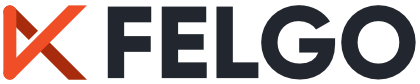
Setup project-wide defaults to a standard arrangement.
The command is defined in the Core
component of the Qt6
package, which can be loaded like so:
find_package(Qt6 REQUIRED COMPONENTS Core)
This command was introduced in Qt 6.3.
qt_standard_project_setup()
If versionless commands are disabled, use qt6_standard_project_setup()
instead. It supports the same set of arguments as this
command.
This command simplifies the task of setting up a typical Qt application. It would usually be called immediately after the first find_package(Qt6)
call, normally in the top level CMakeLists.txt
file
and before any targets have been defined. It does the following things:
CMAKE_AUTOMOC
and CMAKE_AUTOUIC
are set to true if they are not already defined. This enables all Qt-related autogen features by default for subsequently created
targets in the current directory scope and below.CMAKE_INSTALL_BINDIR
,
CMAKE_INSTALL_LIBDIR
, and so on.
CMAKE_RUNTIME_OUTPUT_DIRECTORY
variable is not already set, it will be set to ${CMAKE_CURRENT_BINARY_DIR}
.CMAKE_INSTALL_RPATH
will be augmented as described below.On platforms that support RPATH
(other than Apple platforms), two values are appended to the CMAKE_INSTALL_RPATH
variable by this command. $ORIGIN
is appended so that libraries will
find other libraries they depend on in the same directory as themselves. $ORIGIN/<reldir>
is also appended, where <reldir>
is the relative path from CMAKE_INSTALL_BINDIR
to
CMAKE_INSTALL_LIBDIR
. This allows executables installed to CMAKE_INSTALL_BINDIR
to find any libraries they may depend on installed to CMAKE_INSTALL_LIBDIR
. Any duplicates in
CMAKE_INSTALL_RPATH
are removed. In practice, these two values ensure that executables and libraries will find their link-time dependencies, assuming projects install them to the default locations the install(TARGETS) command uses when no destination is explicitly provided.
The qt_standard_project_setup()
command can effectively be disabled by setting the QT_NO_STANDARD_PROJECT_SETUP variable to true.
cmake_minimum_required(VERSION 3.16...3.22) project(MyThings) find_package(Qt6 REQUIRED COMPONENTS Core) qt_standard_project_setup() qt_add_executable(MyApp main.cpp) set_target_properties(MyApp PROPERTIES WIN32_EXECUTABLE TRUE MACOSX_BUNDLE TRUE ) # App bundles on macOS have an .app suffix if(APPLE) set(executable_path "$<TARGET_FILE_NAME:MyApp>.app") else() set(executable_path "\${QT_DEPLOY_BIN_DIR}/$<TARGET_FILE_NAME:MyApp>") endif() # Helper app, not necessarily built as part of this project. qt_add_executable(HelperApp helper.cpp) set(helper_app_path "\${QT_DEPLOY_BIN_DIR}/$<TARGET_FILE_NAME:HelperApp>") # The following script must only be executed at install time set(deploy_script "${CMAKE_CURRENT_BINARY_DIR}/deploy_MyApp.cmake") file(GENERATE OUTPUT ${deploy_script} CONTENT " # Including the file pointed to by QT_DEPLOY_SUPPORT ensures the generated # deployment script has access to qt_deploy_runtime_dependencies() include(\"${QT_DEPLOY_SUPPORT}\") qt_deploy_runtime_dependencies( EXECUTABLE \"${executable_path}\" ADDITIONAL_EXECUTABLES \"${helper_app_path}\" GENERATE_QT_CONF VERBOSE )") # Omitting RUNTIME DESTINATION will install a non-bundle target to CMAKE_INSTALL_BINDIR, # which coincides with the default value of QT_DEPLOY_BIN_DIR used above, './bin'. # Installing macOS bundles always requires an explicit BUNDLE DESTINATION option. install(TARGETS MyApp HelperApp # Install to CMAKE_INSTALL_PREFIX/bin/MyApp.exe # and ./binHelperApp.exe BUNDLE DESTINATION . # Install to CMAKE_INSTALL_PREFIX/MyApp.app/Contents/MacOS/MyApp ) install(SCRIPT ${deploy_script}) # Add its runtime dependencies
See also qt_generate_deploy_app_script().
As part of the free Business evaluation, we offer a free welcome call for companies, to talk about your requirements, and how the Felgo SDK & Services can help you. Just sign up and schedule your call.
Sign up now to start your free Business evaluation: