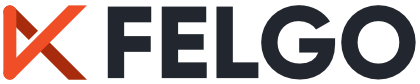
The QTextureData class stores texture information such as the target, height, width, depth, layers, wrap, and if mipmaps are enabled. More...
Header: | #include <Qt3DRender/QTextureData> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS 3drender) target_link_libraries(mytarget PRIVATE Qt6::3drender) |
qmake: | QT += 3drender |
Since: | Qt 5.7 |
QTextureData() | |
void | addImageData(const Qt3DRender::QTextureImageDataPtr &imageData) |
QAbstractTexture::ComparisonFunction | comparisonFunction() const |
QAbstractTexture::ComparisonMode | comparisonMode() const |
int | depth() const |
QAbstractTexture::TextureFormat | format() const |
int | height() const |
QList<Qt3DRender::QTextureImageDataPtr> | imageData() const |
bool | isAutoMipMapGenerationEnabled() const |
int | layers() const |
QAbstractTexture::Filter | magnificationFilter() const |
float | maximumAnisotropy() const |
QAbstractTexture::Filter | minificationFilter() const |
void | setAutoMipMapGenerationEnabled(bool autoMipMap) |
void | setComparisonFunction(QAbstractTexture::ComparisonFunction comparisonFunction) |
void | setComparisonMode(QAbstractTexture::ComparisonMode comparisonMode) |
void | setDepth(int depth) |
void | setFormat(QAbstractTexture::TextureFormat format) |
void | setHeight(int height) |
void | setLayers(int layers) |
void | setMagnificationFilter(QAbstractTexture::Filter filter) |
void | setMaximumAnisotropy(float maximumAnisotropy) |
void | setMinificationFilter(QAbstractTexture::Filter filter) |
void | setTarget(QAbstractTexture::Target target) |
void | setWidth(int width) |
void | setWrapModeX(QTextureWrapMode::WrapMode wrapModeX) |
void | setWrapModeY(QTextureWrapMode::WrapMode wrapModeY) |
void | setWrapModeZ(QTextureWrapMode::WrapMode wrapModeZ) |
QAbstractTexture::Target | target() const |
int | width() const |
QTextureWrapMode::WrapMode | wrapModeX() const |
QTextureWrapMode::WrapMode | wrapModeY() const |
QTextureWrapMode::WrapMode | wrapModeZ() const |
Creates a new QTextureData instance.
Adds an extra image layer to the texture using imageData.
Note: The texture image should be loaded with the size specified on the texture. However, if no size is specified, the size of the first texture image file is used as default.
Returns the current comparison function.
See also setComparisonFunction().
Returns the current comparison mode.
See also setComparisonMode().
Returns the texture depth.
See also setDepth().
Returns the texture format
See also setFormat().
Returns the texture height.
See also setHeight().
Returns the data of the images used by this texture.
Returns whether the texture has auto mipmap generation enabled.
Returns the texture layers.
See also setLayers().
Returns the current magnification filter.
See also setMagnificationFilter().
Returns the current maximum anisotropy.
See also setMaximumAnisotropy().
Returns the current minification filter.
See also setMinificationFilter().
Sets whether the texture has automatic mipmap generation enabled, to autoMipMap.
See also isAutoMipMapGenerationEnabled().
Sets the comparison function to comparisonFunction.
See also comparisonFunction().
Sets the comparison mode to comparisonMode.
See also comparisonMode().
Sets the texture depth to depth
See also depth().
Sets the texture format to format.
See also format().
Sets the target height to height.
See also height().
Sets the texture layers to layers.
See also layers().
Sets the magnification filter to filter.
See also magnificationFilter().
Sets the maximum anisotropy to maximumAnisotropy.
See also maximumAnisotropy().
Sets the minification filter to filter.
See also minificationFilter().
Sets the target texture to target.
See also target().
Sets the texture width to width.
See also width().
Sets the wrap mode X to wrapModeX.
See also wrapModeX().
Sets the wrap mode Y to wrapModeY.
See also wrapModeY().
Sets the wrap mode Z to wrapModeZ.
See also wrapModeZ().
Returns the texture data target.
See also setTarget().
Returns the texture width.
See also setWidth().
Returns the current wrap mode X.
See also setWrapModeX().
Returns the current wrap mode Y.
See also setWrapModeY().
Returns the current wrap mode Z.
See also setWrapModeZ().