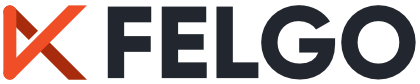
The Facebook item provides access to the native Facebook SDK on iOS and Android. More...
Import Statement: | import Felgo 4.0 |
Inherits: |
You can use the Facebook item to retrieve information about a logged in user and to send graph requests.
For more information also have a look at Facebook Plugin.
accessToken : string |
A read-only property returning the currently logged-in user's Facebook access token. Please note that there is no onAccessTokenChanged
signal. Instead, you can read the updated accessToken after a call to
openSession().
The accessToken can be used to send Facebook requests from outside your app, for example your server backend. It is valid for about 60 days and expires afterwards. After a call to openSession() you can store the accessToken and later on send other web requests with the same token for about 60 days. At every further openSession() call at application startup, the accessToken will stay the same until it expires. Thus in applications it is preferable to call openSession() when the application starts for example in Component.onCompleted()
and afterwards work with the accessToken.
Note: If you call closeSession() before openSession(), a new token is created.
See Facebook SSO for more informations on how long a user is active and how the user credentials are stored.
See also openSession() and closeSession().
advertiserIdCollectionEnabled : bool |
This property controls whether the Facebook SDK collects advertiser IDs.
Set it to true to true
to collect advertiser ID. Set it to false
to never collect the ID.
You can read more about app events and advertiser IDs at the official Facebook documentation.
The default value of this property is false
.
See also autoLogAppEventsEnabled.
appId : string |
Your Facebook application's app id. Be sure to set this property before calling any Facebook request functions, any changes afterwards will be ignored and will result in a ResultState of NoSession
.
If you want separate Facebook apps for iOS and Android, you can use the following example code:
Facebook { // A different application key is used for Android and iOS appId: Qt.platform.os === "ios" ? "<ios-app-id>" : "<android-app-id>" }
autoLogAppEventsEnabled : bool |
This property controls whether the Facebook SDK sends app events.
Set it to true to true
to send events. Set it to false
to send no automatic events.
You can read more about app events and advertiser IDs at the official Facebook documentation.
The default value of this property is false
.
See also advertiserIdCollectionEnabled.
The read-only grantedPermissions property contains a list of the current Facebook permissions granted by the user.
Regardless of your defined readPermissions and publishPermissions a user can simply deny a certain permission for your app. This property allows you to check the granted permissons if some requests may fail.
A full list of available permissions can be found at the Facebook Login Permissions.
See also readPermissions and publishPermissions.
profile : FacebookProfile |
A read-only property returning information about the currently logged in user. More details are listed at FacebookProfile.
The publishPermissions property contains a list of permissions needed for POST
graph requests.
A full list of available permissions can be found at the Facebook Login Permissions.
Note: Except for certain approved Facebook partners, using the Graph API to post Facebook messages is no longer supported. It is required to use the native share dialogs provided by the Facebook SDK instead.
The example below requests basic publish actions permissions. The publish_actions
permission allows your app to post on the news feed or post scores for games.
Facebook { publishPermissions: [ "publish_actions" ] }
See also readPermissions and grantedPermissions.
The readPermissions property contains a list of permissions needed for GET
graph requests.
A full list of available permissions can be found at the Facebook Login Permissions.
If no readPermissions are specified, the default permissions include access to the user name, first and last name, the gender, locale and the Facebook user id.
This example requests access to the email address and the user's friends in addition to the default public profile:
Facebook { readPermissions: [ "public_profile", "email", "user_friends" ] }
Note: If your app asks for more than than public_profile
, email
and user_friends
it will require review by Facebook before the app can request permission for these features
when used by people other than you as the app developer.
See also publishPermissions and grantedPermissions.
sessionState : enumeration |
Read only property holding the state of the current Facebook session. You have to open a session with openSession() before sending any requests to the Facebook API.
The possible values for sessionState
are:
Facebook.NoSession
(for newly initialized sessions without any interaction so far)Facebook.SessionOpening
(for sessions currently being opened after a call to openSession())
Facebook.SessionOpened
(for successfully opened sessions after calling openSession())
Facebook.SessionClosed
(for closed sessions after calling closeSession())
Facebook.SessionFailed
(for failed sessions during authentication with openSession())
Facebook.SessionPermissionDenied
(for sessions where the user denied a certain permission request)Please also have a look at Facebook SSO for more informations how long a user is active and how the user credentials are stored.
getGraphRequestFinished(string graphPath, enumeration resultState, string result) |
This handler is called after the getGraphRequest() request has finished. graphPath contains the initial sent API path.
