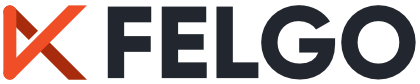
Felgo and Qt provide you with tools to connect to basically anything, like services, servers and other devices.
We have a dedicated doc for this use-case, you can find it here: Access a REST Service
It also includes how to read and parse JSON data.
The easiest way to work with XML data is by using the XmlListModel type. After we set the XML source and add some queries to identify the items and item attributes, we can directly use this model to display the items with components like AppListView or Repeater.
The following example sets up a XmlListModel to load data from a local XML file and displays the items in a ListPage:
data.xml
<?xml version="1.0" encoding="UTF-8" ?> <data> <item>Item 1</item> <item>Item 2</item> <item>Item 3</item> <item>Item 4</item> <item>Item 5</item> <item>Item 6</item> <item>Item 7</item> <item>Item 8</item> <item>Item 9</item> </data>
Main.qml
import Felgo import QtQuick.XmlListModel App { // model for loading and parsing xml data XmlListModel { id: xmlModel // set xml source to load data from local file or web service source: Qt.resolvedUrl("data.xml") // set query that returns items query: "/data/item" // specify roles to access item data XmlRole { name: "itemText"; query: "string()" } } NavigationStack { // we display the xml model in a list ListPage { id: page title: "Parse XML" model: xmlModel delegate: SimpleRow { text: itemText } } } }
On macOS, iOS and Android, you can discover devices and services in the local network via Bonjour/ZeroConf.
These protocols allow devices to broadcast active network services. You can obtain information about the service type, IP addresses and port, and other attributes.
You can use ZeroConf::startNetworkServiceDiscovery() to start searching for services in the active network.
See ZeroConf for an example.
You can use the full range of Qt Connectivity and Networking features with Felgo.
This includes:
As Felgo relies on QML and Javascript, updates to the UI are already handled in a highly asynchronous and event-driven way. You weave-in your Javascript code as part of signal handlers that execute when certain events or user interactions happen. The QML rendering engine is highly optimized, so you do not need to worry about blocking the UI when adding your view logic.
Communication with your application backend is also easy, as you can use the HttpRequest Element asynchronously. The following example fetches a JSON response containing the URL to an image. The request happens asynchronously. The returned URL is then set as the AppImage source, which is able to asynchronously load an image from an URL:
import Felgo import QtQuick App { property string serverUrl: "https://jsonplaceholder.typicode.com/photos/1" property var jsonData: undefined // handler function to be executed when the App Item is fully created, starts web requests Component.onCompleted: { // the 3rd parameter of open(...) is the asynchronous flag var request = HttpRequest .get(serverUrl) .then(function(res) { jsonData = res.body // keep JSON result }) .catch(function(err) { console.log(err.message) console.log(err.response.text) }); } AppPage { // just some spinning icon to show asynchronous loading of image AppIcon { anchors.centerIn: parent iconType: IconType.refresh NumberAnimation on rotation { loops: Animation.Infinite from: 0 to: 360 duration: 1000 } } AppImage { // expression for source relies on the jsonData property (property binding!) // the REST api returns the web url to an image, which we can set directly to load the image source: jsonData !== undefined ? jsonData.url : "" anchors.fill: parent fillMode: AppImage.PreserveAspectFit // additionally you can make the image loading itself asynchronous // this is recommended for images loaded from web source asynchronous: true } } }
After request completion, the result is stored to the jsonData
property. The expression for the image source relies on the property, so the AppImage will automatically update
and show the image as soon as the data arrives - nothing more to do.
Find more examples for frequently asked development questions and important concepts in the following guides: