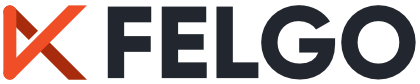
The Base component for BoxCollider, CircleCollider and PolygonCollider. All physics colliders including BoxCollider, CircleCollider and PolygonCollider inherit from ColliderBase. More...
Import Statement: | import Felgo 4.0 |
Inherited By: |
It is a convenience component for a physics Body with support for forwarding the position change of the physics body to the contained entity. But it also forwards the position change of the entity to the physics system, if collisionTestingOnlyMode is set to true. See Type of Games Using Physics for an explanation of these 2 type of games.
The derived components need to overwrite the fixtures property and add the fixture(s) that define the physics shape. The most common components include a single Box, Circle or Polygon fixture, for which there already exist corresponding Felgo components BoxCollider, CircleCollider and PolygonCollider.
If you need more complex fixtures, inherit from the ColliderBase.
The CircleCollider for example is implemented like the following:
import Felgo ColliderBase { id: collider objectName: "circleCollider" property alias fixture: fixture property alias friction: fixture.friction property alias restitution: fixture.restitution property alias density: fixture.density property alias categories: fixture.categories property alias collidesWith: fixture.collidesWith property alias sensor: fixture.sensor property alias groupIndex: fixture.groupIndex property alias radius: fixture.radius fixtures: Circle { id: fixture x: collider.x y: collider.y sensor: collisionTestingOnlyMode } }
active : bool |
This property holds whether the physics Body is active, i.e. if it is affected by the physics simulation.
This property is connected to the EntityBase::collidersActive property, which is by default true. If you set it to true, but the collidersActive property is false, this collider will still be inactive!
See also Body::active.
angularDamping : real |
This property holds the damping of linearVelocity. Damping is used to reduce the world velocity of bodies. Damping is different than friction because friction only occurs with contact. Damping is not a replacement for friction and the two effects should be used together.
Damping parameters should be between 0 and infinity, with 0 meaning no damping, and infinity meaning full damping. Normally you will use a damping value between 0 and 0.1.
The default value is 0.
See also angularVelocity and linearDamping.
angularVelocity : real |
This property holds the angular speed of the body at the center of mass in radians/second.
See also Body::angularVelocity.
body : Body |
This property alias allows access to the physics Body of this component.
Usually, you will not directly need to access this property, because you can access all body properties by the provided alias properties.
It can be used to convert vectors and points between world and local coordinates with toWorldVector(), toWorldPoint(), toLocalVector() and toLocalPoint() methods.
bodyType : BodyType |
Set this property to either Body.Static, Body.Dynamic or Body.Kinetic. The is required so the Body enum is known.
Use the Static type for non-moving objects. Note that only dynamic bodies can collide with each other.
By default, bodyType is set to Body.Dynamic.
See also Body::bodyType.
bullet : bool |
Set this flag to true for a very fast moving, small body that may tunnel through other bodies.
The default value is false.
See also Body::bullet.
collisionTestingOnlyMode : bool |
Set this property to false when the position & rotation should be updated by Box2D (the default value). When you set it to true, the positions are taken from the target and the colliders are only used for collision detection but not for position updating.
The target is set to the owningEntity in the ColliderBase, so any position update of the entity gets forwarded to the physics system. So when you set it to true, the entity position gets modified from other components like MoveToPointHelper or NumberAnimation).
If you set this property to true, the fixture in the derived collider classes BoxCollider, CircleCollider and PolygonCollider get set their Fixture::isSensor flag to true and sleepingAllowed to false. Otherwise no position updating would be possible.
By default, this property is set to false
.
See also Body::collisionTestingOnlyMode.
fixedRotation : bool |
Set this property to true if you do not want the body to rotate. The default value is false, so the body can freely rotate.
See also Body::fixedRotation.
Use this property to add fixtures in your derived components. Only set this property when directly creating from ColliderBase, and not when using BoxCollider, CircleCollider or PolygonCollider.
Here is an example:
ColliderBase { fixtures: Circle { id: fixture x: collider.x y: collider.y sensor: collisionTestingOnlyMode } }
See also Body::fixtures.
force : point |
If you set this property, this force is applied continuously every frame from the center of the body. The force is set in kg*pixels/s^2 and is 0 by default.
