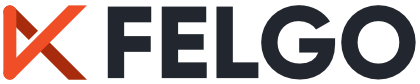
Bluetooth Low Energy characteristics handle data read and write. More...
Import Statement: | import Felgo 4.0 |
Since: | Felgo 3.7.0 |
General info and usage examples for Bluetooth LE: Use Bluetooth Low Energy
Bluetooth Low Energy characteristics are the actual logical input and output nodes for data transfer. The BluetoothLeCharacteristic provides a simple way to describe, read and write characteristics. Notifications are set automatically so value updates trigger QML signals and bindigs.
Declaring Characteristics in QML ahead of time allows the definition of helper functions for formating, data parsing, and handling signals.
This is an example for a read write string in a custom characteristic.
BluetoothLeCharacteristic { id: stringCharacteristic uuid: '{beb5483e-36e1-4688-b7f5-ea07361b26a8}' dataFormat: 0x19 function formatWrite(text) { let data = new Uint8Array(text.length) for (var i = 0; i < text.length; i++) { data[i] = text.charCodeAt(i) } write(data.buffer) } onValidChanged: { // Read initial value once characteristic is valid if (valid) { read() } } }
CharacteristicReadWriteError : enumeration |
Possible error values for characteristic read or write. For example if a the property PropertyType.Read
is not set, the readWriteError
signal will be emitted with the value CharacteristicReadWriteError.ReadNotAllowed
.
CharacteristicReadWriteError.NoError
CharacteristicReadWriteError.ReadNotAllowed
CharacteristicReadWriteError.WriteNotAllowed
PropertyType : enumeration |
Characteristic property definitions, properties describe operation permissions for the containing characteristic.
PropertyType.Unknown
PropertyType.Broadcasting
PropertyType.Read
PropertyType.WriteNoResponse
PropertyType.Write
PropertyType.Notify
PropertyType.Indicate
PropertyType.WriteSigned
PropertyType.ExtendedProperty
See also BluetoothLeCharacteristic::properties and QLowEnergyCharacteristic::PropertyType.
dataFormat : int |
Some characteristics provide the Characteristic Presentation Format descriptor that carries the format that the value uses, in most cases is missing and should be provided via a XML BluetoothLE profile. It can also be set
here as an int value. If a dataFormat value is set, an automatic convertion is used for unsigned and string values, providing the result in the value property. The default value is -1
.
Some common formats are:
descriptors : var |
A list of objects containing the descriptors values for the characteristic, each descriptor contains a string name, a string uuid and a var value.
name : string |
Name of the characteristic reported by the remote device, if the device does report and empty name it's possible to set this value with the fallback name.
properties : BluetoothLeCharacteristic::PropertyTypes |
Holds the property flags for the characteristic.
This is a test of the characteristic read permission.
BluetoothLeCharecteristic { Component.onCompleted: { var canRead = properties.indexOf(BluetoothLeCharecteristic.Read) > -1; } }
Note: If the characteristic descriptors contain the PropertyType.Notify
, the BluetoothLeDevice sends automatically a descriptor write to enable
notifications. Value updates from the remote device will trigger QML bindings to the corresponding characteristic value.
uuid : var |
Reading the uuid will always return the long 128bit string formated value as "{xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx}" where 'x' is a hex digit. A short 16bit uuid can be set using the 0xXXXX notation for standard characteristics defined by the Bluetooth SIG.
valid : bool |
Set if a valid characteristic is discovered for the current uuid.
value : var |
The value of the characteristic parsed using the dataFormat provided. This property returns undefined if the dataFormat property is not set.
valueBase64 : string |
A base64 encoded string of the raw binary data value.
valueRaw : ArrayBuffer |
The raw binary value as an ArrayBuffer.
A read or write error was emitted durint read or write. For example trying to read a characteristic that does not allow reading.
Note: The corresponding handler is onReadWriteError
.
void read() |
Requests a characteristic read, the characteristic value is updated if the read is successful, otherwise a readWriteError signal will be emitted.
void write(ArrayBuffer data, QLowEnergyService::WriteMode writeMode = QLowEnergyService::WriteWithResponse) |
Starts a characteristic write with the given data. If the remote device has notifications enabled, the value is updated as soon as the device receives the write request. You can also request a read after writing to the characteristic, as long as the characteristic has a property allowing the writeMode.