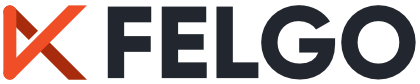
The QtWin namespace contains miscellaneous Windows-specific functions. More...
Header: | #include <QtWin> |
qmake: | QT += winextras |
enum | HBitmapFormat { HBitmapNoAlpha, HBitmapPremultipliedAlpha, HBitmapAlpha } |
enum | WindowFlip3DPolicy { FlipDefault, FlipExcludeAbove, FlipExcludeBelow } |
Q_DECL_IMPORT | disableBlurBehindWindow(QWindow *window) |
void | disableBlurBehindWindow(int *window) |
Q_DECL_IMPORT | enableBlurBehindWindow(QWindow *window, const int ®ion) |
Q_DECL_IMPORT | enableBlurBehindWindow(QWindow *window) |
void | enableBlurBehindWindow(int *window, const int ®ion) |
void | enableBlurBehindWindow(int *window) |
Q_DECL_IMPORT | extendFrameIntoClientArea(QWindow *window, int left, int top, int right, int bottom) |
Q_DECL_IMPORT | extendFrameIntoClientArea(QWindow *window, const QMargins &margins) |
void | extendFrameIntoClientArea(int *window, const QMargins &margins) |
void | extendFrameIntoClientArea(int *window, int left, int top, int right, int bottom) |
Q_DECL_IMPORT | isCompositionEnabled() |
Q_DECL_IMPORT | isCompositionOpaque() |
Q_DECL_IMPORT | isWindowExcludedFromPeek(QWindow *window) |
bool | isWindowExcludedFromPeek(int *window) |
Q_DECL_IMPORT | isWindowPeekDisallowed(QWindow *window) |
bool | isWindowPeekDisallowed(int *window) |
Q_DECL_IMPORT | markFullscreenWindow(QWindow *window, bool fullscreen = true) |
void | markFullscreenWindow(int *window, bool fullscreen = true) |
Q_DECL_IMPORT | resetExtendedFrame(QWindow *window) |
void | resetExtendedFrame(int *window) |
Q_DECL_IMPORT | setCompositionEnabled(bool enabled) |
Q_DECL_IMPORT | setCurrentProcessExplicitAppUserModelID(const class QString &id) |
Q_DECL_IMPORT | setWindowDisallowPeek(QWindow *window, bool disallow) |
void | setWindowDisallowPeek(int *window, bool disallow) |
Q_DECL_IMPORT | setWindowExcludedFromPeek(QWindow *window, bool exclude) |
void | setWindowExcludedFromPeek(int *window, bool exclude) |
Q_DECL_IMPORT | setWindowFlip3DPolicy(QWindow *window, QtWin::WindowFlip3DPolicy policy) |
void | setWindowFlip3DPolicy(int *window, QtWin::WindowFlip3DPolicy policy) |
Q_DECL_IMPORT | taskbarActivateTab(QWindow *window) |
void | taskbarActivateTab(int *window) |
Q_DECL_IMPORT | taskbarActivateTabAlt(QWindow *window) |
void | taskbarActivateTabAlt(int *window) |
Q_DECL_IMPORT | taskbarAddTab(QWindow *window) |
void | taskbarAddTab(int *window) |
Q_DECL_IMPORT | taskbarDeleteTab(QWindow *window) |
void | taskbarDeleteTab(int *window) |
QtWin::WindowFlip3DPolicy | windowFlip3DPolicy(int *window) |
The QtWin namespace contains miscellaneous Windows-specific functions.
This enum defines how the conversion between HBITMAP
and QPixmap is performed.
Constant | Value | Description |
---|---|---|
QtWin::HBitmapNoAlpha |
0 |
The alpha channel is ignored and always treated as being set to fully opaque. This is preferred if the HBITMAP is used with standard GDI calls, such as BitBlt() . |
QtWin::HBitmapPremultipliedAlpha |
1 |
The HBITMAP is treated as having an alpha channel and premultiplied colors. This is preferred if the HBITMAP is accessed through the AlphaBlend() GDI
function. |
QtWin::HBitmapAlpha |
2 |
The HBITMAP is treated as having a plain alpha channel. This is the preferred format if the HBITMAP is going to be used as an application icon or a systray icon. |
This enum was introduced or modified in Qt 5.2.
See also fromHBITMAP() and toHBITMAP().
This enum type specifies the Flip3D window policy.
Constant | Value | Description |
---|---|---|
QtWin::FlipDefault |
0 |
Let the OS decide whether to include the window in the Flip3D rendering. |
QtWin::FlipExcludeAbove |
2 |
Exclude the window from Flip3D and display it above the Flip3D rendering. |
QtWin::FlipExcludeBelow |
1 |
Exclude the window from Flip3D and display it below the Flip3D rendering. |
This enum was introduced or modified in Qt 5.2.
