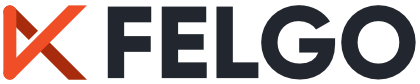
Felgo offers an extensive animation framework with the Animation QML components. The following examples show some possible uses.
You can create a spinner by animating the rotation of an item. With infinite loops, it becomes an endless spinner.
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Spinner" AppText { id: label anchors.centerIn: parent text: "Weeeeeeeee" } NumberAnimation { running: true // Start automatically loops: Animation.Infinite // Repeat endless (unless stopped) target: label // The animated item id property: "rotation" // The animated property from: 0 // Start value. If not defined, the current value is used to: 360 // End value duration: 1000 // Duration of the animation } } } }
You can trigger an animation with a button click by calling its start() function.
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Triggered Animation" AppButton { anchors.horizontalCenter: parent.horizontalCenter text: "Spin" flat: false // The button is disabled while the animation is running enabled: !animation.running onClicked: { // We start the animation after a button click animation.start() } } AppText { id: label anchors.centerIn: parent // The text changes while the animation is running text: animation.running? "Weeeeeeeee" : "Spin me!" } NumberAnimation { id: animation target: label // The animated item id property: "rotation" // The animated property from: 0 // Start value. If not defined, the current value is used to: 360 // End value duration: 1000 // Duration of the animation } } } }
The create more juicy animations, you can apply easing functions. We take the example from above and make it more fancy with easing.
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Animation with Easing" AppButton { anchors.horizontalCenter: parent.horizontalCenter text: "Spin" flat: false // The button is disabled while the animation is running enabled: !animation.running onClicked: { // We start the animation after a button click animation.start() } } AppText { id: label anchors.centerIn: parent // The text changes while the animation is running text: animation.running? "Weeeeeeeee" : "Spin me!" } NumberAnimation { id: animation target: label // The animated item id property: "rotation" // The animated property from: 0 // Start value. If not defined, the current value is used to: 360 // End value duration: 1000 // Duration of the animation easing.type: Easing.InOutBack // Use an easing function for this animation } } } }
Some properties do not have a numeric value, like for example the color property of a Rectangle. In such cases, there are distinct animation types to smoothly animate those properties.
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Color Animation" AppButton { anchors.horizontalCenter: parent.horizontalCenter text: "Colorize" flat: false onClicked: { animation.start() } } Rectangle { id: rectangle anchors.centerIn: parent width: dp(200) height: dp(200) color: "#ff0000" // Beautiful red color } ColorAnimation { id: animation target: rectangle // The animated item id property: "color" // The animated property to: "#0000ff" // Beautiful blue color duration: 1000 // Duration of the animation } } } }
You can create complex animations by nesting animations. For this you can use the SequentialAnimation and ParallelAnimation types. You can combine those types in any order
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Complex Animation" AppButton { anchors.centerIn: parent text: "Move!" flat: false onClicked: { // Start the animation by calling start() of the top-most item complexAnimation.start() } } Rectangle { id: rectangle width: dp(100) height: dp(100) color: "black" } // All child animations will be executed one after the other SequentialAnimation { // You can start the whole animation by calling start() of the top-most item id: complexAnimation // 1. Move to right NumberAnimation { target: rectangle property: "x" to: dp(200) duration: 1000 } // 2. Move down and change color // All child animations will be executed in parallel ParallelAnimation { NumberAnimation { target: rectangle property: "y" to: dp(400) duration: 1000 easing.type: Easing.InOutQuad } ColorAnimation { target: rectangle property: "color" to: "blue" duration: 1000 } } // 3. Move left, change color and rotate // All child animations will be executed in parallel ParallelAnimation { NumberAnimation { target: rectangle property: "x" to: 0 duration: 1000 } ColorAnimation { target: rectangle property: "color" to: "black" duration: 1000 } NumberAnimation { target: rectangle property: "rotation" from: 0 to: 360 duration: 1000 } } // 4. Move up NumberAnimation { target: rectangle property: "y" to: 0 duration: 1000 easing.type: Easing.InBack } } } } }
Often you want to execute an action after an animation stops. We prepared to examples how you could do so.
import Felgo import QtQuick App { NavigationStack { AppPage { title: "Triggered Animation" Column { anchors.centerIn: parent AppButton { anchors.horizontalCenter: parent.horizontalCenter text: "Animation" flat: false enabled: !animation.running onClicked: { animationLog.text += "\nAnimation started" animation.start() } } AppButton { anchors.horizontalCenter: parent.horizontalCenter text: "SequentialAnimation" flat: false enabled: !sequentialAnimation.running onClicked: { animationLog.text += "\nSequentialAnimation started" sequentialAnimation.start() } } } AppText { id: animationLog } // The animations don't actually animate anything this example, but still they will run NumberAnimation { id: animation duration: 1000 onStopped: { animationLog.text += "\nAnimation stopped" } } SequentialAnimation { id: sequentialAnimation NumberAnimation { duration: 1000 } ScriptAction { script: { animationLog.text += "\nSequentialAnimation stopped" } } } } } }
It is possible to animate every basic property of your UI elements. You can also define behaviors to automatically animate properties whenever they change.
The following example allows to fade a text by animating its opacity:
import Felgo import QtQuick App { AppPage { // button at the bottom allows to toggle the text AppButton { anchors.horizontalCenter: parent.horizontalCenter anchors.bottom: parent.bottom text: "Toggle Text Item" onClicked: textItem.opacity = textItem.visible ? 0 : 1 // toggle textItem visibility } // centered text which fades when opacity changes AppText { id: textItem anchors.centerIn: parent text: "Hello World!" visible: opacity != 0 // also set invisible when fully transparent // when opacity changes ... Behavior on opacity { NumberAnimation { duration: 500 } // ... animate to reach new value within 500ms } } } }
Find more examples for frequently asked development questions and important concepts in the following guides: