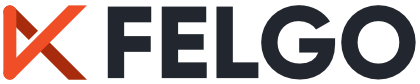
Handler for mouse and tablet hover. More...
Import Statement: | import QtQuick |
Inherits: |
HoverHandler detects a hovering mouse or tablet stylus cursor.
A binding to the hovered property is the easiest way to react when the cursor enters or leaves the parent Item. The point property provides more detail, including the cursor position. The acceptedDevices, acceptedPointerTypes, and acceptedModifiers properties can be used to narrow the behavior to detect hovering of specific kinds of devices or while holding a modifier key.
The cursorShape property allows changing the cursor whenever hovered changes to
true
.
See also MouseArea and PointHandler.
acceptedDevices : flags |
The types of pointing devices that can activate the pointer handler.
By default, this property is set to PointerDevice.AllDevices. If you set it to an OR combination of device types, it will ignore pointer events from the non-matching devices.
For example, an item could be made to respond to mouse hover in one way, and stylus hover in another way, with two handlers:
import QtQuick Rectangle { width: 150; height: 50; radius: 3 color: mouse.hovered ? "goldenrod" : stylus.hovered ? "tomato" : "wheat" HoverHandler { id: stylus acceptedDevices: PointerDevice.Stylus cursorShape: Qt.CrossCursor } HoverHandler { id: mouse acceptedDevices: PointerDevice.Mouse cursorShape: Qt.PointingHandCursor } }
The available device types are as follows:
Constant | Description |
---|---|
PointerDevice.Mouse |
A mouse. |
PointerDevice.TouchScreen |
A touchscreen. |
PointerDevice.TouchPad |
A touchpad or trackpad. |
PointerDevice.Stylus |
A stylus on a graphics tablet. |
PointerDevice.Airbrush |
An airbrush on a graphics tablet. |
PointerDevice.Puck |
A digitizer with crosshairs, on a graphics tablet. |
PointerDevice.AllDevices |
Any type of pointing device. |
See also QInputDevice::DeviceType.
acceptedModifiers : flags |
If this property is set, a hover event is handled only if the given keyboard modifiers are pressed. The event is ignored without the modifiers.
This property is set to Qt.KeyboardModifierMask
by default, resulting in handling hover events regardless of any modifier keys.
For example, an Item could have two handlers of the same type, one of which is enabled only if the required keyboard modifiers are pressed:
import QtQuick Rectangle { width: 150; height: 50; radius: 3 color: control.hovered ? "goldenrod" : shift.hovered ? "wheat" : "beige" HoverHandler { id: control acceptedModifiers: Qt.ControlModifier cursorShape: Qt.PointingHandCursor } HoverHandler { id: shift acceptedModifiers: Qt.ShiftModifier cursorShape: Qt.CrossCursor } }
The available modifiers are as follows:
Constant | Description |
---|---|
Qt.NoModifier |
No modifier key is allowed. |
Qt.ShiftModifier |
A Shift key on the keyboard must be pressed. |
Qt.ControlModifier |
A Ctrl key on the keyboard must be pressed. |
Qt.AltModifier |
An Alt key on the keyboard must be pressed. |
Qt.MetaModifier |
A Meta key on the keyboard must be pressed. |
Qt.KeypadModifier |
A keypad button must be pressed. |
Qt.GroupSwitchModifier |
A Mode_switch key on the keyboard must be pressed. X11 only (unless activated on Windows by a command line argument). |
Qt.KeyboardModifierMask |
The handler ignores modifier keys. |
See also Qt::KeyboardModifier.
acceptedPointerTypes : flags |
The types of pointing instruments (generic, stylus, eraser, and so on) that can activate the pointer handler.
By default, this property is set to PointerDevice.AllPointerTypes. If you set it to an OR combination of device types, it will ignore events from non-matching events.
For example, you could provide feedback by changing the cursor depending on whether a stylus or eraser is hovering over a graphics tablet:
import QtQuick Rectangle { id: rect width: 150; height: 150 HoverHandler { id: stylus acceptedPointerTypes: PointerDevice.Pen cursorShape: Qt.CrossCursor } HoverHandler { id: eraser acceptedPointerTypes: PointerDevice.Eraser cursorShape: Qt.BlankCursor target: Image { parent: rect source: "images/cursor-eraser.png" visible: eraser.hovered x: eraser.point.position.x y: eraser.point.position.y - 32 } } }
The available pointer types are as follows:
Constant | Description |
---|---|
PointerDevice.Generic |
A mouse or a device that emulates a mouse. |
PointerDevice.Finger |
A finger on a touchscreen (hover detection is unlikely). |
PointerDevice.Pen |
A stylus on a graphics tablet. |
PointerDevice.Eraser |
An eraser on a graphics tablet. |
PointerDevice.Cursor |
A digitizer with crosshairs, on a graphics tablet. |
PointerDevice.AllPointerTypes |
Any type of pointing device. |
See also QPointingDevice::PointerType.