It is a convenience function for applying forces, which should usually be done over a longer time period. So instead of setting up a Timer object and call applyForce() in there, you can set this property to anything non-zero and it is applied automatically.
If you want to apply a force from an origin point that is not the body center, you can use applyForce(). An applied force from no center has the effect of the object being rotated. Except if the fixedRotation property is set.
Note: A force is only applied when the bodyType is not set to Body.Static. Applying the force wakes up the body, so awake gets set to true if it was false before.
See also Body::force and Body::applyForce().
gravityScale : real |
Scales the gravity defined in the PhysicsWorld before being applied to this body. A scale of 0 would mean this body is not affected by gravity.
The default value is 1.0, so the defined gravity is unchanged.
See also Body::gravityScale.
linearDamping : real |
This property holds the damping of linearVelocity. Damping is used to reduce the world velocity of bodies. Damping is different than friction because friction only occurs with contact. Damping is not a replacement for friction and the two effects should be used together.
Damping parameters should be between 0 and infinity, with 0 meaning no damping, and infinity meaning full damping. Normally you will use a damping value between 0 and 0.1.
The default value is 0.
See also linearVelocity and angularDamping.
linearVelocity : point |
The linearVelocity holds the current speed in x and y direction of the physics body at the center of mass in local coordinates in pixels/second.
If you want to retrieve the world coordinates of the current velocity, use Body::toWorldVector(linearVelocity).
You can also calculate the rotation of a local linearVelocity by using Math::atan2(). Consider this example: MyGameBuildEntityButton.qml:
// get the current local forward vector var localForward = linearVelocity; // mirror the current velocity horizontally localForward.x *= -1; // calculate the new angle of the mirrored direction var newAngle = Math.atan2(localForward.y, localForward.x); // set the mirrored angle to the entity AND to the collider, as the rotation of the entity is not automatically forwarded because collisionTestingOnlyMode is set to false entity.rotation = newAngle; collider.rotation = newAngle;
See also Body::linearVelocity.
sleeping : bool |
Read this this flag to know if this physics body with bodyType Body.Dynamic or Body.Kinetic is sleeping.
This is a read-only property and only for detecting if the body is sleeping - to control if sleeping is allowed use sleepingAllowed.
See also sleepingAllowed and Body::bodyType.
sleepingAllowed : bool |
This property holds whether the body is allowed to get inactive for performance optimizations. The default value is the inverse of collisionTestingOnlyMode, so sleepingAllowed: !collisionTestingOnlyMode
. This is needed to avoid sleeping when the collider gets used for collision
detection, because if no forces or impulses are applied to the body it will sleep by default.
If collisionTestingOnlyMode is left at its default value (Which is false), this property has the default value true
.
See also Body::sleepingAllowed.
torque : real |
If you set this property, this torque is applied continuously every frame. This affects the angular velocity without affecting the linear velocity. The torque is in kg*degrees/second^2 and is 0 by default.
This is a convenience function for applying torques, which should usually be done over a longer time period. So instead of setting up a Timer object and call applyTorque() in there, you can set this property to anything non-zero and it is applied automatically.
Note: A torque is only applied when the bodyType is not set to Body.Static. Applying the torque wakes up the body, so active gets set to true if it was false before.
See also Body::torque and Body::applyTorque().
applyLinearImpulse(impulseVector, worldPoint) |
Applies an impulse with the direction of impulseVector at the worldPoint of the physics Body. The impulseVector is in world coordinates, so if you want to apply the impulse based on the current body rotation use Body::toWorldVector().
Consider this example:
BoxCollider { id: collider MouseArea { anchors.fill: parent onClicked: { var forwardVectorInBody = collider.body.toWorldVector(Qt.point(10, 0)); // apply the impulse from the world center, in the direction of movement collider.applyLinearImpulse(forwardVectorInBody, collider.body.getWorldCenter()); } } }
You can apply an impulse to let the physics object immediately react to it. So the difference to the Body::applyForce() function is, that a force is applied steadily, while an impulse is only a single shot.