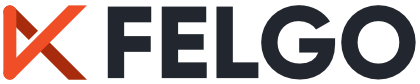
The Cbordump example demonstrates how to parse files in CBOR-format.
The Cbordump example reads from files or stdin content in CBOR-format and dumps the decoded content to stdout. The cbordump utility can output in CBOR diagnostic notation (which is similar to JSON), or it can have a verbose output where each byte input is displayed with the encoding beside it. This example shows how to use the QCborStreamReader class directly to parse CBOR content.
The Cbordumper class contains a QCborStreamReader object that is initialized using the QFile object argument passed to the CborDumper constructor. Based on the arguments the dump function calls either dumpOne() or dumpOneDetailed() to dump the contents to stdout,
struct CborDumper { enum DumpOption { ShowCompact = 0x01, ShowWidthIndicators = 0x02, ShowAnnotated = 0x04 }; Q_DECLARE_FLAGS(DumpOptions, DumpOption) CborDumper(QFile *f, DumpOptions opts_); QCborError dump(); private: void dumpOne(int nestingLevel); void dumpOneDetailed(int nestingLevel); void printByteArray(const QByteArray &ba); void printWidthIndicator(quint64 value, char space = '\0'); void printStringWidthIndicator(quint64 value); QCborStreamReader reader; QByteArray data; QStack<quint8> byteArrayEncoding; qint64 offset = 0; DumpOptions opts; };
The type() function of the QCborStreamReader is used in a switch statement to print out for each type. If the type is an array or map, the content is iterated upon, and for each entry the dumpOne() function is called recursively with a higher indentation argument. If the type is a tag, it is printed out and dumpOne() is called once without increasing the indentation argument.
This function dumps out both the incoming bytes and the decoded contents on the same line. It uses lambda functions to print out the bytes and decoded content, but otherwise has a similar structure as dumpOne().
The tagDescriptions table, describing the CBOR-tags available, is automatically generated from an XML-file available from the iana.org website.
See also QCborStreamReader and CBOR Support in Qt.
As part of the free Business evaluation, we offer a free welcome call for companies, to talk about your requirements, and how the Felgo SDK & Services can help you. Just sign up and schedule your call.
Sign up now to start your free Business evaluation: