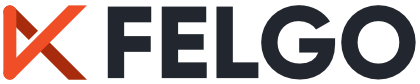
Provides often-needed functionality like generating a random number between 2 values. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
This component allows easy access to often-needed functionality like generating a random number between 2 values.
Other functions will follow based on customer requests, to simplify often-needed tasks that are not supported by default as dedicated functions in JavaScript.
Usually, you can use the functionality from the GameWindow::utils property, so you do not need to make more instances of this component as the utils
property is accessible globally in all QML files.
The following example shows how to call the Utils::generateRandomValueBetween() method from a TestEntity.qml file:
import Felgo EntityBase { Component.onCompleted: { // the utils property is available to all elements, because it is contained in the root GameWindow element console.debug("random value between 10 and 15:", utils.generateRandomValueBetween(10, 15) ) } }
clamp(num, minimum, maximum) |
Returns a number whose value is limited to the given range minimum and maximum.
For example, limit the output of this computation to between 0 and 255
var x = Math.random()*512 console.debug("Limit to [0,255]: ", utils.clamp(x, 0, 255) )
cropPath(path, cropExtension) |
Crops any input path and returns only the file name without path but with file ending. The second parameter specifies if the file extension should be cropped too.
console.debug("crop results in fireParticle.png : ", utils.cropPath(particles/fireParticle.png) ) console.debug("crop results in fireParticle : ", utils.cropPath(particles/fireParticle.png, true) )
decimals(number, scientific) |
Returns the number of decimals for a given number. By default scientific is false and a simple algorithm is used to count zeros after the decimal point, therefore only simple floats (0.003) can be used and no scientific numbers (0.003005 or .34e1). Enable the scientific flag to use scientific numbers.
The following code shows how it works.
import QtQuick import Felgo GameWindow { Scene { Component.onCompleted: { console.debug("2 has "+utils.decimals('2')+" decimals") // Answer: 0 console.debug(".3 has "+utils.decimals('.3')+" decimals") // Answer: 1 console.debug("0.2 has "+utils.decimals('.02')+" decimals") // Answer: 2 console.debug("0.2 has "+utils.decimals('.02002')+" decimals") // Answer: 2 console.debug("0.2 has "+utils.decimals('.02002',true)+" decimals") // Answer: 5 console.debug("34e-100 has "+utils.decimals('34e-100',true)+" decimals") // Answer: 100 console.debug("1.7e-99 has "+utils.decimals('1.7e-99',true)+" decimals") // Answer: 100 console.debug(".3e1 has "+utils.decimals('.3e1',true)+" decimals") // Answer: 0 console.debug(".34e1 has "+utils.decimals('.34e1',true)+" decimals") // Answer: 1 } } }
generateRandomValueBetween(minimum, maximum) |
Returns a floating point value between minimum and maximum.
For example the call generateRandomValueBetween(10, 15)
returns a random value between 10 and 15.
The minimum value can also be negative, e.g. generateRandomValueBetween(-40, 30)
returns a value between -40 and 30, including the possibility to return 0.
Internally, Math.random() is used for calculating the random number.
|
Returns a random color.
For example the call randomColor()
returns a random value between #FFFFFF and #000000.
This method was introduced in Felgo 2.0.2.