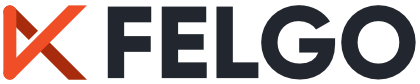
The QVoice class represents a particular voice. More...
Header: | #include <QVoice> |
CMake: | find_package(Qt6 REQUIRED COMPONENTS TextToSpeech) target_link_libraries(mytarget PRIVATE Qt6::TextToSpeech) |
qmake: | QT += texttospeech |
QVoice(QVoice &&other) | |
QVoice(const QVoice &other) | |
QVoice() | |
QVoice & | operator=(QVoice &&other) |
QVoice & | operator=(const QVoice &other) |
~QVoice() | |
QVoice::Age | age() const |
QVoice::Gender | gender() const |
QLocale | locale() const |
QString | name() const |
void | swap(QVoice &other) |
QString | ageName(QVoice::Age age) |
QString | genderName(QVoice::Gender gender) |
bool | operator!=(const QVoice &lhs, const QVoice &rhs) |
QDataStream & | operator<<(QDataStream &stream, const QVoice &voice) |
QDebug | operator<<(QDebug debug, const QVoice &voice) |
bool | operator==(const QVoice &lhs, const QVoice &rhs) |
QDataStream & | operator>>(QDataStream &stream, QVoice &voice) |
To get a voice that is supported by the current text-to-speech engine, use QTextToSpeech::availableVoices().
The age of a voice.
Constant | Value | Description |
---|---|---|
QVoice::Child |
0 |
Voice of a child |
QVoice::Teenager |
1 |
Voice of a teenager |
QVoice::Adult |
2 |
Voice of an adult |
QVoice::Senior |
3 |
Voice of a senior |
QVoice::Other |
4 |
Voice of unknown age |
The gender of a voice.
Constant | Value | Description |
---|---|---|
QVoice::Male |
0 |
Voice of a male |
QVoice::Female |
1 |
Voice of a female |
QVoice::Unknown |
2 |
Voice of unknown gender |
[read-only]
age : const AgeThis property holds the age of a voice
Access functions:
QVoice::Age | age() const |
[read-only]
gender : const GenderThis property holds the gender of a voice
Access functions:
QVoice::Gender | gender() const |
[read-only, since 6.4]
locale : const QLocaleThis property holds the locale of the voice
The locale includes the language and the territory (i.e. accent or dialect) of the voice.
This property was introduced in Qt 6.4.
Access functions:
QLocale | locale() const |
[read-only]
name : const QStringThis property holds the name of a voice
Access functions:
QString | name() const |
Constructs a QVoice object by moving from other.
Copy-constructs a QVoice from other.
Constructs an empty QVoice.
Moves other into this QVoice object.
Assigns other to this QVoice object.
Destroys the QVoice instance.
[static]
QString QVoice::ageName(QVoice::Age age)Returns a string representing the age class of a voice.
[static]
QString QVoice::genderName(QVoice::Gender gender)̈́ Returns the gender name of a voice.
[since 6.4]
void QVoice::swap(QVoice &other)Swaps other with this voice. This operation is very fast and never fails.
This function was introduced in Qt 6.4.
Returns whether the lhs voice and the rhs voice are different.
[since 6.4]
QDataStream &operator<<(QDataStream &stream, const QVoice &voice)Serializes voice to data stream stream.
This function was introduced in Qt 6.4.
See also Serializing Qt Data Types.
[since 6.4]
QDebug operator<<(QDebug debug, const QVoice &voice)Writes information about voice to the debug stream.
This function was introduced in Qt 6.4.
See also QDebug.
Returns whether the lhs voice and the rhs voice are identical.
Two voices are identical if name, locale, gender, and age are identical, and if they belong to the same text-to-speech engine.
[since 6.4]
QDataStream &operator>>(QDataStream &stream, QVoice &voice)Deserializes voice from data stream stream.
This function was introduced in Qt 6.4.
See also Serializing Qt Data Types.