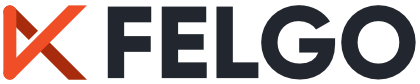
Abstract model mapper class for candlestick series. More...
Header: | #include <QCandlestickModelMapper> |
Since: | Qt 5.8 |
Inherits: | QObject |
Inherited By: |
QCandlestickModelMapper(QObject *parent = nullptr) | |
QAbstractItemModel * | model() const |
virtual Qt::Orientation | orientation() const = 0 |
QCandlestickSeries * | series() const |
void | setModel(QAbstractItemModel *model) |
void | setSeries(QCandlestickSeries *series) |
void | modelReplaced() |
void | seriesReplaced() |
int | close() const |
int | firstSetSection() const |
int | high() const |
int | lastSetSection() const |
int | low() const |
int | open() const |
void | setClose(int close) |
void | setFirstSetSection(int firstSetSection) |
void | setHigh(int high) |
void | setLastSetSection(int lastSetSection) |
void | setLow(int low) |
void | setOpen(int open) |
void | setTimestamp(int timestamp) |
int | timestamp() const |
Abstract model mapper class for candlestick series.
Model mappers allow the use of a QAbstractItemModel-derived model as a data source for a chart series, creating a connection between a QCandlestickSeries and the model object. A model mapper maintains an equal size across all QCandlestickSets.
Note: The model used must support adding and removing rows/columns and modifying the data of the cells.
Defines the model that is used by the mapper.
Access functions:
QAbstractItemModel * | model() const |
void | setModel(QAbstractItemModel *model) |
Notifier signal:
void | modelReplaced() |
Defines the QCandlestickSeries object that is used by the mapper.
Note: All data in the series is discarded when it is set to the mapper. When a new series is specified, the old series is disconnected (preserving its data).
Access functions:
QCandlestickSeries * | series() const |
void | setSeries(QCandlestickSeries *series) |
Notifier signal:
void | seriesReplaced() |
Constructs a model mapper object as a child of parent.
[protected]
int QCandlestickModelMapper::close() constReturns the row/column of the model that contains the close values of the sets in the series. Default value is -1 (invalid mapping).
See also setClose().
[protected]
int QCandlestickModelMapper::firstSetSection() constReturns the section of the model that is used as the data source for the first candlestick set. Default value is -1 (invalid mapping).
See also setFirstSetSection().
[protected]
int QCandlestickModelMapper::high() constReturns the row/column of the model that contains the high values of the sets in the series. Default value is -1 (invalid mapping).
See also setHigh().
[protected]
int QCandlestickModelMapper::lastSetSection() constReturns the section of the model that is used as the data source for the last candlestick set. Default value is -1 (invalid mapping).
See also setLastSetSection().
[protected]
int QCandlestickModelMapper::low() constReturns the row/column of the model that contains the low values of the sets in the series. Default value is -1 (invalid mapping).
See also setLow().
[signal]
void QCandlestickModelMapper::modelReplaced()Emitted when the model, to which the mapper is connected, has changed.
Note: Notifier signal for property model.
See also model.
[protected]
int QCandlestickModelMapper::open() constReturns the row/column of the model that contains the open values of the sets in the series. Default value is -1 (invalid mapping).
See also setOpen().
[pure virtual]
Qt::Orientation QCandlestickModelMapper::orientation() constReturns the orientation that is used when QCandlestickModelMapper accesses the model. This determines whether the consecutive values of the set are read from rows (Qt::Horizontal) or from columns (Qt::Vertical).
[signal]
void QCandlestickModelMapper::seriesReplaced()Emitted when the series to which mapper is connected to has changed.
Note: Notifier signal for property series.
See also series.
[protected]
void QCandlestickModelMapper::setClose(int
close)Sets the row/column of the model that contains the close values of the sets in the series. Default value is -1 (invalid mapping).
See also close().
[protected]
void QCandlestickModelMapper::setFirstSetSection(int firstSetSection)Sets the section of the model that is used as the data source for the first candlestick set. Parameter firstSetSection specifies the section of the model. Default value is -1.
See also firstSetSection().
[protected]
void QCandlestickModelMapper::setHigh(int high)Sets the row/column of the model that contains the high values of the sets in the series. Default value is -1 (invalid mapping).
See also high().
[protected]
void QCandlestickModelMapper::setLastSetSection(int lastSetSection)Sets the section of the model that is used as the data source for the last candlestick set. Parameter lastSetSection specifies the section of the model. Default value is -1.
See also lastSetSection().
[protected]
void QCandlestickModelMapper::setLow(int low)Sets the row/column of the model that contains the low values of the sets in the series. Default value is -1 (invalid mapping).
See also low().
[protected]
void QCandlestickModelMapper::setOpen(int open)Sets the row/column of the model that contains the open values of the sets in the series. Default value is -1 (invalid mapping).
See also open().
[protected]
void QCandlestickModelMapper::setTimestamp(int
timestamp)Sets the row/column of the model that contains the timestamp values of the sets in the series. Default value is -1 (invalid mapping).
See also timestamp().
[protected]
int QCandlestickModelMapper::timestamp() constReturns the row/column of the model that contains the timestamp values of the sets in the series. Default value is -1 (invalid mapping).
See also setTimestamp().