See also setWindowFlip3DPolicy().
Disables the previously enabled blur effect for the specified window.
This function was introduced in Qt 5.2.
See also enableBlurBehindWindow().
This function overloads QtWin::disableBlurBehindWindow().
This function was introduced in Qt 5.2.
Enables the blur effect for the specified region of the specified window.
This function was introduced in Qt 5.2.
See also disableBlurBehindWindow().
Enables the blur effect for the specified window.
This function was introduced in Qt 5.2.
See also disableBlurBehindWindow().
This function overloads QtWin::enableBlurBehindWindow().
This function was introduced in Qt 5.2.
This function overloads QtWin::enableBlurBehindWindow().
This function was introduced in Qt 5.2.
Extends the glass frame into the client area of the specified window using the left, top, right, and bottom margin values.
Pass -1 as values for any of the four margins to fully extend the frame, creating a sheet of glass effect.
If you want the extended frame to act like a standard window border, you should handle that yourself.
Note: If window is a QWidget handle, set the Qt::WA_NoSystemBackground attribute for your widget.
This function was introduced in Qt 5.2.
See also resetExtendedFrame().
This function overloads QtWin::extendFrameIntoClientArea().
Extends the glass frame into the client area of the specified window using the specified margins.
This function was introduced in Qt 5.2.
This function overloads QtWin::extendFrameIntoClientArea().
Convenience overload that allows passing frame sizes in a margins structure.
This function was introduced in Qt 5.2.
This function overloads QtWin::extendFrameIntoClientArea().
This function was introduced in Qt 5.2.
Returns the DWM composition state.
This function was introduced in Qt 5.2.
Returns true if the specified window is excluded from Aero Peek.
This function was introduced in Qt 5.2.
This function overloads QtWin::isWindowExcludedFromPeek().
This function was introduced in Qt 5.2.
Returns true if Aero Peek is disallowed on the thumbnail of the specified window.
This function was introduced in Qt 5.2.
This function overloads QtWin::isWindowPeekDisallowed().
This function was introduced in Qt 5.2.
Marks the specified window as running in the full-screen mode if fullscreen is true, so that the shell handles it correctly. Otherwise, removes the mark.
Note: You do not usually need to call this function, because the Windows taskbar always tries to determine whether a window is running in the full-screen mode.
This function was introduced in Qt 5.2.
This function overloads QtWin::markFullscreenWindow().
This function was introduced in Qt 5.2.
Resets the glass frame and restores the window attributes.
This convenience function calls extendFrameIntoClientArea() with margins set to 0.
Note: You must unset the Qt::WA_NoSystemBackground attribute for extendFrameIntoClientArea() to work.
This function was introduced in Qt 5.2.
See also extendFrameIntoClientArea().
This function overloads QtWin::resetExtendedFrame().
This function was introduced in Qt 5.2.
See also isCompositionEnabled().
Sets the Application User Model ID id.
For more information, see Application User Model IDs.
This function was introduced in Qt 5.2.
Disables Aero Peek for the specified window when hovering over the taskbar thumbnail of the window with the mouse pointer if disallow is true; otherwise allows it.
The default is false.
This function was introduced in Qt 5.2.
This function overloads QtWin::setWindowDisallowPeek().
This function was introduced in Qt 5.2.
Excludes the specified window from Aero Peek if exclude is true.
This function was introduced in Qt 5.2.
See also isWindowExcludedFromPeek().
This function overloads QtWin::setWindowExcludedFromPeek().
This function was introduced in Qt 5.2.
Sets the Flip3D policy policy for the specified window.
This function was introduced in Qt 5.2.
See also windowFlip3DPolicy().
This function overloads QtWin::setWindowFlip3DPolicy().
This function was introduced in Qt 5.2.
Activates an item on the taskbar without activating the window itself.
This function was introduced in Qt 5.2.
This function overloads QtWin::taskbarActivateTab().
This function was introduced in Qt 5.2.
Marks the item that represents the specified window on the taskbar as active, but does not activate it visually.
This function was introduced in Qt 5.2.
This function overloads QtWin::taskbarActivateTabAlt().
This function was introduced in Qt 5.2.
Adds an item for the specified window to the taskbar.
This function was introduced in Qt 5.2.
This function overloads QtWin::taskbarAddTab().
This function was introduced in Qt 5.2.
Removes the specified window from the taskbar.
This function was introduced in Qt 5.2.
This function overloads QtWin::taskbarDeleteTab().
This function was introduced in Qt 5.2.
This function overloads QtWin::windowFlip3DPolicy().
This function was introduced in Qt 5.2.
See also setWindowFlip3DPolicy().