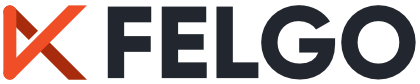
The QAxBase class is an abstract class that provides an API to initialize and access a COM object. More...
Header: | #include <QAxBase> |
qmake: | QT += axcontainer |
Inherited By: |
virtual void | clear() |
void | disableClassInfo() |
void | disableEventSink() |
void | disableMetaObject() |
bool | setControl(const int &) |
virtual bool | initialize(IUnknown **ptr) |
bool | initializeActive(IUnknown **ptr) |
bool | initializeFromFile(IUnknown **ptr) |
bool | initializeLicensed(IUnknown **ptr) |
bool | initializeRemote(IUnknown **ptr) |
The QAxBase class is an abstract class that provides an API to initialize and access a COM object.
QAxBase is an abstract class that cannot be used directly, and is instantiated through the subclasses QAxObject and QAxWidget. This class provides the API to access the COM object directly through its IUnknown implementation. If the COM object implements the IDispatch interface, the properties and methods of that object become available as Qt properties and slots.
connect(buttonBack, SIGNAL(clicked()), webBrowser, SLOT(GoBack()));
Properties exposed by the object's IDispatch implementation can be read and written through the property system provided by the Qt Object Model (both subclasses are QObjects, so you can use QObject::setProperty() and QObject::property()). Properties with multiple parameters are not supported.
activeX->setProperty("text", "some text"); int value = activeX->property("value");
Write-functions for properties and other methods exposed by the object's IDispatch implementation can be called directly using dynamicCall(), or indirectly as slots connected to a signal.
webBrowser->dynamicCall("GoHome()");
Outgoing events supported by the COM object are emitted as standard Qt signals.
connect(webBrowser, SIGNAL(TitleChanged(QString)), this, SLOT(setCaption(QString)));
QAxBase transparently converts between COM data types and the equivalent Qt data types. Some COM types have no equivalent Qt data structure.
Supported COM datatypes are listed in the first column of following table. The second column is the Qt type that can be used with the QObject property functions. The third column is the Qt type that is used in the prototype of generated signals and slots for in-parameters, and the last column is the Qt type that is used in the prototype of signals and slots for out-parameters.
COM type | Qt property | in-parameter | out-parameter |
---|---|---|---|
VARIANT_BOOL | bool | bool | bool& |
BSTR | QString | const QString& | QString& |
char, short, int, long | int | int | int& |
uchar, ushort, uint, ulong | uint | uint | uint& |
float, double | double | double | double& |
DATE | QDateTime | const QDateTime& | QDateTime& |
CY | qlonglong | qlonglong | qlonglong& |
OLE_COLOR | QColor | const QColor& | QColor& |
SAFEARRAY(VARIANT) | QList<QVariant> | const QList<QVariant>& | QList<QVariant>& |
SAFEARRAY(int), SAFEARRAY(double), SAFEARRAY(Date) | QList<QVariant> | const QList<QVariant>& | QList<QVariant>& |
SAFEARRAY(BYTE) | QByteArray | const QByteArray& | QByteArray& |
SAFEARRAY(BSTR) | QStringList | const QStringList& | QStringList& |
VARIANT | type-dependent | const QVariant& | QVariant& |
IFontDisp* | QFont | const QFont& | QFont& |
IPictureDisp* | QPixmap | const QPixmap& | QPixmap& |
IDispatch* | QAxObject* | QAxBase::asVariant() |
QAxObject* (return value) |
IUnknown* | QAxObject* | QAxBase::asVariant() |
QAxObject* (return value) |
SCODE, DECIMAL | unsupported | unsupported | unsupported |
VARIANT* (Since Qt 4.5) | unsupported | QVariant& | QVariant& |
Supported are also enumerations, and typedefs to supported types.
To call the methods of a COM interface described by the following IDL
dispinterface IControl { properties: [id(1)] BSTR text; [id(2)] IFontDisp *font; methods: [id(6)] void showColumn([in] int i); [id(3)] bool addColumn([in] BSTR t); [id(4)] int fillList([in, out] SAFEARRAY(VARIANT) *list); [id(5)] IDispatch *item([in] int i); };
use the QAxBase API like this:
QAxObject object("<CLSID>"); QString text = object.property("text").toString(); object.setProperty("font", QFont("Times New Roman", 12)); connect(this, SIGNAL(clicked(int)), &object, SLOT(showColumn(int))); bool ok = object.dynamicCall("addColumn(const QString&)", "Column 1").toBool(); QList<QVariant> varlist; QList<QVariant> parameters; parameters << QVariant(varlist); int n = object.dynamicCall("fillList(QList<QVariant>&)", parameters).toInt(); QAxObject *item = object.querySubItem("item(int)", 5);
Note that the QList the object should fill has to be provided as an element in the parameter list of QVariants.
