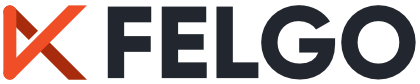
Positions its children in a column. More...
Import Statement: | import QtQuick |
Inherits: |
Column is a type that positions its child items along a single column. It can be used as a convenient way to vertically position a series of items without using anchors.
Below is a Column that contains three rectangles of various sizes:
Column { spacing: 2 Rectangle { color: "red"; width: 50; height: 50 } Rectangle { color: "green"; width: 20; height: 50 } Rectangle { color: "blue"; width: 50; height: 20 } }
The Column automatically positions these items in a vertical formation, like this:
If an item within a Column is not visible, or if it has a width or height of 0, the item will not be laid out and it will not be visible within the column. Also, since a Column automatically positions its children vertically, a child item within a Column should not set its y position or vertically anchor itself using the top, bottom, anchors.verticalCenter, fill or centerIn anchors. If you need to perform these actions, consider positioning the items without the use of a Column.
Note that items in a Column can use the Positioner attached property to access more information about its position within the Column.
For more information on using Column and other related positioner-types, see Item Positioners.
A Column animate items using specific transitions when items are added to or moved within a Column.
For example, the Column below sets the move property to a specific Transition:
Column { spacing: 2 Rectangle { color: "red"; width: 50; height: 50 } Rectangle { id: greenRect; color: "green"; width: 20; height: 50 } Rectangle { color: "blue"; width: 50; height: 20 } move: Transition { NumberAnimation { properties: "x,y"; duration: 1000 } } focus: true Keys.onSpacePressed: greenRect.visible = !greenRect.visible }
When the Space key is pressed, the visible value of the green Rectangle is toggled. As it appears and disappears, the blue Rectangle moves within the Column, and the move transition is automatically applied to the blue Rectangle:
See also Row, Grid, Flow, Positioner, ColumnLayout, and Qt Quick Examples - Positioners.
These properties hold the padding around the content.
This QML property was introduced in Qt 5.6.
add : Transition |
This property holds the transition to be run for items that are added to this positioner. For a positioner, this applies to:
The transition can use the ViewTransition property to access more details about the item that is being added. See the ViewTransition documentation for more details and examples on using these transitions.
Note: This transition is not applied to the items that are already part of the positioner at the time of its creation. In this case, the populate transition is applied instead.
See also populate, ViewTransition, and Qt Quick Examples - Positioners.
move : Transition |
This property holds the transition to run for items that have moved within the positioner. For a positioner, this applies to:
The transition can use the ViewTransition property to access more details about the item that is being moved. Note, however, that for this move transition, the ViewTransition.targetIndexes and ViewTransition.targetItems lists are only set when this transition is triggered by the addition of other items in the positioner; in other cases, these lists will be empty. See the ViewTransition documentation for more details and examples on using these transitions.
See also add, populate, ViewTransition, and Qt Quick Examples - Positioners.
populate : Transition |
This property holds the transition to be run for items that are part of this positioner at the time of its creation. The transition is run when the positioner is first created.
The transition can use the ViewTransition property to access more details about the item that is being added. See the ViewTransition documentation for more details and examples on using these transitions.
See also add, ViewTransition, and Qt Quick Examples - Positioners.
spacing : real |
The spacing is the amount in pixels left empty between adjacent items. The default spacing is 0.
See also Grid::spacing.
|
This signal is emitted when positioning has been completed.
Note: The corresponding handler is onPositioningComplete
.
This signal was introduced in Qt 5.9.
|
Column typically positions its children once per frame. This means that inside script blocks it is possible for the underlying children to have changed, but the Column to have not yet been updated accordingly.
This method forces the Column to immediately respond to any outstanding changes in its children.
Note: methods in general should only be called after the Component has completed.
This method was introduced in Qt 5.9.