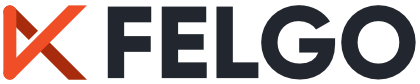
The QWindowsWindowFunctions class is an inline class containing miscellaneous functionality for Windows window specific functionality. More...
Header: | #include <QWindowsWindowFunctions> |
Since: | Qt 5.5 |
typedef | IsTabletModeType |
typedef | SetHasBorderInFullScreen |
typedef | SetTouchWindowTouchType |
typedef | SetWindowActivationBehaviorType |
enum | TouchWindowTouchType { NormalTouch, FineTouch, WantPalmTouch } |
int | Q_DECLARE_FLAGS(int, QWindowsWindowFunctions::TouchWindowTouchType) |
bool | isTabletMode() |
const int | isTabletModeIdentifier() |
void | setHasBorderInFullScreen(int *window, bool border) |
const int | setHasBorderInFullScreenIdentifier() |
void | setTouchWindowTouchType(int *window, int type) |
const int | setTouchWindowTouchTypeIdentifier() |
void | setWindowActivationBehavior(int behavior) |
const int | setWindowActivationBehaviorIdentifier() |
The QWindowsWindowFunctions class is an inline class containing miscellaneous functionality for Windows window specific functionality.
A common usage pattern is as follows:
int main(int argc, char **argv) { QApplication app(argc, argv); QPushButton topLevelWidget("Hello World!"); topLevelWidget.winId(); //have to create the QWindow QWindow *tlwWindow = topLevelWidget.windowHandle(); QWindowsWindowFunctions::setTouchWindowTouchType(tlwWindow, QWindowsWindowFunctions::WantPalmTouch); topLevelWidget.show(); return app.exec(); }
Note: There is no binary compatibility guarantee for this class, meaning that an application using it is only guaranteed to work with the Qt version it was developed against.
This is the typedef for the function returned by QGuiApplication::platformFunction() when passed isTabletModeIdentifier().
This typedef was introduced in Qt 5.9.
This is the typedef for the function returned by QGuiApplication::platformFunction when passed setHasBorderInFullScreenIdentifier.
This is the typedef for the function returned by QGuiApplication::platformFunction when passed setTouchWindowTouchTypeIdentifier.
This is the typedef for the function returned by QGuiApplication::platformFunction() when passed setWindowActivationBehaviorIdentifier().
This typedef was introduced in Qt 5.7.
See also QWidget::activateWindow() and QWindow::requestActivate().
This enum represents the supported TouchWindow touch flags for RegisterTouchWindow().
Constant | Value |
---|---|
QWindowsWindowFunctions::NormalTouch |
0x00000000 |
QWindowsWindowFunctions::FineTouch |
0x00000001 |
QWindowsWindowFunctions::WantPalmTouch |
0x00000002 |
[static]
bool QWindowsWindowFunctions::isTabletMode()This is a convenience function that can be used directly instead of resolving the function pointer. Returns true if Windows 10 operates in Tablet Mode. In this mode, Windows forces all application main windows to open in maximized state. Applications should then avoid resizing windows or restoring geometries to non-maximized states.
This function was introduced in Qt 5.9.
See also QWidget::showMaximized(), QWidget::saveGeometry(), and QWidget::restoreGeometry().
[static]
const int QWindowsWindowFunctions::isTabletModeIdentifier()Returns a bytearray that can be used to query QGuiApplication::platformFunction() to retrieve the IsTabletModeType function.
This function was introduced in Qt 5.9.
[static]
void QWindowsWindowFunctions::setHasBorderInFullScreen(int *window, bool border)[static]
const int
QWindowsWindowFunctions::setHasBorderInFullScreenIdentifier()This function returns the bytearray that can be used to query QGuiApplication::platformFunction to retrieve the SetHasBorderInFullScreen function.
This function was introduced in Qt 5.6.
[static]
void QWindowsWindowFunctions::setTouchWindowTouchType(int *window, int type)[static]
const int
QWindowsWindowFunctions::setTouchWindowTouchTypeIdentifier()This function returns the bytearray that can be used to query QGuiApplication::platformFunction to retrieve the SetTouchWindowTouchType function.
[static]
void QWindowsWindowFunctions::setWindowActivationBehavior(int behavior)This is a convenience function that can be used directly instead of resolving the function pointer. behavior will be relayed to the function retrieved by QGuiApplication.
This function was introduced in Qt 5.7.
See also QWidget::activateWindow() and QWindow::requestActivate().
[static]
const int
QWindowsWindowFunctions::setWindowActivationBehaviorIdentifier()This function returns a bytearray that can be used to query QGuiApplication::platformFunction() to retrieve the SetWindowActivationBehaviorType function.
This function was introduced in Qt 5.7.
See also QWidget::activateWindow() and QWindow::requestActivate().