::Key k) |
QString | metaDataKeyToString(QMediaMetaData::Key key) |
QHash<QMediaMetaData::Key, QVariant> | data |
QMetaType | keyType(QMediaMetaData::Key key) |
bool | operator!=(const QMediaMetaData &a, const QMediaMetaData &b) |
bool | operator==(const QMediaMetaData &a, const QMediaMetaData &b) |
Note: Not all identifiers are supported on all platforms.
Common attributes | ||
---|---|---|
Value | Description | Type |
Title | The title of the media. | QString |
Author | The authors of the media. | QStringList |
Comment | A user comment about the media. | QString |
Description | A description of the media. | QString |
Genre | The genre of the media. | QStringList |
Date | The date of the media. | QDateTime. |
Language | The language of media. | QLocale::Language |
Publisher | The publisher of the media. | QString |
Copyright | The media's copyright notice. | QString |
Url | A Url pointing to the origin of the media. | QUrl |
Media attributes | ||
MediaType | The type of the media (audio, video, etc). | QString |
FileFormat | The file format of the media. | QMediaFormat::FileFormat |
Duration | The duration in millseconds of the media. | qint64 |
Audio attributes | ||
AudioBitRate | The bit rate of the media's audio stream in bits per second. | int |
AudioCodec | The codec of the media's audio stream. | QMediaFormat::AudioCodec |
Video attributes | ||
VideoFrameRate | The frame rate of the media's video stream. | qreal |
VideoBitRate | The bit rate of the media's video stream in bits per second. | int |
VideoCodec | The codec of the media's video stream. | QMediaFormat::VideoCodec |
Music attributes | ||
AlbumTitle | The title of the album the media belongs to. | QString |
AlbumArtist | The principal artist of the album the media belongs to. | QString |
ContributingArtist | The artists contributing to the media. | QStringList |
TrackNumber | The track number of the media. | int |
Composer | The composer of the media. | QStringList |
LeadPerformer | The lead performer in the media. | QStringList |
ThumbnailImage | An embedded thumbnail image. | QImage |
CoverArtImage | An embedded cover art image. | QImage |
Image and video attributes | ||
Orientation | The rotation angle of an image or video. | int |
Resolution | The dimensions of an image or video. | QSize |
The following meta data keys can be used:
Constant | Value | Description |
---|---|---|
QMediaMetaData::Title |
0 |
Media title |
QMediaMetaData::Author |
1 |
Media author |
QMediaMetaData::Comment |
2 |
Comment |
QMediaMetaData::Description |
3 |
Brief desripttion |
QMediaMetaData::Genre |
4 |
Genre the media belongs to |
QMediaMetaData::Date |
5 |
Creation date |
QMediaMetaData::Language |
6 |
Media language |
QMediaMetaData::Publisher |
7 |
Media publisher info. |
QMediaMetaData::Copyright |
8 |
Media copyright info. |
QMediaMetaData::Url |
9 |
Publisher's website URL |
QMediaMetaData::Duration |
10 |
Media playback duration |
QMediaMetaData::MediaType |
11 |
Type of the media |
QMediaMetaData::FileFormat |
12 |
File format |
QMediaMetaData::AudioBitRate |
13 |
|
QMediaMetaData::AudioCodec |
14 |
|
QMediaMetaData::VideoBitRate |
15 |
|
QMediaMetaData::VideoCodec |
16 |
|
QMediaMetaData::VideoFrameRate |
17 |
|
QMediaMetaData::AlbumTitle |
18 |
Album's title |
QMediaMetaData::AlbumArtist |
19 |
Artist's info. |
QMediaMetaData::ContributingArtist |
20 |
|
QMediaMetaData::TrackNumber |
21 |
|
QMediaMetaData::Composer |
22 |
Media composer's info. |
QMediaMetaData::LeadPerformer |
23 |
|
QMediaMetaData::ThumbnailImage |
24 |
Media thumbnail image |
QMediaMetaData::CoverArtImage |
25 |
Media cover art |
QMediaMetaData::Orientation |
26 |
|
QMediaMetaData::Resolution |
27 |
[invokable]
void QMediaMetaData::clear()Removes all data from the meta data object.
Note: This function can be invoked via the meta-object system and from QML. See Q_INVOKABLE.
[invokable]
void QMediaMetaData::insert(QMediaMetaData::Key
k, const QVariant &value)Inserts a value into a Key: k.
Note: This function can be invoked via the meta-object system and from QML. See Q_INVOKABLE.
[invokable]
bool QMediaMetaData::isEmpty() constReturns true
if the meta data contains no items: otherwise returns false
.
Note: This function can be invoked via the meta-object system and from QML. See Q_INVOKABLE.
[static protected]
QMetaType QMediaMetaData::keyType(QMediaMetaData::Key key)Returns the meta type used to store data for Key key.
[invokable]
QList<QMediaMetaData::Key>
QMediaMetaData::keys() constReturns a QList of QMediaMetaData::Keys.
Note: This function can be invoked via the meta-object system and from QML. See Q_INVOKABLE.
[static invokable]
QString QMediaMetaData::metaDataKeyToString(QMediaMetaData::Key key)returns a string representation of key that can be used when presenting meta data to users.
Note: This function can be invoked via the meta-object system and from QML. See Q_INVOKABLE.
[invokable]
void QMediaMetaData::remove(QMediaMetaData::Key
k)Removes meta data from a Key: k.
Note: This function can be invoked via the meta-object system and from QML. See Q_INVOKABLE.
[invokable]
QString QMediaMetaData::stringValue(QMediaMetaData::Key key) constReturns the meta data for key key as a QString.
This is mainly meant to simplify presenting the meta data to a user.
Note: This function can be invoked via the meta-object system and from QML. See Q_INVOKABLE.
[invokable]
QVariant QMediaMetaData::value(QMediaMetaData::Key key) constReturns the meta data value for Key key, or a null QVariant if no meta data for the key is available.
Note: This function can be invoked via the meta-object system and from QML. See Q_INVOKABLE.
Returns data stored at the Key k.
QMediaMetaData rockBallad1; rockBalad[QMediaMetaData::Genre]="Rock"
This variable holds the meta data.
Note: this is a protected
member of its class.
Compares two meta data objects a and b, and returns false
if they are identical or true
if they differ.
Compares two meta data objects a and b, and returns true
if they are identical or false
if they differ.