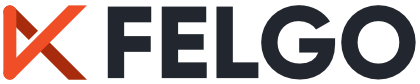
In order to store data persistent between app runs, you can make use of different database features.
You can store simple key/value pairs using the App::settings property. If you format the value with JSON, you can also use this to store more complex data in a very easy way.
import Felgo import QtQuick App { id: app // this property holds how often the app was started property int numberAppStarts Component.onCompleted: { // this code reads the numberAppStarts value from the database // getValue() returns undefined, if no setting for this key is found, so when this is the first start of the app var tempNumberAppStarts = app.settings.getValue("numberAppStarts") if(tempNumberAppStarts === undefined) tempNumberAppStarts = 1 else tempNumberAppStarts++ app.settings.setValue("numberAppStarts", tempNumberAppStarts) numberAppStarts = tempNumberAppStarts } NavigationStack { AppPage { title: "Settings" AppText { anchors.centerIn: parent text: "App starts: " + numberAppStarts } } } }
This Storage component is used internally for the App settings from the example above. You can also use it as standalone component.
import Felgo import QtQuick App { id: app // this property holds how often the app was started property int numberAppStarts Component.onCompleted: { // this code reads the numberAppStarts value from the database // getValue() returns undefined, if no setting for this key is found, so when this is the first start of the app var tempNumberAppStarts = storage.getValue("numberAppStarts") if(tempNumberAppStarts === undefined) tempNumberAppStarts = 1 else tempNumberAppStarts++ storage.setValue("numberAppStarts", tempNumberAppStarts) numberAppStarts = tempNumberAppStarts } Storage { id: storage } NavigationStack { AppPage { title: "Settings" AppText { anchors.centerIn: parent text: "App starts: " + numberAppStarts } } } }
This Settings component pretty similar to the App settings, however the usage is a bit different. It uses the defined properties as keys, to store and retrieve the values.
On iOS and Android, you can use the native Keychain to store, read and delete data securely and persistently.
On iOS, each stored value is handled as a separate keychain item representing a generic password. On Android, each value is persisted in SharedPreferences
with AES-256 encryption. The automatically generated
encryption key is securely stored via the Android KeyStore system.
Here is an example how to use the Keychain storage functionality using the Felgo NativeUtils:
import Felgo import QtQuick App { AppButton { text: "Store in Keychain" onClicked: NativeUtils.setKeychainValue("identifier", "value") } AppButton { text: "Read Keychain" onClicked: NativeUtils.getKeychainValue("identifier") } AppButton { text: "Delete from Keychain" onClicked: NativeUtils.clearKeychainValue("identifier") } }
The Firebase plugin allows to use the Firebase backend services. FirebaseAuth provides e-mail authentication to create user accounts, log in and log out. You can access the FirebaseDatabase and store and retrieve user-specific data with and without authentication. Use FirebaseStorage to upload binary files to the cloud.
This example shows how to read and write public data with the FirebaseDatabase item. If you want only authenticated users to access your database, have a look at the Private Database Example
import Felgo import QtQuick App { FirebaseDatabase { id: firebaseDb // we store the read values in those properties to display them in our text items property string testValue: "" property string realtimeTestValue: "" // define which values should be read in realtime realtimeValueKeys: ["public/testValue"] // update our local realtimeTestValue property if database value changed onRealtimeValueChanged: (success, key, value) => { if(success && key === "testValue") { realtimeTestValue = value } } // update our local testValue property if read from database was successful onReadCompleted: (success, key, value) => { if(success) { console.debug("Read value " + value + " for key " + key) testValue = value } else { console.debug("Error: " + value) } } // add some debug output to check if write was successful onWriteCompleted: (success, key, value) => { if(success) { console.debug("Successfully wrote to DB") } else { console.debug("Write failed with error: " + message) } } } NavigationStack { AppPage { title: "Realtime Database" // our simple UI Column { anchors.fill: parent spacing: dp(15) // button to update public/testValue with test-[currenttimestamp] AppButton { text: "Update Value" anchors.horizontalCenter: parent.horizontalCenter onClicked: firebaseDb.setValue("public/testValue", "test-" + Date.now()) } // button to read the public/testValue manually AppButton { text: "Get Value" anchors.horizontalCenter: parent.horizontalCenter onClicked: firebaseDb.getValue("public/testValue") } // display the testValue property with a simple property binding AppText { width: parent.width horizontalAlignment: AppText.AlignHCenter text: "Manual TestValue: " + firebaseDb.testValue } // display the realtimeTestValue property with a simple property binding AppText { width: parent.width horizontalAlignment: AppText.AlignHCenter text: "Realtime TestValue: " + firebaseDb.realtimeTestValue } }// Column }// Page }// NavigationStack }// App
You can access a local SQLite Database using the Qt Quick Local Storage QML Types.
For more details on this, please go to Access, Store and Share Files.
Find more examples for frequently asked development questions and important concepts in the following guides: