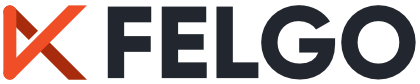
The AdMobRewardedVideo item allows monetizing your app with rewarded video ads on Android and iOS. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 2.13.0 |
Inherits: |
Rewarded videos are skippable full-screen videos shown to the user.
You can reward the user for watching a full video until the end. You can choose how this reward looks like: give away some virtual currency, virtual items or unlock features within your app or game are popular examples.
Note: In contrast to interstitials, it is not possible to have more than one AdmobRewardedVideo item in your code. This restriction comes from the native AdMob SDK for iOS and Android.
Here is an example how you can reward the user with virtual currency after he watched a rewarded video ad:
import Felgo import QtQuick App { id: app // start currency property int token: 20 NavigationStack { AppPage { title: "Admob" // we show the token in the navigation bar rightBarItem: NavigationBarItem { AppText { anchors.verticalCenter: parent.verticalCenter text: app.token color: Theme.isIos ? Theme.tintColor : "white" font.bold: true } } AdMobRewardedVideo { id: myRewardedVideo // test ad for rewarded videos // create your own from the AdMob Backend adUnitId: system.isPlatform(System.IOS) ? "ca-app-pub-3940256099942544/1712485313" : "ca-app-pub-3940256099942544/5224354917" // if the video is fully watched, we add an amount of tokens configured in the backend onRewardedVideoRewarded: (type, amount) => { app.token += 10 } onRewardedVideoClosed: { // load a new video every time it got shown, to give the user a fresh ad loadRewardedVideo() } // load interstitial at app start to cache it onPluginLoaded: { loadRewardedVideo() } } AppButton { anchors.centerIn: parent text: "Token--" onClicked: { // decrease token by 1 app.token-- if(app.token < 10) { // show the new video if user is below 10 token myRewardedVideo.showRewardedVideoIfLoaded() } } } } } }
With this example code, you can motivate users to watch an ad because they get something for it. Those users who do not want to watch an ad, can also purchase a virtual currency with the above code example. By only showing the ad if the user is below a certain virtual currency threshold, you guarantee users who bought a virtual currency pack do not see the ad.
Rewarded ads are the preferred way to use mobile ads these days, so we highly recommend to use this feature in your apps. The perfect time to show a rewarded ad is when a user navigates between pages of your app. For example after he finished a game or after he had a success moment like completing a level. You could motivate the user even more to watch an ad by giving extra benefits after such a success moment. This helps you to earn money, while on the other hand giving a benefit to the user - a win-win situation.
For more information on the other available ad types and how to add this component to your app, see the AdMob Plugin.
[since Felgo 2.13.0] adUnitId : string |
Provide your Ad unit ID retrieved from the AdMob console in the format ca-app-pub-xxxxxxxxxxxxxxxx/xxxxxxxxxx
here. If you do not have an AdMob account yet, you can create
a new one at http://www.google.com/ads/admob/. Once you are logged in, you can acquire a new Ad unit ID by creating a new app and defining a new ad unit.
This property was introduced in Felgo 2.13.0.
[since Felgo 2.13.0] childDirectedTreatment : bool |
Set this property to true
if you want to indicate that you want Google to treat your content as child-directed. Google will then take steps to disable IBA and remarketing ads for your ads.
More information can also be found here.
This property was introduced in Felgo 2.13.0.
[since Felgo 3.9.0] requestAdTrackingAuthorization : bool |
Set this property to true
to automatically request ad tracking authorization on iOS.
The item will ask the user for permission to use the advertising identifier (IDFA) before showing any ad. If the user accepts, ads can be attribute to them better.
The item still shows the ad even if the user denies the request.
Note: This property only has an effect on iOS versions 14 and above. Earlier iOS versions and Android do not require the authorization.
To support the ad tracking authorization, adapt your ios/Project-Info.plist
. Add the NSUserTrackingUsageDescription
key with a description of usage. Example:
<key>NSUserTrackingUsageDescription</key> <string>This identifier will be used to deliver personalized ads to you.</string>
You can find more information here.
