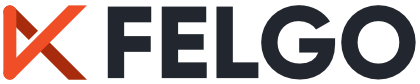
iOS | Android |
---|---|
|
|
This is a list of common widgets and controls available with Felgo. Many of those components are also used in examples in the documentation topics on the left. In addition to the Felgo controls, you can also use the Qt Quick Controls and apply custom styling.
An intermediate spinning progress indicator |
|
A button with a default raised and flat button style |
|
A material style card |
|
Adds Tinder-like swipe feature to a component |
|
A checkbox with a platform-specific styling for iOS and Android |
|
An item for displaying icons from an icon font |
|
An image with a default source |
|
Basic item for any type of lists, with text, images, icons etc |
|
A ListView providing a native AppScrollIndicator, an empty view and swipe gestures for its list delegates |
|
Displays a map view with the current user position |
|
A modal dialog that covers the whole application |
|
Displays a custom component as a modal overlay |
|
A sheet of paper with shadow |
|
A radio with a platform-specific styling for iOS and Android |
|
A slider control with two handles to set a range |
|
A native styled scroll indicator for usage with AppFlickable |
|
A slider control with one handle |
|
An on/off button-like control |
|
A tab bar with Theme-based iOS and Android styles |
|
A tab button to be used with AppTabBar for Theme-based iOS and Android styled tabs |
|
A styled text control |
|
A multi-line TextEdit with a given placeholder text |
|
A single-line TextField input control |
|
A single-line TextInput with a given placeholder text (deprecated) |
|
Shows a tool tip overlay for a target item |
|
Allows to pick a date, time or interval with flickable tumblers |
|
A dialog with custom content and one or two buttons |
|
A material-design styled floating action button |
|
A button with an icon as visual representation |
|
A GridView to show and select multiple photos from the device |
|
Global object for displaying standard user interaction dialogs |
|
Global object for invoking native system dialogs |
|
Displays page indicators and allows switching to the previous or next page |
|
A modal, full-screen picture viewer component |
|
A helper adding a pull-to-refresh control to an AppListView |
|
An image item with rounded corners and an optional border |
|
A search bar with native styling for iOS and Android based on the Theme settings |
|
A control that allows to jump to specific sections of a ListView |
|
A ListView delegate item with two labels and an optional image |
|
A ListView delegate item for displaying sections in a list view |
|
A button with a default style to be used together with SwipeOptionsContainer |
|
A ListView delegate container to implement swipeable rows within a ListView |
|
A row with a label and an AppTextField |
|
A helper item for handling data reload actions as soon as the item becomes visible |
The component documentations also contain examples like this:
import Felgo App { NavigationStack { AppPage { title: "Icons" // Felgo contains the FontAwesome icon font to display icons AppIcon { anchors.centerIn: parent iconType: IconType.heart } } } }
The NativeUtils component provides additional convenient access to several native device features and widgets.
Below is a list if the most important methods from NativeUtils:
displayAlertDialog | Displays a native alert dialog with a given title, an optional description that can provide more details, an OK button and an optional Cancel button. |
displayAlertSheet | Displays a modal alert sheet with the specific options. It uses an AlertDialog on Android and a UIActionSheet on iOS. |
displayCameraPicker | Allows to take a photo by starting the native camera, if available. |
displayDatePicker | Allows to choose a date from a calendar by displaying a native date picker dialog, if available. |
displayImagePicker | Allows to choose a photo from the device by displaying the native image picker, if available. |
displayMessageBox | Displays a native-looking message box dialog with a given title, an optional description that can provide more details, an OK button and an optional Cancel button. |
displayTextInput | Displays a native-looking message input dialog with a given title, a description that can provide more details, a placeholder that is displayed as long as the input field is empty (optional) and a prefilled text. |
getContacts | Returns a list of all contacts including name and phone number. |
openApp | Tries to launch an app identified by launchParam on the device. The required parameter value depends on the platform. |
openUrl | Opens the urlString with the default application associated with the given URL protocol of the current platform. |
sendEmail | Opens the native email app prefilled with the given to receiver, subject and message. |
share | Opens the native share dialog with a given text and url. |
storeContacts | Stores one or more contacts to the device address book. |
Each .qml
file of your project is an own reusable QML Component. The specified root QML Item determines the base component, which your new item extends. The new QML type can then add properties, signals and
functions, which form the public interface of the component.
To define the layout for the item, add some child elements and position them in the view. Each child element may also be extended with new features and properties directly where it is used. You do not require to create an own QML Item to make small additions to a single item instance in your UI.
The following example shows a clickable CustomButton.qml
component with a background and text. Once imported, you can use the new type in your app:
import Felgo import "relative-path-to-directory-of-custom-button" App { CustomButton { anchors.centerIn: parent // new count property only for this button instance property int count: 0 text: "You clicked "+count+" times." backgroundColor: "yellow" onClicked: count++ } }
import QtQuick // CustomButton extends Item, which is the base type for all UI elements Item { // the default (implicit) size is based on the size of the child text element // the width and height thus depend on the actual text value implicitWidth: textItem.implicitWidth implicitHeight: textItem.implicitHeight // public properties and signals property color backgroundColor: "green" property string text: "Click Me!" signal clicked() // child elements Rectangle { anchors.fill: parent color: parent.backgroundColor } Text { id: textItem // id for referencing this text item within the component text: parent.text anchors.centerIn: parent } // handle touch/click and fire signal when a click happens MouseArea { anchors.fill: parent onClicked: parent.clicked() // emits clicked signal of CustomButton } }
Find more examples for frequently asked development questions and important concepts in the following guides: