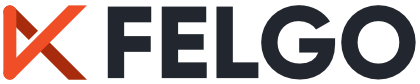
The RevoluteJoint forces two bodies to share a common anchor point around which the bodies rotate. More...
Import Statement: | import Felgo 4.0 |
Inherits: | |
Inherited By: |
The RevoluteJoint rotates two bodies around a common anchor point with a single degree of freedom: the relative rotation of the two bodies. The relative angle between the bodies is 0 after connection and is positive when bodyB rotates counter-clockwise about the localAnchorA.
To control the angle between two bodies there are 2 options:
You can set the lowerAngle and upperAngle property to limit the angle between two bodies. To enable these limits, set enableLimit to true.
For applying a torque about the localAnchorA, you can set the motorSpeed and maxMotorTorque properties. For enabling them, set enableMotor to true.
For a comprehensive documentation of all joints see the Box2D documentation at http://www.box2d.org/manual.html#_Toc258082974.
The following example shows how two physics bodies connected with a RevoluteJoint and the motor torque is toggled by clicking on the Scene. By pressing the left and right keys, the motor speed is modified. The source code of the full example is provided in the revolute folder of the Box2D Examples.
import QtQuick 2.0 import Felgo 4.0 GameWindow { id: screen Keys.onPressed: onKeysPressed(event) function onKeysPressed(event) { if (event.key === Qt.Key_Left) { revolute.motorSpeed += 5 console.debug("decreasing motor speed to", revolute.motorSpeed) } else if (event.key === Qt.Key_Right) { revolute.motorSpeed -= 5 console.debug("increasing motor speed to", revolute.motorSpeed) } } Scene { PhysicsWorld { id: world anchors.fill: parent z:1 // for putting the debugDraw on top of the entities in QML renderer } Wall { id: ground height: 20 anchors { left: parent.left; right: parent.right; top: parent.bottom } } Wall { id: ceiling height: 20 anchors { left: parent.left; right: parent.right; bottom: parent.top } } Wall { id: leftWall width: 20 anchors { right: parent.left; bottom: ground.top; top: ceiling.bottom } } Wall { id: rightWall width: 20 anchors { left: parent.right; bottom: ground.top; top: ceiling.bottom } } Square { id: rod x: world.width/2+middleBall.radius+15 y: world.height/2 width: 80 height: 20 } Ball { id: middleBall x: world.width/2 y: world.height/2 radius: 20 } RevoluteJoint { id: revolute maxMotorTorque: 30000 // initially, the speed is 0 - it gets modified by pressing left and right keys motorSpeed: 0 bodyA: middleBall.body bodyB: rod.body enableMotor: true // start with enabled motor, to toggle click on the window } Text { z: 1 // put on top of DebugDraw in QML renderer color: "white" text: "Press left and right keys to change the RevoluteJoint motorSpeed" } } // end of Scene EntityManager { id: entityManager } MouseArea { anchors.fill: parent onClicked: revolute.enableMotor = !revolute.enableMotor } }
enableLimit : bool |
Set this property to true for lowerAngle and upperAngle to have an effect. By default, it is false.
enableMotor : bool |
Set this property to true for maxMotorTorque and motorSpeed to have an effect. By default, it is false.
localAnchorA : point |
The local anchor point relative to the Joint::bodyA center in pixels.
The default is (0, 0), which means the center of Joint::bodyA.
localAnchorB : point |
The local anchor point relative to the Joint::bodyB center in pixels.
The default is (0, 0), which means the center of Joint::bodyB.
lowerAngle : real |
The lower limit for the revolute joint angle range in degrees. To enable it, set enableLimit to true.
Note: The limit range should include zero, otherwise the joint will lurch when the simulation begins.
maxMotorTorque : real |
The maximum torque in kg*pixels^2/second^2. To enable it, set enableMotor to true.
The joint motor will maintain the specified motorSpeed unless the required torque exceeds this maximum. When the maximum torque is exceeded, the joint will slow down and can even reverse.
motorSpeed : real |
The speed of the motor in degrees per second. To enable it, set enableMotor to true.
upperAngle : real |
The upper limit for the revolute joint angle range in degrees. To enable it, set enableLimit to true.
Note: The limit range should include zero, otherwise the joint will lurch when the simulation begins.