Note: This property only has an effect on iOS and is ignored on other platforms.
This property was introduced in Felgo 3.9.0.
Provide your device id as an array of string values here to receive test ads for the specific test devices.
Note: If you test your application with live ads frequently, Google might ban your account for fraud. Therefore if you are not able to provide the testDeviceIds and test with live ads instead.
You can find your testdeviceIds in the device log after you first run an your app with integrated AdMob plugin, search for "To get test ads on this device, call adRequest.addTestDevice".
This property was introduced in Felgo 2.13.0.
|
This handler is called when a rewarded video is dismissed by the user, either while it was still playing or has already finished. If the user watched the video until the end, rewardedVideoRewarded will also be called.
Note: The corresponding handler is onRewardedVideoClosed
.
This signal was introduced in Felgo 2.13.0.
See also rewardedVideoRewarded, loadRewardedVideo(), and showRewardedVideoIfLoaded().
|
This handler is called if a rewarded video can not be loaded, e.g. due to a network error.
Note: The corresponding handler is onRewardedVideoFailedToReceive
.
This signal was introduced in Felgo 2.13.0.
See also loadRewardedVideo() and showRewardedVideoIfLoaded().
|
This handler is called after the user clicked an ad while the video was playing and the app is going to be moved to the background to display the ad, e.g. in a browser.
Note: The corresponding handler is onRewardedVideoLeftApplication
.
This signal was introduced in Felgo 2.13.0.
See also loadRewardedVideo() and showRewardedVideoIfLoaded().
|
This handler is called when a rewarded video ad is displayed. At this point in time, the video might still be loading, rewardedVideoStarted will be emitted when it actually starts playing.
Note: The corresponding handler is onRewardedVideoOpened
.
This signal was introduced in Felgo 2.13.0.
See also rewardedVideoStarted, loadRewardedVideo(), and showRewardedVideoIfLoaded().
|
This handler is called after the loadRewardedVideo() request has finished and the rewarded video is ready to display.
Note: The corresponding handler is onRewardedVideoReceived
.
This signal was introduced in Felgo 2.13.0.
See also loadRewardedVideo() and showRewardedVideoIfLoaded().
This handler is called when the user finished watching the video and can be rewarded by the app.
The parameters type and amount contain information about the reward you can give your user.
Note: The corresponding handler is onRewardedVideoRewarded
.
This signal was introduced in Felgo 2.13.0.
See also rewardedVideoClosed, loadRewardedVideo(), and showRewardedVideoIfLoaded().
|
This handler is called when a rewarded video has started playing.
Note: The corresponding handler is onRewardedVideoStarted
.
This signal was introduced in Felgo 2.13.0.
See also rewardedVideoOpened, loadRewardedVideo(), and showRewardedVideoIfLoaded().
|
Call this method to start downloading a rewarded video ad in the background. When finished, the rewardedVideoReceived signal will be emitted.
This method was introduced in Felgo 2.13.0.
See also rewardedVideoReceived and showRewardedVideoIfLoaded().
|
This method displays a rewarded video ad that was previously requested via a call to the loadRewardedVideo() method.
For example, it can be called directly after rewardedVideoReceived is emitted:
import Felgo App { NavigationStack { AppPage { title: "Admob Rewarded Video" AdMobRewardedVideo { adUnitId: system.isPlatform(System.IOS) ? "ca-app-pub-3940256099942544/1712485313" : "ca-app-pub-3940256099942544/5224354917" // rewarded video test ad by AdMob testDeviceIds: [ "" ] onRewardedVideoReceived: { showRewardedVideoIfLoaded() } onPluginLoaded: { loadRewardedVideo() } } } } }
Note: After showing a rewarded video ad, it is unloaded again. Call loadRewardedVideo() to load another one.
This method was introduced in Felgo 2.13.0.
See also loadRewardedVideo() and rewardedVideoReceived.