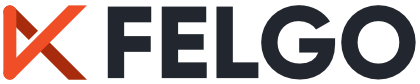
Queries attached gamepads and related events. More...
Header: | #include <QGamepadManager> |
qmake: | QT += gamepad |
Inherits: | QObject |
flags | GamepadAxes |
enum | GamepadAxis { AxisInvalid, AxisLeftX, AxisLeftY, AxisRightX, AxisRightY } |
enum | GamepadButton { ButtonInvalid, ButtonA, ButtonB, ButtonX, ..., ButtonGuide } |
flags | GamepadButtons |
const QList<int> | connectedGamepads() const |
QString | gamepadName(int deviceId) const |
bool | isGamepadConnected(int deviceId) const |
bool | configureAxis(int deviceId, QGamepadManager::GamepadAxis axis) |
bool | configureButton(int deviceId, QGamepadManager::GamepadButton button) |
bool | isConfigurationNeeded(int deviceId) const |
void | resetConfiguration(int deviceId) |
bool | setCancelConfigureButton(int deviceId, QGamepadManager::GamepadButton button) |
void | setSettingsFile(const QString &file) |
void | axisConfigured(int deviceId, QGamepadManager::GamepadAxis axis) |
void | buttonConfigured(int deviceId, QGamepadManager::GamepadButton button) |
void | configurationCanceled(int deviceId) |
void | connectedGamepadsChanged() |
void | gamepadAxisEvent(int deviceId, QGamepadManager::GamepadAxis axis, double value) |
void | gamepadButtonPressEvent(int deviceId, QGamepadManager::GamepadButton button, double value) |
void | gamepadButtonReleaseEvent(int deviceId, QGamepadManager::GamepadButton button) |
void | gamepadConnected(int deviceId) |
void | gamepadDisconnected(int deviceId) |
void | gamepadNameChanged(int deviceId, const QString &name) |
QGamepadManager * | instance() |
Queries attached gamepads and related events.
QGamepadManager provides a high-level interface for querying the attached gamepads and events related to all of the connected devices.
The GamepadAxes type is a typedef for QFlags<GamepadAxis>. It stores an OR combination of GamepadAxis values.
The GamepadButtons type is a typedef for QFlags<GamepadButton>. It stores an OR combination of GamepadButton values.
Access functions:
const QList<int> | connectedGamepads() const |
Notifier signal:
void | connectedGamepadsChanged() |
[signal]
void QGamepadManager::axisConfigured(int
deviceId, QGamepadManager::GamepadAxis axis)[signal]
void QGamepadManager::buttonConfigured(int deviceId, QGamepadManager::GamepadButton button)[signal]
void QGamepadManager::configurationCanceled(int deviceId)[slot]
bool QGamepadManager::configureAxis(int
deviceId, QGamepadManager::GamepadAxis axis)Configures axis on the gamepad with the specified deviceId. Returns true
in case of success.
[slot]
bool QGamepadManager::configureButton(int
deviceId, QGamepadManager::GamepadButton button)Configures the specified button on the gamepad with this deviceId. Returns true
in case of success.
Returns a QList containing the deviceId values of the connected gamepads.
Note: Getter function for property connectedGamepads.
[signal]
void QGamepadManager::gamepadAxisEvent(int deviceId, QGamepadManager::GamepadAxis axis, double value)[signal]
void QGamepadManager::gamepadButtonPressEvent(int deviceId, QGamepadManager::GamepadButton button, double value)[signal]
void QGamepadManager::gamepadButtonReleaseEvent(int deviceId, QGamepadManager::GamepadButton button)[signal]
void QGamepadManager::gamepadConnected(int deviceId)[signal]
void QGamepadManager::gamepadDisconnected(int deviceId)Returns the name of the gamepad identified by deviceId. If deviceId does not identify a connected gamepad, returns an empty string.
This function was introduced in Qt 5.11.
[signal]
void QGamepadManager::gamepadNameChanged(int deviceId, const QString &name)[static]
QGamepadManager *QGamepadManager::instance()Returns the instance of the QGamepadManager.
[slot]
bool QGamepadManager::isConfigurationNeeded(int deviceId) constReturns a boolean indicating whether configuration is needed for the specified deviceId.
Returns a boolean indicating whether the gamepad with the specified deviceId is connected or not.
[slot]
void QGamepadManager::resetConfiguration(int deviceId)Resets the configuration on the gamepad with the specified deviceId.
[slot]
bool QGamepadManager::setCancelConfigureButton(int deviceId, QGamepadManager::GamepadButton button)Configures button as the cancel button on the gamepad with id deviceId. Returns true
in case of success.
[slot]
void QGamepadManager::setSettingsFile(const QString &file)Sets the name of the file that stores the button and axis configuration data.