If you need to access properties or pass parameters of unsupported datatypes you must access the COM object directly through its IDispatch
implementation or other interfaces. Those interfaces can be retrieved
through queryInterface().
IUnknown *iface = 0; activeX->queryInterface(IID_IUnknown, (void**)&iface); if (iface) { // use the interface iface->Release(); }
To get the definition of the COM interfaces you will have to use the header files provided with the component you want to use. Some compilers can also import type libraries using the #import compiler directive. See the component documentation to find out which type libraries you have to import, and how to use them.
If you need to react to events that pass parameters of unsupported datatypes you can use the generic signal that delivers the event data as provided by the COM event.
See also QAxObject, QAxWidget, QAxScript, and ActiveQt Framework.
[virtual]
void QAxBase::clear()Disconnects and destroys the COM object.
If you reimplement this function you must also reimplement the destructor to call clear(), and call this implementation at the end of your clear() function.
Disables the class info generation for this ActiveX container. If you don't require any class information about the ActiveX control use this function to speed up the meta object generation.
Note that this function must be called immediately after construction of the object
Disables the event sink implementation for this ActiveX container. If you don't intend to listen to the ActiveX control's events use this function to speed up the meta object generation.
Some ActiveX controls might be unstable when connected to an event sink. To get OLE events you must use standard COM methods to register your own event sink. Use queryInterface() to get access to the raw COM object.
Note that this function should be called immediately after construction of the object.
Disables the meta object generation for this ActiveX container. This also disables the event sink and class info generation. If you don't intend to use the Qt meta object implementation call this function to speed up instantiation of the control. You will still be able to call the object through dynamicCall(), but signals, slots and properties will not be available with QObject APIs.
Some ActiveX controls might be unstable when used with OLE automation. Use standard COM methods to use those controls through the COM interfaces provided by queryInterface().
Note that this function must be called immediately after construction of the object.
[virtual protected]
bool QAxBase::initialize(IUnknown
**ptr)This virtual function is called by setControl() and creates the requested COM object. ptr is set to the object's IUnknown implementation. The function returns true if the object initialization succeeded; otherwise the function returns false.
The default implementation interprets the string returned by control(), and calls initializeRemote(), initializeLicensed() or initializeActive() if the string matches the respective patterns. If control() is the name of an existing file, initializeFromFile() is called. If no pattern is matched, or if remote or licensed initialization fails, CoCreateInstance is used directly to create the object.
See the control property documentation for details about supported patterns.
The interface returned in ptr must be referenced exactly once when this function returns. The interface provided by e.g. CoCreateInstance is already referenced, and there is no need to reference it again.
[protected]
bool QAxBase::initializeActive(IUnknown **ptr)Connects to an active instance running on the current machine, and returns the IUnknown interface to the running object in ptr. This function returns true if successful, otherwise returns false.
This function is called by initialize() if the control string contains the substring "}&".
See also initialize().
[protected]
bool QAxBase::initializeFromFile(IUnknown **ptr)Creates the COM object handling the filename in the control property, and returns the IUnknown interface to the object in ptr. This function returns true if successful, otherwise returns false.
This function is called by initialize() if the control string is the name of an existing file.
See also initialize().
[protected]
bool QAxBase::initializeLicensed(IUnknown **ptr)Creates an instance of a licensed control, and returns the IUnknown interface to the object in ptr. This functions returns true if successful, otherwise returns false.
This function is called by initialize() if the control string contains the substring "}:". The license key needs to follow this substring.
See also initialize().
[protected]
bool QAxBase::initializeRemote(IUnknown **ptr)Creates the instance on a remote server, and returns the IUnknown interface to the object in ptr. This function returns true if successful, otherwise returns false.
This function is called by initialize() if the control string contains the substring "/{". The information about the remote machine needs to be provided in front of the substring.
See also initialize().