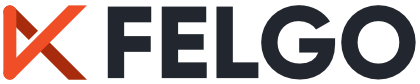
A sound object in 3D space. More...
Header: | #include <QSpatialSound> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS SpatialAudio) target_link_libraries(mytarget PRIVATE Qt6::SpatialAudio) |
qmake: | QT += spatialaudio |
Inherits: | QObject |
enum class | DistanceModel { Logarithmic, Linear, ManualAttenuation } |
enum | Loops { Infinite, Once } |
|
|
QSpatialSound(QAudioEngine *engine) | |
virtual | ~QSpatialSound() |
bool | autoPlay() const |
float | directivity() const |
float | directivityOrder() const |
float | distanceCutoff() const |
QSpatialSound::DistanceModel | distanceModel() const |
QAudioEngine * | engine() const |
int | loops() const |
float | manualAttenuation() const |
float | nearFieldGain() const |
float | occlusionIntensity() const |
QVector3D | position() const |
QQuaternion | rotation() const |
void | setAutoPlay(bool autoPlay) |
void | setDirectivity(float alpha) |
void | setDirectivityOrder(float alpha) |
void | setDistanceCutoff(float cutoff) |
void | setDistanceModel(QSpatialSound::DistanceModel model) |
void | setLoops(int loops) |
void | setManualAttenuation(float attenuation) |
void | setNearFieldGain(float gain) |
void | setOcclusionIntensity(float occlusion) |
void | setPosition(QVector3D pos) |
void | setRotation(const QQuaternion &q) |
void | setSize(float size) |
void | setSource(const QUrl &url) |
void | setVolume(float volume) |
float | size() const |
QUrl | source() const |
float | volume() const |
void | autoPlayChanged() |
void | directivityChanged() |
void | directivityOrderChanged() |
void | distanceCutoffChanged() |
void | distanceModelChanged() |
void | loopsChanged() |
void | manualAttenuationChanged() |
void | nearFieldGainChanged() |
void | occlusionIntensityChanged() |
void | positionChanged() |
void | rotationChanged() |
void | sizeChanged() |
void | sourceChanged() |
void | volumeChanged() |
QSpatialSound represents an audible object in 3D space. You can define its position and orientation in space, set the sound it is playing and define a volume for the object.
The object can have different attenuation behavior, emit sound mainly in one direction or spherically, and behave as if occluded by some other object.
Defines how the volume of the sound scales with distance to the listener.
Constant | Value | Description |
---|---|---|
QSpatialSound::DistanceModel::Logarithmic |
0 |
Volume decreases logarithmically with distance. |
QSpatialSound::DistanceModel::Linear |
1 |
Volume decreases linearly with distance. |
QSpatialSound::DistanceModel::ManualAttenuation |
2 |
Attenuation is defined manually using the manualAttenuation property. |
Lets you control the sound playback loop using the following values:
Constant | Value | Description |
---|---|---|
QSpatialSound::Infinite |
-1 |
Playback infinitely |
QSpatialSound::Once |
1 |
Playback once |
Determines whether the sound should automatically start playing when a source gets specified.
The default value is true
.
Access functions:
bool | autoPlay() const |
void | setAutoPlay(bool autoPlay) |
Notifier signal:
void | autoPlayChanged() |
Defines the directivity of the sound source. A value of 0 implies that the sound is emitted equally in all directions, while a value of 1 implies that the source mainly emits sound in the forward direction.
Valid values are between 0 and 1, the default is 0.
Access functions:
float | directivity() const |
void | setDirectivity(float alpha) |
Notifier signal:
void | directivityChanged() |
Defines the order of the directivity of the sound source. A higher order implies a sharper localization of the sound cone.
The minimum value and default for this property is 1.
Access functions:
float | directivityOrder() const |
void | setDirectivityOrder(float alpha) |
Notifier signal:
void | directivityOrderChanged() |
Defines a distance beyond which sound coming from the source will cutoff. If the listener is further away from the sound object than the cutoff distance it won't be audible anymore.
Access functions:
float | distanceCutoff() const |
void | setDistanceCutoff(float cutoff) |
Notifier signal:
void | distanceCutoffChanged() |
Defines distance model for this sound source. The volume starts scaling down from size to distanceCutoff. The volume is constant for distances smaller than size and zero for distances larger than the cutoff distance.
Access functions:
QSpatialSound::DistanceModel | distanceModel() const |
void | setDistanceModel(QSpatialSound::DistanceModel model) |
Notifier signal:
void | distanceModelChanged() |
See also QSpatialSound::DistanceModel.
Determines how many times the sound is played before the player stops. Set to QSpatialSound::Infinite to play the current sound in a loop forever.
The default value is 1
.
Access functions:
int | loops() const |
void | setLoops(int loops) |
Notifier signal:
void | loopsChanged() |
Defines a manual attenuation factor if distanceModel is set to QSpatialSound::DistanceModel::ManualAttenuation.
Access functions:
float | manualAttenuation() const |
void | setManualAttenuation(float attenuation) |
Notifier signal:
void | manualAttenuationChanged() |
Defines the near field gain for the sound source. Valid values are between 0 and 1. A near field gain of 1 will raise the volume of the sound signal by approx 20 dB for distances very close to the listener.
Access functions:
float | nearFieldGain() const |
void | setNearFieldGain(float gain) |
Notifier signal:
void | nearFieldGainChanged() |
Defines how much the object is occluded. 0 implies the object is not occluded at all, 1 implies the sound source is fully occluded by another object.
A fully occluded object will still be audible, but especially higher frequencies will be dampened. In addition, the object will still participate in generating reverb and reflections in the room.
Values larger than 1 are possible to further dampen the direct sound coming from the source.
The default is 0.
Access functions:
float | occlusionIntensity() const |
void | setOcclusionIntensity(float occlusion) |
Notifier signal:
void | occlusionIntensityChanged() |
Defines the position of the sound source in 3D space. Units are in centimeters by default.
Access functions:
QVector3D | position() const |
void | setPosition(QVector3D pos) |
Notifier signal:
void | positionChanged() |
See also QAudioEngine::distanceScale.
Defines the orientation of the sound source in 3D space.
Access functions:
QQuaternion | rotation() const |
void | setRotation(const QQuaternion &q) |
Notifier signal:
void | rotationChanged() |
Defines the size of the sound source. If the listener is closer to the sound object than the size, volume will stay constant. The size is also used to for occlusion calculations, where large sources can be partially occluded by a wall.
Access functions:
float | size() const |
void | setSize(float size) |
Notifier signal:
void | sizeChanged() |
The source file for the sound to be played.
Access functions:
QUrl | source() const |
void | setSource(const QUrl &url) |
Notifier signal:
void | sourceChanged() |
Defines the volume of the sound.
Values between 0 and 1 will attenuate the sound, while values above 1 provide an additional gain boost.
Access functions:
float | volume() const |
void | setVolume(float volume) |
Notifier signal:
void | volumeChanged() |
Creates a spatial sound source for engine. The object can be placed in 3D space and will be louder the closer to the listener it is.
[slot]
void QSpatialSound::pause()Pauses sound playback. Calling play() will continue playback.
[slot]
void QSpatialSound::play()Starts playing back the sound. Does nothing if the sound is already playing.
[slot]
void QSpatialSound::stop()Stops sound playback and resets the current position and current loop count to 0. Calling play() will start playback at the beginning of the sound file.
[virtual]
QSpatialSound::~QSpatialSound()Destroys the sound source.
Returns the engine associated with this listener.