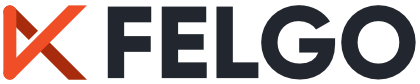
A PDF viewer widget. More...
Header: | #include <QPdfView> |
Inherits: | QAbstractScrollArea |
|
|
QPdfView(QWidget *parent) | |
virtual | ~QPdfView() |
QPdfDocument * | document() const |
QMargins | documentMargins() const |
QPdfView::PageMode | pageMode() const |
QPdfPageNavigator * | pageNavigator() const |
int | pageSpacing() const |
void | setDocument(QPdfDocument *document) |
void | setDocumentMargins(QMargins margins) |
void | setPageSpacing(int spacing) |
qreal | zoomFactor() const |
QPdfView::ZoomMode | zoomMode() const |
void | setPageMode(QPdfView::PageMode mode) |
void | setZoomFactor(qreal factor) |
void | setZoomMode(QPdfView::ZoomMode mode) |
void | documentChanged(QPdfDocument *document) |
void | documentMarginsChanged(QMargins documentMargins) |
void | pageModeChanged(QPdfView::PageMode pageMode) |
void | pageSpacingChanged(int pageSpacing) |
void | zoomFactorChanged(qreal zoomFactor) |
void | zoomModeChanged(QPdfView::ZoomMode zoomMode) |
QPdfView is a PDF viewer widget that offers a user experience similar to many common PDF viewer applications, with two modes. In the MultiPage
mode, it supports
flicking through the pages in the entire document, with narrow gaps between the page images. In the SinglePage
mode, it shows one page at a time.
This enum describes the overall behavior of the PDF viewer:
Constant | Value | Description |
---|---|---|
QPdfView::PageMode::SinglePage |
0 |
Show one page at a time. |
QPdfView::PageMode::MultiPage |
1 |
Allow scrolling through all pages in the document. |
This enum describes the magnification behavior of the PDF viewer:
Constant | Value | Description |
---|---|---|
QPdfView::ZoomMode::Custom |
0 |
Use zoomFactor only. |
QPdfView::ZoomMode::FitToWidth |
1 |
Automatically choose a zoom factor so that the width of the page fits in the view. |
QPdfView::ZoomMode::FitInView |
2 |
Automatically choose a zoom factor so that the entire page fits in the view. |
This property holds the document to be viewed.
Access functions:
QPdfDocument * | document() const |
void | setDocument(QPdfDocument *document) |
Notifier signal:
void | documentChanged(QPdfDocument *document) |
This property holds the margins around the page view.
Access functions:
QMargins | documentMargins() const |
void | setDocumentMargins(QMargins margins) |
Notifier signal:
void | documentMarginsChanged(QMargins documentMargins) |
This property holds whether to show one page at a time, or all pages in the document. The default is SinglePage
.
Access functions:
QPdfView::PageMode | pageMode() const |
void | setPageMode(QPdfView::PageMode mode) |
Notifier signal:
void | pageModeChanged(QPdfView::PageMode pageMode) |
This property holds the size of the padding between pages in the MultiPage mode.
Access functions:
int | pageSpacing() const |
void | setPageSpacing(int spacing) |
Notifier signal:
void | pageSpacingChanged(int pageSpacing) |
This property holds the ratio of pixels to points. The default is 1
, meaning one point (1/72 of an inch) equals 1 logical pixel.
Access functions:
qreal | zoomFactor() const |
void | setZoomFactor(qreal factor) |
Notifier signal:
void | zoomFactorChanged(qreal zoomFactor) |
This property indicates whether to use a custom size for the page(s), or zoom them to fit to the view. The default is CustomZoom
.
Access functions:
QPdfView::ZoomMode | zoomMode() const |
void | setZoomMode(QPdfView::ZoomMode mode) |
Notifier signal:
void | zoomModeChanged(QPdfView::ZoomMode zoomMode) |
Constructs a PDF viewer with parent widget parent.
[virtual]
QPdfView::~QPdfView()Destroys the PDF viewer.
This accessor returns the navigation stack that will handle back/forward navigation.