The possible values for resultState
are:
Facebook.ResultOk
(request successfully performed)Facebook.ResultPermissionDenied
(the user did not allow the requested permissions for a getGraphRequest() request)
Facebook.ResultError
(the request ended with another error)Facebook.ResultInvalidSession
(the plugin needs an open session before it can call the API, make sure to call openSession() before)
If resultState is Facebook.Ok
the result parameter contains the requested json response object in string representation.
Note: The corresponding handler is onGetGraphRequestFinished
.
openSessionFailed(string reason) |
This handler provides additional information from the Facebook SDK if a call to openSession() result in a Facebook.SessionFailed
or
Facebook.SessionPermissionDenied resultState.
Note: The corresponding handler is onOpenSessionFailed
.
postGraphRequestFinished(string graphPath, enumeration resultState, string result) |
This handler is called after the postGraphRequest() request has finished. graphPath contains the initial sent API path.
The possible values for resultState
are:
Facebook.ResultOk
(request successfully performed)Facebook.ResultPermissionDenied
(the user did not allow the requested permissions for a postGraphRequest() request)
Facebook.ResultError
(the request ended with another error)Facebook.ResultInvalidSession
(the plugin needs an open session before it can call the API, make sure to call openSession() before)
If resultState is Facebook.Ok
the result parameter contains the requested json response object in string representation.
Note: The corresponding handler is onPostGraphRequestFinished
.
This handler is emitted after a successful call to openSession() and provides the currently granted (grantedPermissions) and denied (deniedPermissions) permissions for the retrieved Facebook session.
Note: The corresponding handler is onSessionOpened
.
void closeSession() |
Closes an active session with Facebook. You don't have to call this function explicitly as the session gets closed when exiting the app automatically.
See also sessionState.
void fetchUserDetails() |
Call this method to fetch additional user details into the profile property. Here is an example how to get the currently logged in user details after opening a session:
Facebook { appId: "YOUR_APP_ID" Component.onComplete: { openSession(); } onSessionStateChanged: { if (sessionState === Facebook.SessionOpened) { fetchUserDetails() } } onProfileChanged: { console.debug("Currently logged in user last name:", profile.lastName) } }
Sends a read request to the Graph API at graphPath path with a params map (optional) by using the http GET
method.
The full documenation about the OpenGraph API can be found at http://developers.facebook.com/docs/reference/api/.
The following example queries the Facebook friends of the currently logged in user:
facebook.getGraphRequest("me/friends");
Note: For receiving the friends list, the readPermissions property must contain the user_friends
permission.
See also getGraphRequestFinished.
void openSession() |
Call this method to authorize your game and open an active session. Be sure to call openSession()
in your app before sending any request to the Facebook API.
The following example opens a session at app startup and prints information about session state changes:
Facebook { id: facebook appId: "YOUR_APP_ID" onSessionStateChanged: { if (sessionState === Facebook.SessionOpened) { console.debug("Session opened."); } else if (sessionState === Facebook.SessionOpening) { console.debug("Session opening..."); } else if (sessionState === Facebook.SessionClosed) { console.debug("Session closed."); } else if (sessionState === Facebook.SessionFailed) { console.debug("Session failed."); } else if (sessionState === Facebook.SessionPermissionDenied) { console.debug("User denied requested permissions."); } } Component.onComplete: { facebook.openSession(); } }
See Facebook SSO for more informations how long a user is active and how the user credentials are stored automatically.
See also sessionState.
Sends a publish request to the Graph API at graphPath path with a params map (optional) by using the http POST
method.
The full documenation about the OpenGraph API can be found at http://developers.facebook.com/docs/reference/api/.
Note: Except for certain approved Facebook partners, using the Graph API to post Facebook messages is no longer supported. It is required to use the native share dialogs provided by the Facebook SDK instead.
See also postGraphRequestFinished.
void printAndroidKeyHash() |
Call this method to print out the currently used signing key hash to the log output during development.
Using the Facebook login in your android app requires you to provide the android signing key hash at your Facebook Developers app page. This method makes reading that hash easy. You need to provide the key hash for each signing certificate key store you are using (usually you use different signing certificates for development and release of your app).
Note: Make sure to remove the call to this method again before releasing your app, as this might be sensitive information.
Alternatively, you can also get the key hash from command line (requires the JDK in your path):
Windows:
keytool -exportcert -alias androiddebugkey -keystore "C:\\Users\\.android\\debug.keystore" | "C:\\OpenSSL\\bin\\openssl" sha1 -binary |"C:\\OpenSSL\bin\\openssl" base64
macOS / Linux:
keytool -exportcert -alias androiddebugkey -keystore ~/.android/debug.keystore | openssl sha1 -binary | openssl base64
When asked for, provide the password android
. You can then copy and paste the hash from the command line.