[read-only] active : bool |
This holds true whenever this Input Handler has taken sole responsibility for handing one or more EventPoints, by successfully taking an exclusive grab of those points. This means that it is keeping its properties up-to-date according to the movements of those Event Points and actively manipulating its target (if any).
[since 6.3] blocking : bool |
Whether this handler prevents other items or handlers behind it from being hovered at the same time. This property is false
by default.
This property was introduced in Qt 6.3.
[since 5.15] cursorShape : Qt::CursorShape |
This property holds the cursor shape that will appear whenever hovered is true
and no other handler is overriding it.
The available cursor shapes are:
The default value of this property is not set, which allows any active handler on the same parent item to determine the cursor shape. This property can be reset to the initial condition by setting it to
undefined
.
If any handler with defined cursorShape
is active, that cursor will appear. Else if the HoverHandler
has a defined cursorShape
, that cursor will appear. Otherwise, the cursor of parent item will appear.
Note: When this property has not been set, or has been set to undefined
, if you read the value it will return Qt.ArrowCursor
.
This property was introduced in Qt 5.15.
See also Qt::CursorShape and QQuickItem::cursor().
[since 5.15] dragThreshold : int |
The distance in pixels that the user must drag an event point in order to have it treated as a drag gesture.
The default value depends on the platform and screen resolution. It can be reset back to the default value by setting it to undefined. The behavior when a drag gesture begins varies in different handlers.
This property was introduced in Qt 5.15.
enabled : bool |
If a PointerHandler is disabled, it will reject all events and no signals will be emitted.
grabPermissions : flags |
This property specifies the permissions when this handler's logic decides to take over the exclusive grab, or when it is asked to approve grab takeover or cancellation by another handler.
Constant | Description |
---|---|
PointerHandler.TakeOverForbidden |
This handler neither takes from nor gives grab permission to any type of Item or Handler. |
PointerHandler.CanTakeOverFromHandlersOfSameType |
This handler can take the exclusive grab from another handler of the same class. |
PointerHandler.CanTakeOverFromHandlersOfDifferentType |
This handler can take the exclusive grab from any kind of handler. |
PointerHandler.CanTakeOverFromItems |
This handler can take the exclusive grab from any type of Item. |
PointerHandler.CanTakeOverFromAnything |
This handler can take the exclusive grab from any type of Item or Handler. |
PointerHandler.ApprovesTakeOverByHandlersOfSameType |
This handler gives permission for another handler of the same class to take the grab. |
PointerHandler.ApprovesTakeOverByHandlersOfDifferentType |
This handler gives permission for any kind of handler to take the grab. |
PointerHandler.ApprovesTakeOverByItems |
This handler gives permission for any kind of Item to take the grab. |
PointerHandler.ApprovesCancellation |
This handler will allow its grab to be set to null. |
PointerHandler.ApprovesTakeOverByAnything |
This handler gives permission for any any type of Item or Handler to take the grab. |
The default is PointerHandler.CanTakeOverFromItems | PointerHandler.CanTakeOverFromHandlersOfDifferentType | PointerHandler.ApprovesTakeOverByAnything
which allows most takeover scenarios but avoids e.g. two
PinchHandlers fighting over the same touchpoints.
[read-only] hovered : bool |
Holds true whenever any pointing device cursor (mouse or tablet) is within the bounds of the parent
Item, extended by the margin, if any.
margin : real |
The margin beyond the bounds of the parent item within which an event point can activate this handler. For example, on a PinchHandler where the target is also the parent
, it's useful to set this to a distance at least half the width
of a typical user's finger, so that if the parent
has been scaled down to a very small size, the pinch gesture is still possible. Or, if a TapHandler-based button is
placed near the screen edge, it can be used to comply with Fitts's Law: react to mouse clicks at the screen edge even though the button is visually spaced away from the edge by a few pixels.
The default value is 0.
parent : Item |
The Item which is the scope of the handler; the Item in which it was declared. The handler will handle events on behalf of this Item, which means a pointer event is relevant if at least one of its event points occurs within the Item's interior. Initially target() is the same, but it can be reassigned.
Note: When a handler is declared in a QtQuick3D.Model object, the parent is not an Item, therefore this property is null
.
See also target and QObject::parent().
[read-only] point : HandlerPoint |
The event point currently being handled. When no point is currently being handled, this object is reset to default values (all coordinates are 0).
target : Item |
The Item which this handler will manipulate.
By default, it is the same as the parent, the Item within which the handler is declared. However, it can sometimes be useful to set the target to a different
Item, in order to handle events within one item but manipulate another; or to null
, to disable the default behavior and do something else instead.
canceled(EventPoint point) |
If this handler has already grabbed the given point, this signal is emitted when the grab is stolen by a different Pointer Handler or Item.
Note: The corresponding handler is onCanceled
.
grabChanged(GrabTransition transition, EventPoint point) |
This signal is emitted when the grab has changed in some way which is relevant to this handler.
The transition (verb) tells what happened. The point (object) is the point that was grabbed or ungrabbed.
Note: The corresponding handler is onGrabChanged
.