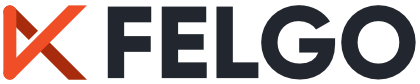
Enables to follow an object, or be moved around freely. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
The camera is a useful component for a variety of games like platformers, side scroller or any games with bigger level size than one screen. It enables you to automatically follow an object as it moves through your game world, or it can be moved around freely.
It supports zooming with Mouse wheel on Desktop and finger pinch gesture on mobile. Zooming is possible in both following and free-move mode.
It also support to limit the visible area, to avoid scrolling outside your visible level border.
In free move mode, the camera is decelerated to have a fluent and natural animation, similar to popular games like Angry Birds.
All you need to do, is to set the gameWindowSize and the entityContainer property for your game. If you set the focusedObject property, the camera follows the object, otherwise it is freely movable.
In this example the camera can be moved around freely by clicking and dragging with the mouse. Change the zoom via the mouse wheel (desktop) or the pinch gesture (mobile devices).
import Felgo import QtQuick GameWindow { activeScene: gameScene EntityManager { id: entityManager entityContainer: gameScene.container } Scene { id: gameScene // set scene alignment sceneAlignmentX: "left" sceneAlignmentY: "top" Camera { id: camera // set the gameWindowSize and entityContainer required for the camera gameWindowSize: Qt.point(gameScene.gameWindowAnchorItem.width, gameScene.gameWindowAnchorItem.height) entityContainer: container // if you don't set the focusedObject property, or set it to null, the camera is freely movable focusedObject: null // set the camera's limits limitLeft: 0 limitRight: 600 limitTop: 0 limitBottom: 400 } Item { id: container // 5 green rectangles to make the player's movement visible Rectangle { x: 0; y: 0 width: 32; height: 32 color: "green" } Rectangle { x: 568; y: 368 width: 32; height: 32 color: "green" } Rectangle { x: 568; y: 0 width: 32; height: 32 color: "green" } Rectangle { x: 0; y: 368 width: 32; height: 32 color: "green" } Rectangle { x: 284; y: 184 width: 32; height: 32 color: "green" } } } }
In the following example the Camera is used to follow an object. You can move the object via the arrow keys.
import Felgo import QtQuick GameWindow { activeScene: gameScene EntityManager { id: entityManager entityContainer: gameScene.container } Scene { id: gameScene // set scene alignment sceneAlignmentX: "left" sceneAlignmentY: "top" // forward the keyboard input to the TwoAxisController Keys.forwardTo: controller Item { id: container PhysicsWorld { debugDrawVisible: false } // the controllable entity EntityBase { id: player x: 100; y: 100 width: 32; height: 32 Rectangle { anchors.fill: parent color: "blue" } // this controller helps to move the player TwoAxisController { id: controller } BoxCollider { anchors.fill: parent // set the force, depending on which arrow keys are pressed force: Qt.point(controller.xAxis * 2000, -controller.yAxis * 2000) // dampen the movement linearDamping: 15 } } // 5 green rectangles to make the player's movement visible Rectangle { x: 0; y: 0 width: 32; height: 32 color: "green" } Rectangle { x: 668; y: 368 width: 32; height: 32 color: "green" } Rectangle { x: 668; y: 0 width: 32; height: 32 color: "green" } Rectangle { x: 0; y: 368 width: 32; height: 32 color: "green" } Rectangle { x: 334; y: 184 width: 32; height: 32 color: "green" } } Camera { id: camera // set the gameWindowSize and entityContainer required for the camera gameWindowSize: Qt.point(gameScene.gameWindowAnchorItem.width, gameScene.gameWindowAnchorItem.height) entityContainer: container // the camera follows the player focusedObject: player // set the camera's limits limitLeft: 0 limitRight: 700 limitTop: 0 limitBottom: 400 } } }
allowZoomWithFocus : bool |
With this property you can enable the pinch to zeeom gesture even when an object is focused. The default value is false.
applyZoomThreshold : real |
entityContainer : Item |
This property holds the container, in which all entities are in.
focusOffset : point |
This property holds the relative screen position of the focusedObject. Set each coordinate to a real value between 0.0 and 1.0. By default, both are 0.5, so the focusedObject is centered on the screen.
See also focusedObject.
focusedObject : Item |
Set this property to any QML item (like an EntityBase), to make the camera automatically follow it. If this property is not set or set to null, the camera can be moved around freely.
freeOffset : point |
This property holds the offset for the free moving camera. For example, if you set this to (100, 100), an entity that is placed at the position (0, 0) is shown at (100, 100) on the screen. You can use this e.g. to display a sidebar.
freePosition : point |
Holds and controls the position of the camera, if it is free moving - so, if focusedObject is not set.
freeVelocityDecelerationFactor : real |
This is the deceleration factor for the free movement velocity. Set this to a value between 0.0 (stop velocity immediately) and 1.0 (no deceleration). By default, this is 0.9.
freeVelocityThreshold : real |
If the free movement velocity is smaller than this value, it will be set to zero. By default, this is 0.4.
gameWindowSize : point |
This property holds the gameWindowSize. Set this to Qt.point(scene.gameWindowAnchorItem.width, scene.gameWindowAnchorItem.height).
limitBottom : var |
Set this property to a number, to set a bottom limit for the camera. If you set limitTop and limitBottom, make sure the difference between these two is at least the height of your gameWindowSize.
See also limitTop.
limitLeft : var |
Set this property to a number, to set a left limit for the camera. If you set limitLeft and limitRight, make sure the difference between these two is at least the width of your gameWindowSize.
See also limitRight.
limitRight : var |
Set this property to a number, to set a right limit for the camera. If you set limitLeft and limitRight, make sure the difference between these two is at least the width of your gameWindowSize.
See also limitLeft.
limitTop : var |
Set this property to a number, to set a top limit for the camera. If you set limitTop and limitBottom, make sure the difference between these two is at least the height of your gameWindowSize.
See also limitBottom.
maxZoom : real |
minZoom : real |
mouseArea : alias |
This is an alias to the internal MouseArea. You can connect to its signals with e.g. mouseArea.onClicked: {...}
.
mouseAreaEnabled : bool |
With this property you can enable or disable the camera's default MouseArea, which allows you to move and zoom the free camera.
pinchArea : alias |
This is an alias to the internal PinchArea.
applyVelocity() |
With this function you can apply the current movement velocity to the camera when you use your own MouseArea instead of the built-in one from the Camera component.
For example after dragging and releasing the free moving camera, you may want the camera to keep scrolling a little further. Use this function to accomplish this.
To disable the built-in MouseArea and PinchArea, set mouseAreaEnabled to false.
applyZoom(zoomFactor, target) |
With this function you can apply a zoomFactor to the camera to zoom in or out. The target is an optional parameter. It is a point and allows you to zoom in towards, or out from a specific target point.
See also maxZoom, minZoom, and applyZoomThreshold.
centerFreeCameraOn(x, y) |
This function allows you to center the free moving camera on a specified position
See also freePosition and moveFreeCamera().
moveFreeCamera(deltaX, deltaY) |
Use this function to move the free camera. deltaX specifies the change of x, deltaY the change of y.
See also freePosition and centerFreeCameraOn().