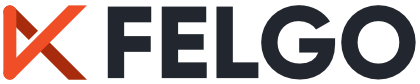
A single-line TextField input control. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 2.7.0 |
Inherits: |
AppTextField is an extension of the basic QML TextField for getting single-line text input from the user.
It implements a default platform-specific styling. Use the properties background, font and others to customize the appearance.
Input controls like AppTextInput or AppTextField, which extends the QML TextField type, own a placeholderText property, which you can use for your placeholder text.
import Felgo import QtQuick App { AppPage { // remove focus from textedit if background is clicked MouseArea { anchors.fill: parent onClicked: textEdit.focus = false } // background for input Rectangle { anchors.fill: textEdit anchors.margins: -dp(8) color: "lightgrey" } // input AppTextField { id: textEdit width: dp(200) placeholderText: "What's your name?" anchors.centerIn: parent } } }
The text input components provide predefined validators that you can use, they are called inputMethodHints.
You can also add custom validators to restrict the accepted input to a certain input type or expression. Input that does not match the validator is not accepted. To do custom validations and show errors for accepted input, you can add simple checks and control the visibility of errors with property bindings:
import Felgo import QtQuick App { // background for input Rectangle { anchors.fill: textInput anchors.margins: -dp(8) color: "lightgrey" } // input AppTextField { id: textInput width: dp(200) placeholderText: "What's your name?" anchors.centerIn: parent // only allow letters and check length validator: RegularExpressionValidator { regularExpression: /[A-Za-z]+/ } property bool isTooLong: textInput.text.length >= 6 } // show error if too long AppText { text: "Error: Use less than 6 letters." color: "red" anchors.top: textInput.bottom anchors.topMargin: dp(16) anchors.left: textInput.left visible: textInput.isTooLong } }
Find more examples for frequently asked development questions and important concepts in the following guides:
backgroundColor : color |
The background color of the text field. If the background is transparent and the text field's borderWidth is 0
, the control uses a native look on
Android.
The default value for Android is transparent
, otherwise it is Theme::backgroundColor.
borderColor : color |
The color of the text field's border.
The default value is Theme::dividerColor.
borderWidth : real |
The width of the text field's border. If the text field has no border and the backgroundColor is transparent, the control uses a native look on Android.
The default value is 0
.
[since Felgo 3.9.1] clearButton : alias |
An alias to the IconButton that removes the current input text.
This property was introduced in Felgo 3.9.1.
See also showClearButton.
[since Felgo 2.7.0] clearsOnBeginEditing : bool |
If set to true
, the text field's current text is cleared when the user selects the text field to begin editing. If false
, the text field places an insertion point at the place where the user
tapped the field.
The default value is false
.
This property was introduced in Felgo 2.7.0.
[since Felgo 3.3.0] clickEnabled : bool |
This property is used for text fields without manual input. When the user selects this text field, the clicked signal is fired instead of setting focus to the text field. This makes sense e.g. for date input fields, where you can show a date picker when the field is selected.
import QtQuick import Felgo App { NavigationStack { AppPage { title: "AppTextField::clickEnabled" AppTextField { id: textField width: parent.width placeholderText: "Select date" clickEnabled: true onClicked: { NativeUtils.displayDatePicker() } Connections { target: NativeUtils onDatePickerFinished: { if(accepted) textField.text = date } } } } } }
The default value is false
.
This property was introduced in Felgo 3.3.0.
[since Felgo 2.7.0] cursorColor : color |
The color of the cursor that marks the current position in the text field. Matches Theme::inputCursorColor by default.
This property was introduced in Felgo 2.7.0.
[since Felgo 3.3.0] inputMode : int |
You can use a set of predefined input modes for different types of text fields:
import QtQuick import Felgo App { NavigationStack { AppPage { title: "AppTextField::inputMode" AppTextField { width: parent.width inputMode: inputModeEmail } } } }
The default value is inputModeDefault
.
This property was introduced in Felgo 3.3.0.
[since Felgo 3.3.0] passwordVisible : bool |
Set this to display the password instead of showing disc characters. This only has an effect if the text field is used with inputModePassword.
This property is also used by the show password button, which can be enabled/disabled using showPasswordVisibleButton.
The default value is false
.
This property was introduced in Felgo 3.3.0.
[since Felgo 3.9.1] passwordVisibleButton : alias |
An alias to the IconButton that toggles password visibility when in inputModePassword.
This property was introduced in Felgo 3.9.1.
See also showPasswordVisibleButton.
placeholderColor : color |
The color for the placeholder text that is displayed when the field is empty.
The default value is Theme::placeholderTextColor.
radius : real |
The Rectangle::radius of the background.
The default value is 0
.
[since Felgo 2.7.0] showClearButton : bool |
If set to true
, a clear button is displayed at the right side of the text field as soon as the text field has contents, as a way for the user to remove the current text.
The default value is false
.
This property was introduced in Felgo 2.7.0.
[since Felgo 3.3.0] showPasswordVisibleButton : bool |
This property is used to show a button to display the password instead of showing disc characters.
The default value is true
if the text field is used with inputModePassword, else false
.
This property was introduced in Felgo 3.3.0.
textColor : color |
An alias to the color property of the inherited TextField.
The default value is Theme.colors.textColor.
[since Felgo 3.7.0] underlineColor : color |
The color of the text field underline for Android. By default matches Theme::inputCursorColor on focus and Theme::placeholderTextColor otherwise.
This property was introduced in Felgo 3.7.0.
|
This signal is fired if the text field uses clickEnabled and is selected.
Note: The corresponding handler is onClicked
.
This signal was introduced in Felgo 3.3.0.